String Operations in Bash
Let's pull some strings and learn to handle strings in bash scripts.

Let’s manipulate some strings!
If you are familiar with variables in bash, you already know that there are no separate data types for string, int etc. Everything is a variable.
But this doesn't mean that you don't have string manipulation functions.
In the previous chapter, you learned arithmetic operators in Bash. In this chapter, you will learn how to manipulate strings using a variety of string operations. You will learn how to get the length of a string, concatenate strings, extract substrings, replace substrings, and much more!
Get string length
Let's start with getting the length of a string in bash.
A string is nothing but a sequence (array) of characters. Let’s create a string named distro and initialize its value to “Ubuntu”.
distro="Ubuntu"
Now to get the length of the distro string, you just have to add #
before the variable name. You can use the following echo statement:
kabary@handbook:~/scripts$ echo ${#distro}
6
Do note that echo command is for printing the value. {#string}
is what gives the length of string.
Concatenating two strings
You can append a string to the end of another string; this process is called string concatenation.
To demonstrate, let’s first create two strings str1 andstr2 as follows:
str1="hand"
str2="book"
Now you can join both strings and assign the result to a new string named str3 as follows:
str3=$str1$str2
It cannot be simpler than this, can it?
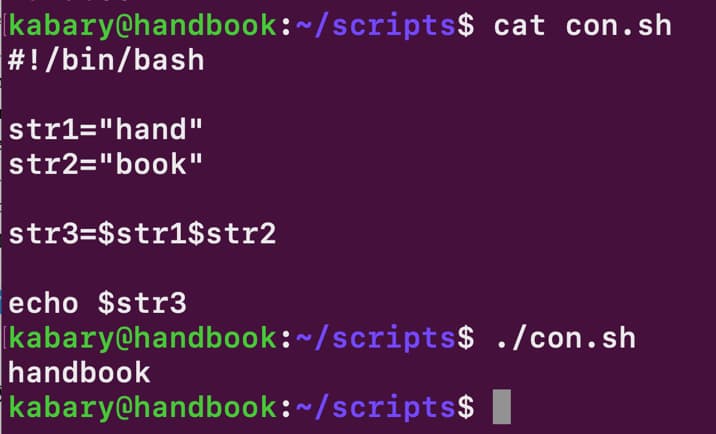
Finding substrings
You can find the position (index) of a specific letter or word in a string. To demonstrate, let’s first create a string named str as follows:
str="Bash is Cool"
Now you can get the specific position (index) of the substring cool. To accomplish that, use the expr
command:
kabary@handbook:~/scripts$ word="Cool"
kabary@handbook:~/scripts$ expr index "$str" "$word"
9
The result 9 is the index where the word “Cool” starts in the str string.
I am deliberately avoiding using conditional statements such as if, else because in this bash beginner series, conditional statements will be covered later.
Extracting substrings
You can also extract substrings from a string; that is to say, you can extract a letter, a word, or a few words from a string.
To demonstrate, let’s first create a string named foss as follows:
foss="Fedora is a free operating system"
Now let’s say you want to extract the first word “Fedora” in the foss string. You need to specify the starting position (index) of the desired substring and the number of characters you need to extract.
Therefore, to extract the substring “Fedora”, you will use 0 as the starting position and you will extract 6 characters from the starting position:
kabary@handbook:~/scripts$ echo ${foss:0:6}
Fedora
Notice that the first position in a string is zero just like the case with arrays in bash. You may also only specify the starting position of a substring and omit the number of characters. In this case, everything from the starting position to the end of the string will be extracted.
