Writing Comments in Bash Scripts: Single Line, Inline and Multi-line Comments
Wondering about comments in bash script? Here's how to add single, inline or multiline comments in Bash scripts.

Comments are integral part of any programming or scripting language. Bash is no different.
Like any programming language, comments in bash script are used to make the code more understandable. You can also use comments to skip part of codes while debugging your script.
In this bash tip, I’ll show you three ways to add comments in your shell script:
- Single line comment
- Inline comment
- Multiline comment
Single line comments in bash script
Any line starting with the hash/pound key # is treated as comment in bash. The only exception to this rule is #! which is shebang and is used to indicate which shell to be used to process the script.
Let’s see it with a sample bash script:
#!/bin/bash
#Define variables here (single line comment)
message="Hello"
day=$(date +%A)
#Print some messages ... another comment
echo "$message $USER! It's $day today. Enjoy your day!"
echo "Goodbye for now!"
As you can see, there are two single line comments in the example above.
Inline comments in bash script
You can also add inline comments in bash scripts. Instead of starting the line with #, add the comment starting with # at the end of code line.
Here’s an example:
#!/bin/bash
message="Hello"
day=$(date +%A) #This will print only the day (example of inline comment)
echo "$message $USER! It's $day today. Enjoy your day!"
echo "Goodbye for now!"
I added an inline comment in the line where variable day has been declared. This tells you that the date command with +%A will only show the present day.
I removed other comments to avoid confusion.
Multiline comments in bash script
Multiline or block comment in bash is not directly possible. You can use multiple single line comments but I know that’s not very convenient, specially when you have to uncomment the entire block of code.
#!/bin/bash
# Add a # in front of every line you want to comment out
# But it's not very convenient way
# message="Hello"
# day=$(date +%A)
# echo "$message $USER! It's $day today. Enjoy your day!"
echo "Goodbye for now!"
Thankfully, there is a workaround to comment multiple lines in bash using here document. It’s a redirection that provides multiple lines of input to a command. When it is not redirected to any command, it can be used to add block comments.
#!/bin/bash
<<Block_comment
message="Hello"
day=$(date +%A) #This will print only the day, not entire date and time
echo "$message $USER! It's $day today. Enjoy your day!"
Block_comment
echo "Goodbye for now!"
If you run the above script, it will only print .Goodbye for now!’. All the code between <<Block_comment and Block_comment is ignored.
If you are debugging your code and want to comment out blocks of code, you may use it. Otherwise, avoid using it in your main scripts because it’s not a shell built-in feature.
Commenting in YAML is a similar experience. Multiline comments are not available there as well.
Conclusion
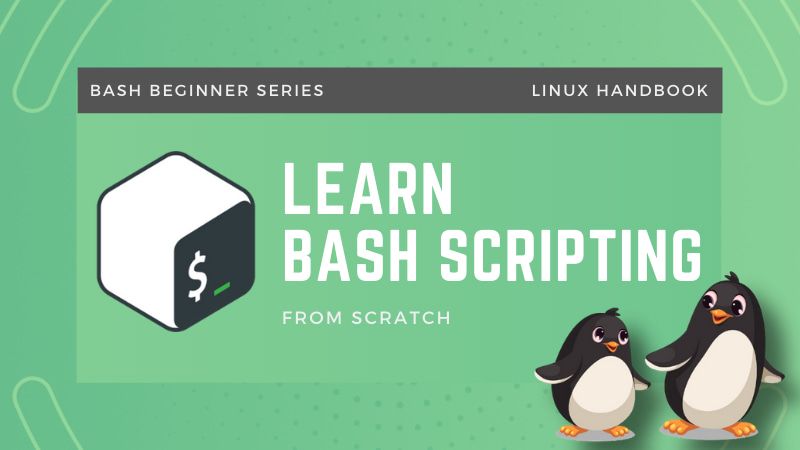
I hope you like this quick bash tip on writing comments in your shell script. Your questions and suggestions are always welcome.
Creator of Linux Handbook and It's FOSS. An ardent Linux user who has new-found love for self-hosting, homelabs and local AI.