Passing Arguments to Bash Scripts
In the third part of the Bash Beginner Series, you'll learn to pass arguments to a bash shell script. You'll also learn about special bash shell variables.

Arguments can be useful, especially with Bash!
So far, you have learned how to use variables to make your bash scripts dynamic and generic, so it is responsive to various data and different user input.
In this tutorial, you will learn how you can pass variables to a bash scripts from the command line.
Passing argument to a bash shell script
The following script count_lines.sh
will output the total number of lines that exist in whatever file the user enters:
#!/bin/bash
echo -n "Please enter a filename: "
read filename
nlines=$(wc -l < $filename)
echo "There are $nlines lines in $filename"
For example, the user can enter the file /etc/passwd
and the script will spit out the number of lines as a result:

This script works fine; however, there is a much better alternative!
Instead of prompting the user for the filename, we can make the user simply pass the filename as a command line argument while running the script as follows:
./count_lines.sh /etc/passwd
The first bash argument (also known as a positional parameter) can be accessed within your bash script using the $1
variable.
So in the count_lines.sh script, you can replace the filename variable with $1
as follows:
#!/bin/bash
nlines=$(wc -l < $1)
echo "There are $nlines lines in $1"
Notice that I also got rid of the read and first echo command as they are no longer needed!
Finally, you can run the script and pass any file as an argument:
./count_lines.sh /etc/group
There are 73 lines in /etc/group
Passing multiple arguments to a bash shell script
You can pass more than one argument to your bash script. In general, here is the syntax of passing multiple arguments to any bash script:
script.sh arg1 arg2 arg3 β¦
The second argument will be referenced by the $2
variable, the third argument is referenced by $3
, .. etc.
The $0 variable contains the name of your bash script in case you were wondering!
Now we can edit our count_lines.sh
bash script so that it can count the lines of more than one file:
#!/bin/bash
n1=$(wc -l < $1)
n2=$(wc -l < $2)
n3=$(wc -l < $3)
echo "There are $n1 lines in $1"
echo "There are $n2 lines in $2"
echo "There are $n3 lines in $3"
You can now run the script and pass three files as arguments to the bash script:

As you can see, the script outputs the number of lines of each of the three files; and needless to say that the ordering of the arguments matters, of course.
Getting creative with arguments in Bash shell
There are a whole lot of Linux commands out there.
Some of them are a bit complicated as they may have long syntax or a long array of options that you can use.
Fortunately, you can use bash arguments to turn a hard command into a pretty easy task!
To demonstrate, take a look at the following find.sh
bash script:
#!/bin/bash
find / -iname $1 2> /dev/null
Itβs a very simple script that yet can prove very useful! You can supply any filename as an argument to the script and it will display the location of your file:

You see how this is now much easier than typing the whole find command! This is a proof that you can use arguments to turn any long complicated command in Linux to a simple bash script.
If you are wondering about the 2> /dev/null
, it means that any error message (like file cannot be accessed) won't be displayed on the screen. I suggest reading about stderr redirection in Linux and /dev/null to get more knowledge on this topic.
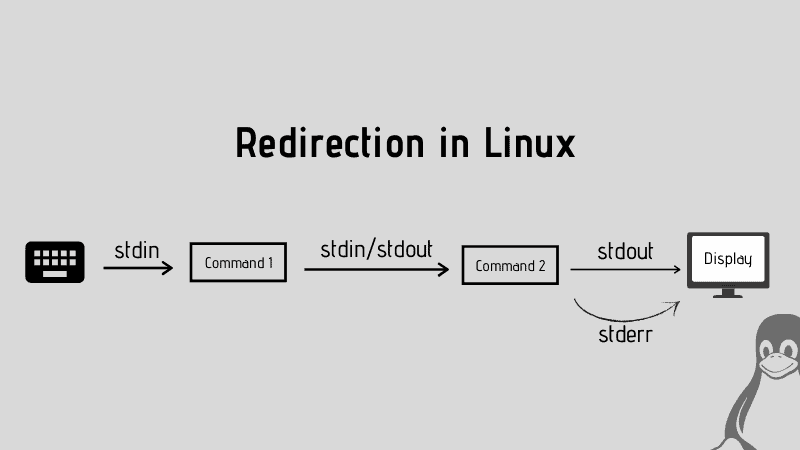
Bonus Tip: Special variables in Bash shell
Bash has a lot of built-in special variables that are quite handy and are available at your disposal.
The table below highlights the most common special built-in bash variables:
Special Variable | Description |
---|---|
$0 | The name of the bash script. |
$1, $2...$n | The bash script arguments. |
$$ | The process id of the current shell. |
$# | The total number of arguments passed to the script. |
$@ | The value of all the arguments passed to the script. |
$? | The exit status of the last executed command. |
$! | The process id of the last executed command. |
To see these special variables in action; take a look at the following variables.sh
bash script:
#!/bin/bash
echo "Name of the script: $0"
echo "Total number of arguments: $#"
echo "Values of all the arguments: $@"
You can now pass any arguments you want and run the script:

Alright, this brings us to the end of this chapter. I hope you now realize how powerful and useful bash arguments can be.
Need some practice, download this PDF and practice passing arguments to bash scripts with simple scripting challenges. Their solutions are also included.
After that, take a look at the next chapter where I am going to show you can create and utilize arrays in your bash scripts.
A Linux sysadmin who likes to code for fun. I have authored Learn Linux Quickly book to help people learn Linux easily. I also like watching the NBA and going for a cruise with my skateboard.