Difference Between Single and Double Quote in Bash Shell
Quotes are an integral part of shell scripting and Linux command but they could often be confusing for new users. This article will demystify quotes for you.
You'll often use quotes in Linux command line. Dealing with spaces in filename? You use quotes. Handling special characters? You use quotes again.
The quotes are 'special feature' in Linux shell and it may get confusing, specially if you are new to Linux commands and shell scripting.
I'll explain the different types of quote characters and their usage in shell scripting.
There are four different types of quote characters:
- Single quote '
- Double quote "
- Backslash \
- Back quote `
Except the backlash, the rest of the three characters occur in pair.
Let's have a look at them in detail.
1. Single quote
The single quote in Shell ignores all type of special characters in it. Everything between the single quotes is considered one single element.
Let's take it with example. Here's a sample text file with some Cricketers from the 90s.
abhishek@its-foss:~$ cat cricket
Allan Donald, South Africa
Steve Waugh, Australia
Mark Waugh, Australia
Henry Olonga, Zimbabwe
Sachin Tendulkar, India
Now, you use grep command to look for cricketers with family name Waugh. This will give you two results:
abhishek@its-foss:~$ grep Waugh cricket
Steve Waugh, Australia
Mark Waugh, Australia
But if you want to search for Steve Waugh only, and you try using it as it is, you'll see an error:
abhishek@its-foss:~$ grep Steve Waugh cricket
grep: Waugh: No such file or directory
cricket:Steve Waugh, Australia
Why? Because space is used for separating command, options and arguments. In the above example, it takes Steve
as the first argument to grep but Waugh
and cricket
are taken as the files in which it should preform the search. Since there is no file named Waugh
, it throws an error for that. At the same time, it also shows the result from the file cricket
.
This is where quote comes to the rescue. When you enclose the arguments in quotes, it is interpreted as a single entity.
abhishek@its-foss:~$ grep 'Steve Waugh' cricket
Steve Waugh, Australia
Basically, when the shell see the first single quote, it ignores any special characters (white space is also a special character) until it finds another single quote (closing quotes).
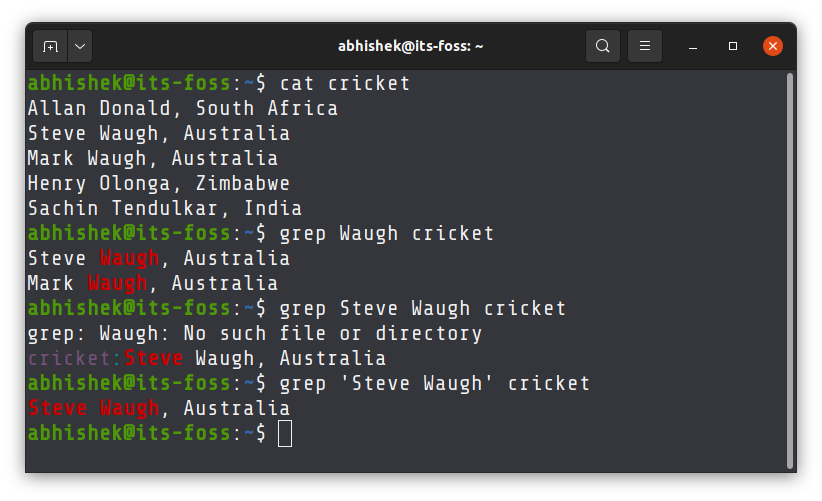
Ignoring all special characters
All special characters lose their meaning if they are enclosed in single quotes. Let's see it with examples.
Let's declare a variable in shell. If you echo the variable name with $, it shows the value of the variable.
abhishek@its-foss:~$ var=my_variable
abhishek@its-foss:~$ echo $var
my_variable
But if you wrap it in single quotes, $ will lose its special power.
abhishek@its-foss:~$ echo '$var'
$var
Now only that. The enter key is also preserved under single quotes.
abhishek@its-foss:~$ echo 'how are
> you?'
how are
you?
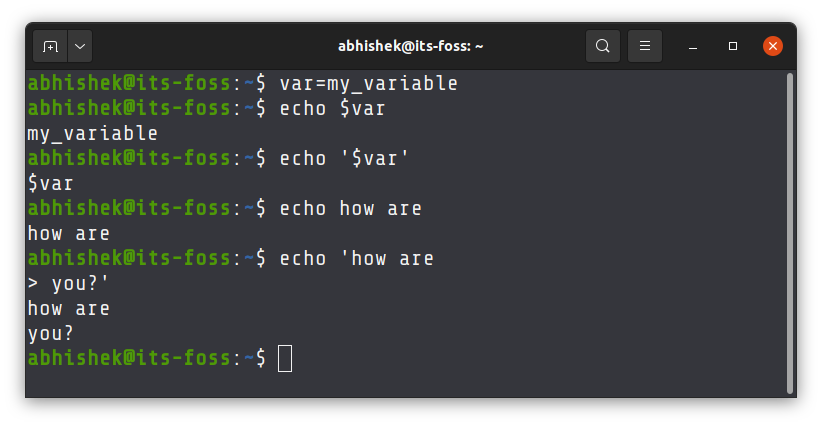
2. Double quote
Double quotes work almost similar to single quotes. Almost because they also tend to ignore all the special characters except:
- Dollar signs $
- Back quotes `
- Backslashes \
Since dollar sign is not ignored, you can expect variable name to be substituted with its value. Which is not the case with the single quotes.
abhishek@its-foss:~$ var=my_variable
abhishek@its-foss:~$ echo "$var"
my_variable
abhishek@its-foss:~$ echo '$var'
$var
The double quotes can be used to hide single quotes from the shell.
abhishek@its-foss:~$ var=My 'own villa' is yellow
own villa: command not found
abhishek@its-foss:~$ var="My 'own villa' is yellow"
abhishek@its-foss:~$ echo $var
My 'own villa' is yellow
Similarly, you can use the single quotes to hide double quotes from the shell.
abhishek@its-foss:~$ var=he said, "Awesome!"
said,: command not found
abhishek@its-foss:~$ var='he said, "Awesome!"'
abhishek@its-foss:~$ echo $var
he said, "Awesome!"