For example, to extract the substring “free operating system” from the foss string; we only need to specify the starting position 12:
kabary@handbook:~/scripts$ echo ${foss:12}
free operating system
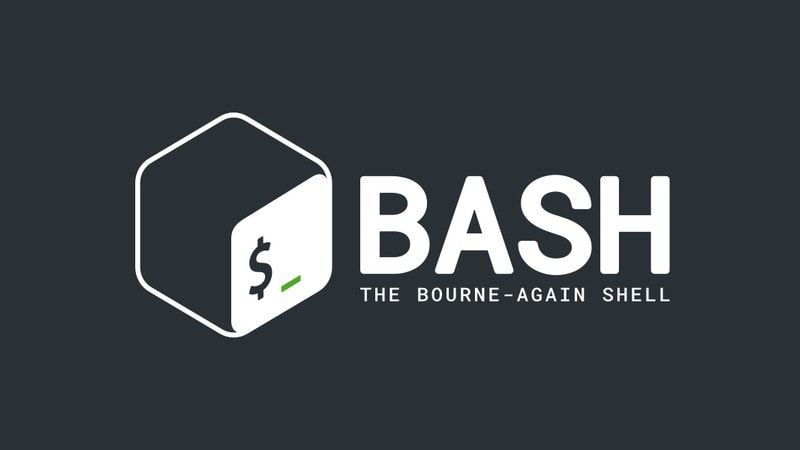
Replacing substrings
You can also replace a substring with another substring; for example, you can replace “Fedora” with “Ubuntu” in the foss string as follows:
kabary@handbook:~/scripts$ echo ${foss/Fedora/Ubuntu}
Ubuntu is a free operating system
Let’s do another example, let’s replace the substring “free” with “popular”:
kabary@handbook:~/scripts$ echo ${foss/free/popular}
Fedora is a popular operating system
Since you are just printing the value with echo command, the original string is not reallt altered.
Deleting substrings
You can also remove substrings. To demonstrate, let’s first create a string named fact as follows:
fact="Sun is a big star"
You can now remove the substring “big” from the string fact:
kabary@handbook:~/scripts$ echo ${fact/big}
Sun is a star
Let’s create another string named cell:
cell="112-358-1321"
Now let’s say you want to remove all the dashes from the cell string; the following statement will only remove the first dash occurrence in the cell string:
kabary@handbook:~/scripts$ echo ${cell/-}
112358-1321
To remove all dash occurrences from the cell string, you have to use double forward slashes as follows:
kabary@handbook:~/scripts$ echo ${cell//-}
1123581321
Notice that you are using echo statements and so the cell string is intact and not modified; you are just displaying the desired result!
To modify the string, you need to assign the result back to the string as follows:
kabary@handbook:~/scripts$ echo $cell
112-358-1321
kabary@handbook:~/scripts$ cell=${cell//-}
kabary@handbook:~/scripts$ echo $cell
1123581321
Converting upper and lower-case letters in string
You can also convert a string to lowercase letter or uppercase letters. Let’s first create two string named legend and actor:
legend="john nash"
actor="JULIA ROBERTS"
You can convert all the letters in the legend string to uppercase:
kabary@handbook:~/scripts$ echo ${legend^^}
JOHN NASH
You can also convert all the letters in the actor string to lowercase:
kabary@handbook:~/scripts$ echo ${actor,,}
julia roberts
You can also convert only the first character of the legend string to uppercase as follows:
kabary@handbook:~/scripts$ echo ${legend^}
John nash
Likewise, you can convert only the first character of the actor string to lowercase as follows:
kabary@handbook:~/scripts$ echo ${actor,}
jULIA ROBERTS
You can also change certain characters in a string to uppercase or lowercase; for example, you can change the letters j
and n
to uppercase in the legend string as follows:
kabary@handbook:~/scripts$ echo ${legend^^[jn]}
JohN Nash
Awesome! This brings us to the end of this tutorial in the bash beginner series. Download the below PDF and practice what you just learned.
I hope you have enjoyed doing string manipulation in bash and check the next chapter as you will learn how to add decision-making skills to your bash scripts!
A Linux sysadmin who likes to code for fun. I have authored Learn Linux Quickly book to help people learn Linux easily. I also like watching the NBA and going for a cruise with my skateboard.