Understanding Variables in Bash Shell Scripting
In the second entry of the bash beginner series, learn about using variables in your bash shell scripts.

Time changes, and so do variables!
You must have played with variables quite a bit if you did any sort of programming.
If you never worked with variables before, you can think of them as a container that stores a piece of information that can vary over time.
Variables always come in handy while writing a bash script and in this tutorial, you will learn how to use variables in your bash scripts.
Using variables in bash shell scripts
In the last tutorial in this series, you learned to write a hello world program in bash.
#! /bin/bash
echo 'Hello, World!'
That was a simple Hello World script. Let's make it a better Hello World.
Let's improve this script by using shell variables so that it greets users with their names. Edit your hello.sh script and use read command to get input from the user:
#! /bin/bash
echo "What's your name, stranger?"
read name
echo "Hello, $name"
Now if you run your hello.sh script; it will prompt you for your name and then it will greet you with whatever name you provide to it:
abhishek@handbook:~/scripts$ ./hello.sh
What's your name, stranger?
Elliot
Hello, Elliot
In the above example, I entered Elliot as my name and then the script greeted me with “Hello, Elliot”. That’s definitely much better than a generic “Hello, World” program. Don't you agree?
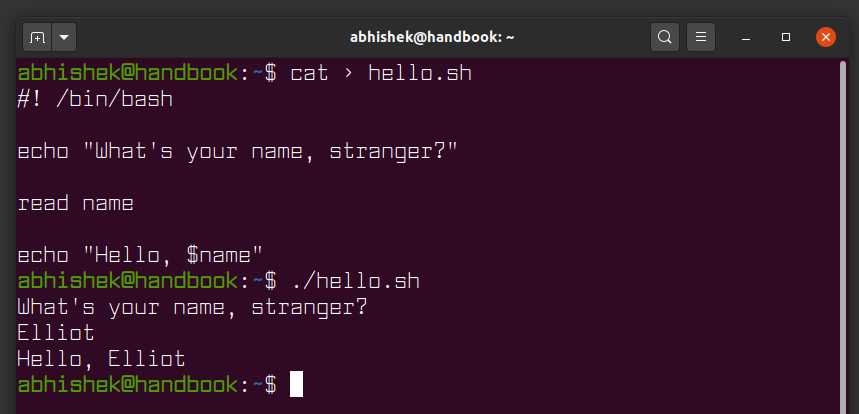
Step by step explanation of the above shell script
Now let’s go over the script line by line here to make sure that you understand everything.
I first included the shebang line to explicitly state that we are going to use bash shell to run this script.
#!/bin/bash
Next, I ask the user to enter his/her name:
echo "What's your name, stranger?"
That’s just a simple echo command to print a line to the terminal; pretty self-explanatory.
Now here’s the line where all the magic happens:
read name
Here, I used the read command to transfer the control from running script to the user, so that the user can enter a name and then store whatever user entered, in the 'name' variable.
Finally, the script greets the user with their name:
echo “Hello, $name”
Notice here, you have to precede the variable name with a dollar sign to get the value stored in the variable name. If you omit the dollar sign, “Hello, name” would be displayed instead.
Integers, strings or characters? How to create different variable data types in bash shell?
Let’s mess around a little bit more with the variables.
You can use the equal sign to create and set the value of a variable. For example, the following line will create a variable named age and will set its value to 27.
age=27
After you have created the age variable, you can change its value as much as you want.
age=3
The above command changes the value of the variable age from 27 to 3. If only times can go back, I can hear you saying!
Variables can hold different types of data; variables can store integers, strings, and characters.
letter=’c’
color=’blue’
year=2020
Constant variables in bash shell
You can also create a constant variable, that is to say, a variable whose value will never change! This can be done by preceding your variable name with the readonly
command:
readonly PI=3.14159
The above command will create a constant variable PI and set its value of 3.14159. Now, you can’t’ change the value of constant variable, if you try, you will get an error:
bash: PI: readonly variable
As you can see, you can only read the value of a constant variable, but you can never change its value after it is created.
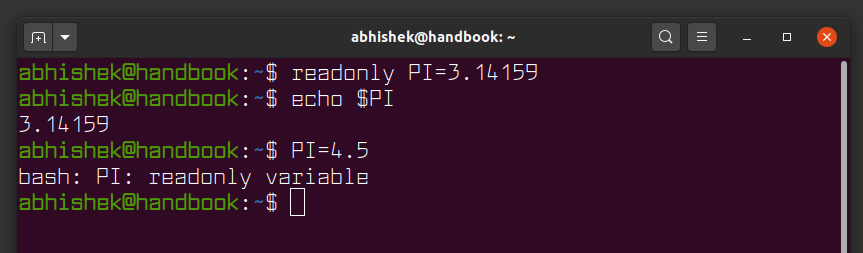
Command substitutions
The ability to store the output of a command into a variable is called command substitution and it’s by far one of the most amazing features of bash.
