Using Command Substitution in Bash Shell
Command substitution is an important feature that allows you to store the output of a command into a variable.
β Sagar Sharma

Command substitution in bash is nothing but passing the output of one command to another for various use cases.
Sounds complex? Let me share a quick example:
# Get the current date using the date command
current_date=$(date)
# Print the current date
echo "Today's date is: $current_date"
The above bash script simply uses the date
command and passes the output to the current_date
variable.
Later on, the variable current_date
was used in the echo statement to print the current date.
If you notice carefully, I used $(...)
around the date command which captured the output of the date command and passed it to the current_date
variable.
Yep, that's one way of command substitution in bash but there are other ways as well. Let's have a look.
How to substitute commands in bash
There are two ways you can substitute commands in bash:
- Using dollar parentheses $()
- Using backticks (` `)
So in this tutorial, I will share how you can use both methods for command substitution and will also share how you can do that in nested form.
Let's start with the first one.
Command substitution using dollar parentheses $()
To use the dollar parentheses for command substitution, all you have to do is follow the given command syntax:
Variable=$(command)
You can also add additional text to support the command output as shown here:
Variable="Optional text $(command)"
Let me give you a simple example. Here, I have used the whoami command to find the currently logged-in user with the echo command:
echo "The current user is: $(whoami)"

You can also assign the whole value to the variable and then use it anywhere in the bash script itself:
#!/bin/bash
current_user=$(whoami)
echo "The current user is: $current_user"
If you're curious, here's the expected output after executing the above script:

While the output is similar to the earlier example, in this script, what I did is substituted the value of the whoami
command and passed it to the current_user
variable.
Later on, used it in the echo statement.
Alternatively, you can use multiple command substitutions.
For example, here I used it two times for the echo statement:
echo "Hello, $(whoami)! You are running this script on $(date)."

Pretty cool. Right?
Command substitution using backticks (` ` )
This is another way of substituting commands in bash.
To use backticks for the command substitution, simply follow the given command syntax:
variable=`command`
If you want to use it with additional text, you can do that too using the following:
variable="Additional text `date`"
To demonstrate this, I used a simple bash script:
#!/bin/bash
result=`echo "5 + 7" | bc`
echo "The result of the calculation is: $result"
In the above script, I used the bc command and piped it to the echo command to calculate the addition of two numbers and stored it in the result
variable.
Later on, it was printed with the echo statement.
Here's what you can expect while executing the above script:

Using backticks for multiple substitutions
To use backticks for multiple substitutions, use the following syntax:
result=`command1 \`command2\` command3\`
Yep, you have to separate every command with the backslash (\).
If you want to add additional text, then it will look like this:
result= `command1 \ "Additional text `command2\` Some more text here `command3\`"`
In simple terms, you can add text anywhere in the syntax between ""
.
For example, here, I used backticks for multiple substitutions to get the current date and calculate addition:
result=`echo "Today is \`date\`, and the result of the calculation is \`echo 5 + 7 | bc\`."`
echo "$result"

Pretty easy. Right?
More on bash and substitution
Process substitution is also an excellent though lesser known bash feature.
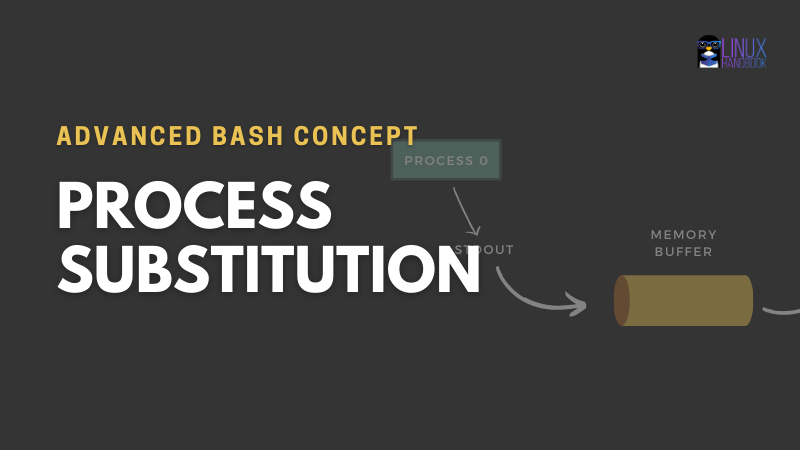
Want to automate operations in bash? Here's how you do it:
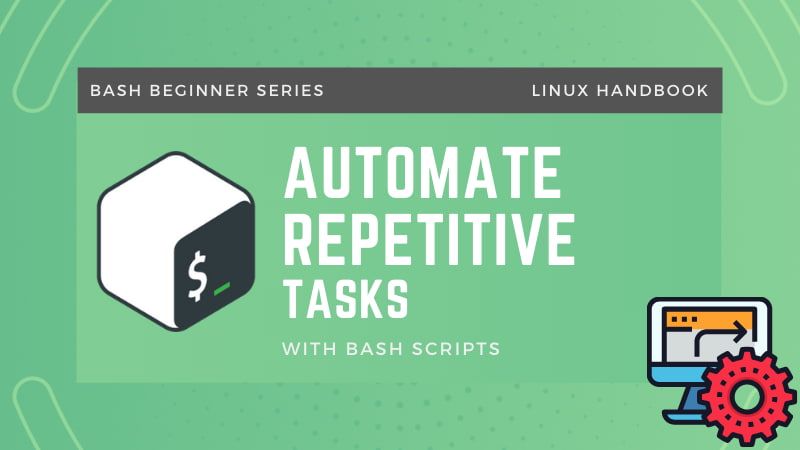
I hope you will find this guide helpful.
A software engineer who loves to tinker with hardware till it gets crashed. While reviving my crashed system, you can find me reading literature, manga, or watering my plants.