Create and Run Your First Bash Shell Script
Take the first step towards shell scripting. Learn what it takes to create a simple bash script and how to run it.

If you have to do it more than once, automate it!
You will often find yourself repeating a single task on Linux over and over again. It may be a simple backup of a directory or it could be cleaning up temporary files or it can even be cloning of a database.
Automating a task is one of the many useful scenarios where you can leverage the power of bash scripting.
Let me show you how to create a simple bash shell script, how to run a bash script and what are the things you must know about shell scripting.
Create and run your first shell script
Let’s first create a new directory named scripts that will host all our bash scripts.
mkdir scripts
cd scripts
Now inside this 'scripts directory', create a new file named hello.sh using the cat command:
cat > hello.sh
Insert the following line in it by typing it in the terminal:
echo 'Hello, World!'
Press Ctrl+D to save the text to the file and come out of the cat command.
You can also use a terminal-based text editor like Vim, Emacs or Nano. If you are using a desktop Linux, you may also use a graphical text editor like Gedit to add the text to this file.
So, basically you are using the echo command to print "Hello World". You can use this command in the terminal directly but in this test, you'll run this command through a shell script.
Now make the file hello.sh executable by using the chmod command as follows:
chmod u+x hello.sh
And finally, run your first shell script by preceding the hello.sh with your desired shell “bash”:
bash hello.sh
You'll see Hello, World!
printed on the screen. That was probably the easiest Hello World program you have ever written, right?
Here's a screenshot of all the steps you saw above:
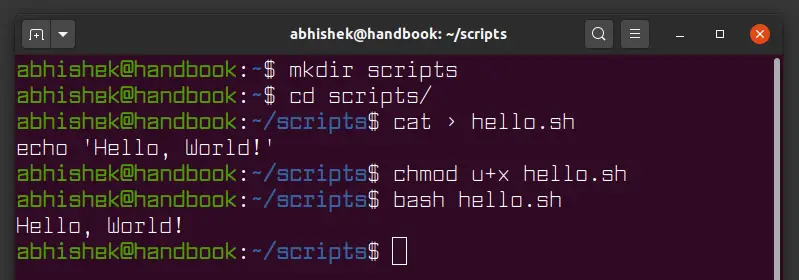
Convert your shell script into bash script
Confused? Don't be confused just yet. I'll explain things to you.
Bash which is short for “Bourne-Again shell” is just one type of many available shells in Linux.
A shell is a command line interpreter that accepts and runs commands. If you have ever run any Linux command before, then you have used the shell. When you open a terminal in Linux, you are already running the default shell of your system.
Bash is often the default shell in most Linux distributions. This is why bash is often synonymous to shell.
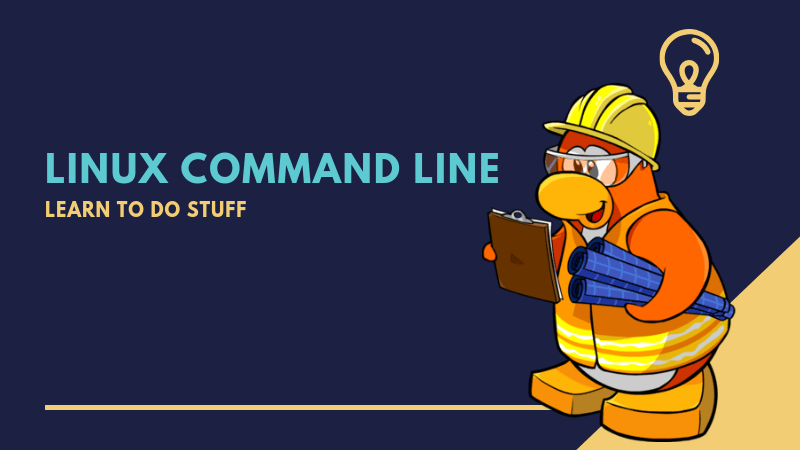
The shell scripts often have almost the same syntaxes, but they also differ sometimes. For example, array index starts at 1 in Zsh instead of 0 in bash. A script written for Zsh shell won't work the same in bash if it has arrays.
To avoid unpleasant surprises, you should tell the interpreter that your shell script is written for bash shell. How do you do that? You use shebang!
The SheBang line at the beginning of shell script
The line “#!/bin/bash” is referred to as the shebang line and in some literature, it’s referred to as the hashbang line and that’s because it starts with the two characters hash ‘#’ and bang ‘!’.
#! /bin/bash
echo 'Hello, World!'
When you include the line “#!/bin/bash” at the very top of your script, the system knows that you want to use bash as an interpreter for your script. Thus, you can run the hello.sh script directly now without preceding it with bash.