3. Backslash
Backslash is like putting single quotes around a single character. The backslash 'escapes' the character it is put before.
This means that the character followed by the backslash will lose its special meaning (if any).
abhishek@its-foss:~$ var=variable
abhishek@its-foss:~$ echo \var
var
abhishek@its-foss:~$ echo $var
variable
abhishek@its-foss:~$ echo \$var
$var
Because v
has no special meaning, echo \var
simply prints var. On the other hand, when it is used with $var
, the backslash escapes the special meaning of $
and thus it is printed as $var
instead of the value of var
.
Continue the line with backslash
You'll notice another practical use of the backslash character in continuing a single command over multiple lines.
When a command is too long or if it is a combination of commands, you'll see some websites using backslash to display the single command in more than one line. This makes the command/code more readable.
Take this long command for example.
docker run --name server --network net -v html:/usr/share/nginx/html -v $PWD/custom-config.conf:/etc/nginx/nginx.conf -p 80:80 --restart on-failure -d nginx:latest
The same command can be broken down into multiple line thanks to backslashes.
docker run --name server --network net \
-v html:/usr/share/nginx/html \
-v $PWD/custom-config.conf:/etc/nginx/nginx.conf \
-p 80:80 --restart on-failure -d nginx:latest
It's the same command, just a little easier to understand.
Backslash inside double quotes
Backslash is one of the three special characters that keeps its special meaning along with dollar sign and back quotes.
This way, you can use backslash to escape the special meaning of dollar sign, double quotes and back quotes in double quotes.
Take the below example where $5
is considered a variable which is not declared and hence it has no value. This is why it is ignored from the output of the echo command.
abhishek@its-foss:~$ echo "Meal costs $5.25"
Meal costs .25
To use this $ without being interpreted as the value of a variable, you have to escape it with /.
abhishek@its-foss:~$ echo "Meal costs \$5.25"
Meal costs $5.25
Here, the backslash (\) is interpreted for its superpower, which is to escape the subsequent character's special meaning.
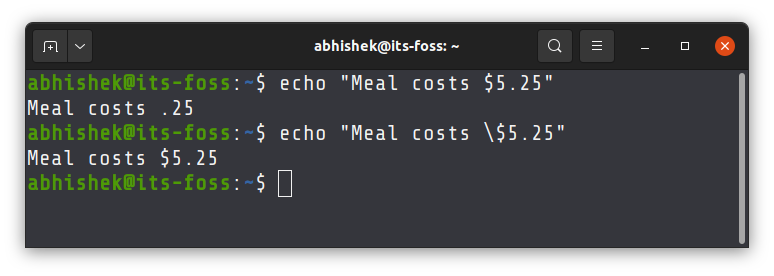
4. Back quotes
The last in this list is back quotes and it has a special meaning. It is used for command substitution.
The shell has this command substitution feature where a specified command is replaced with the output of the command.
In the example below, `date` is replaced by the output of the date command showing the current date and time of the Linux system.
abhishek@its-foss:~$ echo The current date and time is `date`
The current date and time is Monday 23 August 2021 04:55:18 PM IST
One thing to keep in mind is that the substitution will only take place if there is a command between the back quotes. Otherwise, it is displayed as it is.
abhishek@its-foss:~$ echo 'The current date and time is `late`'
The current date and time is `late`
Again, the double quotes interpret the back quotes but the single quotes ignore its special meaning.
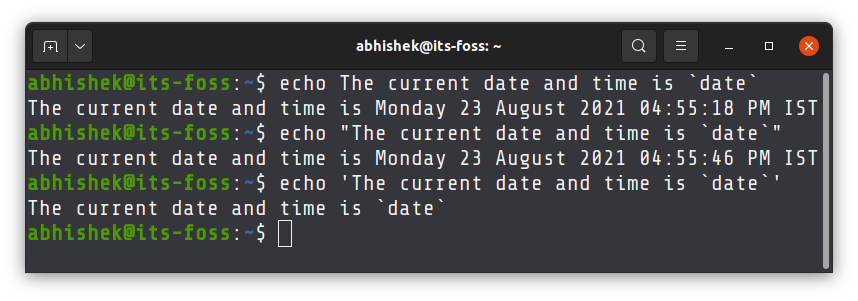
Back quotes are not recommended anymore
For years, the back quotes were used for command substitution in Shell scripting. But these days, modern UNIX and Linux systems prefer the $(command)
construct instead.
abhishek@its-foss:~$ echo The current date and time is $(date)
The current date and time is Monday 23 August 2021 05:55:47 PM IST
Stay with the time and stop using the back quotes now.
I hope you have a better understanding of quotes in Linux and shell scripting now.
Please leave your suggestions and questions in the comment section.
Creator of Linux Handbook and It's FOSS. An ardent Linux user & open source promoter. Huge fan of classic detective mysteries from Agatha Christie and Sherlock Holmes to Columbo & Ellery Queen.