The date command is a classic example to demonstrate command substitution:
TODAY=$(date)
The above command will store the output of the command date into the variable TODAY. Notice, how you need to enclose the date command within a pair of parentheses and a dollar sign (on the left).
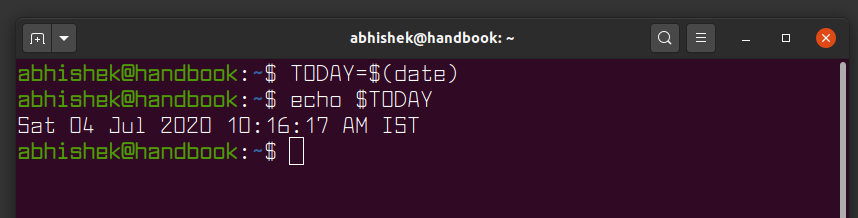
Alternatively, you can also enclose the command within a pair of back quotes:
TODAY=`date`
The back quote method is the old way of doing command substitution, and so I highly recommend that you avoid it and stick with the modern approach:
variable=$(command)
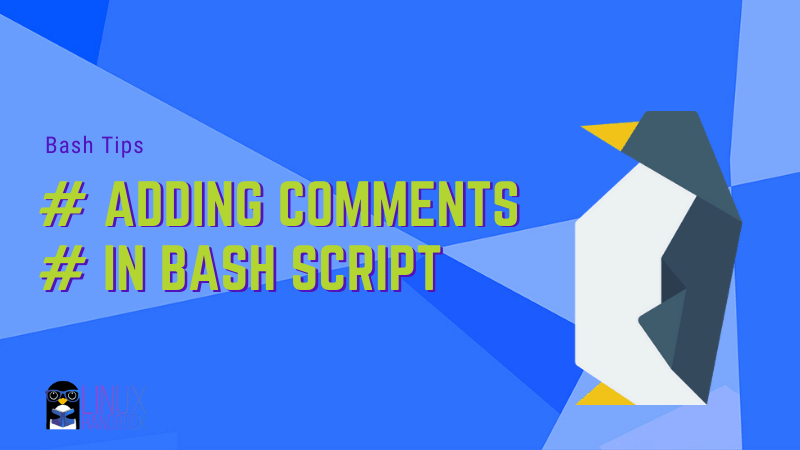
Before you go, try turning Hello World script to a smart HelloWorld script
Now since you just learned how to do command substitution, it would make sense to visit the Hello World script one last time to perfect it!
Last time, you asked the user to enter his/her name so the script greets them; this time, you are not going to ask, your script already knows it!
Use the whoami command along with command substitution to greet whoever run the script:
#! /bin/bash
echo "Hello, $(whoami)"
As you can see, you only needed just two lines! Now run the script:
./hello.sh
It works like a charm!
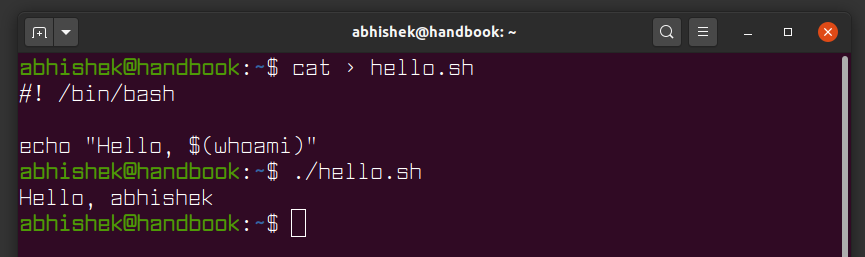
If interested, you should also read about using declare for better variable management in bash.
Alright, this brings us to the end of this tutorial. You may practice what you just learned by solving the problems and refer to their solutions if you get stuck or need a hint.
I hope you have enjoyed working with shell variables as much as me. Check out the next chapter in this series, where I discuss how you can pass arguments to your shell scripts.
A Linux sysadmin who likes to code for fun. I have authored Learn Linux Quickly book to help people learn Linux easily. I also like watching the NBA and going for a cruise with my skateboard.