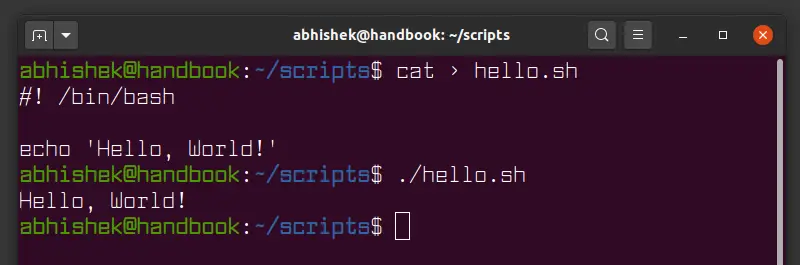
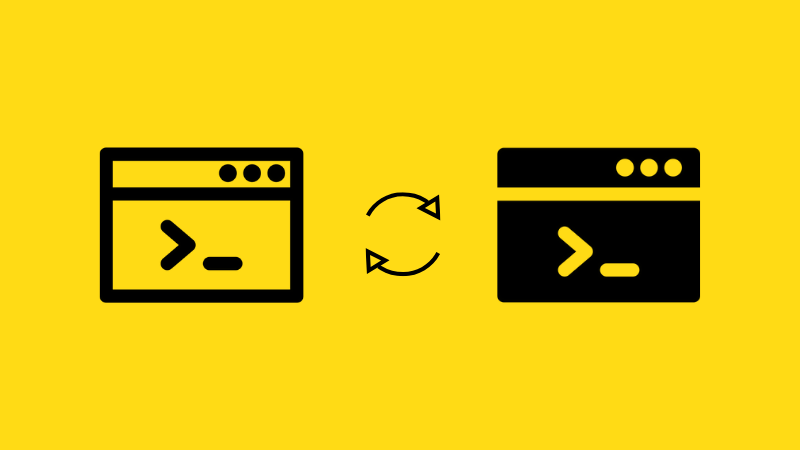
Adding your shell script to the PATH (so that it can be run from any directory)
You may have noticed that I used ./hello.sh to run the script; you will get an error if you omit the leading ./
abhishek@handbook:~/scripts$ hello.sh
hello.sh: command not found
Bash thought that you were trying to run a command named hello.sh. When you run any command on your terminal; they shell looks for that command in a set of directories that are stored in the PATH variable.
You can use echo to view the contents of that PATH variable:
echo $PATH
/home/user/.local/bin:/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin:/usr/games:/usr/local/games:/snap/bin
The colon character (:) separates the path of each of the directories that your shell scans whenever you run a command.
Linux commands like echo, cat etc can be run from anywhere because their executable files are stored in the bin directories. The bin directories are included in the PATH. When you run a command, your system checks the PATH for all the possible places it should look for to find the executable for that command.
If you want to run your bash script from anywhere, as if it were a regular Linux command, add the location of your shell script to the PATH variable.
First, get the location of your script's directory (assuming you are in the same directory), use the pwd command:
pwd
Use the export command to add your scripts directory to the PATH variable.
export PATH=$PATH:/home/user/scripts
Notice that I have appended the 'scripts directory' to the very end to our PATH variable. So that the custom path is searched after the standard directories.
The moment of truth is here; run hello.sh:
abhishek@handbook:~/scripts$ hello.sh
Hello, World!
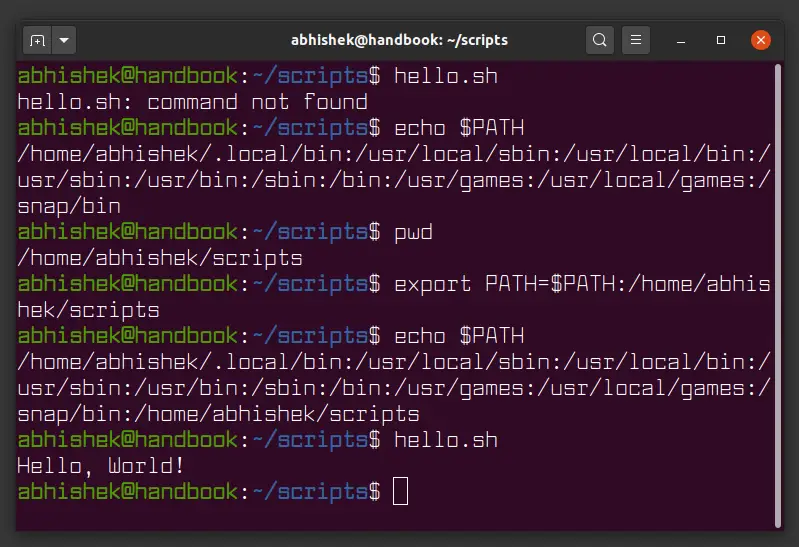
It works! This takes us to the end of this tutorial. I hope you now have some basic idea about shell scripting. You can download the PDF below and practice what you learned with some sample scripting challenges. Their solutions are also provided in case you need hints.
Since I introduced you to PATH variable, stay tuned for the next bash scripting tutorial where I discuss shell variables in detail.
A Linux sysadmin who likes to code for fun. I have authored Learn Linux Quickly book to help people learn Linux easily. I also like watching the NBA and going for a cruise with my skateboard.