Replacing a Substring With Another String in Bash
Learn how to replace a single or multiple occurrences of a substring inside a string in Bash.

Here's the scenario. You have a big string and you want to replace part of it with another string.
For example, you want to change "I am writing a line today" to "I am writing a line now".
In this quick tutorial, I'll show you how to replace a substring natively in Bash. I'll also show the sed command example as an extension.
Replace substring natively in bash (good for a single line)
Bash has some built-in methods for string manipulation. If you want to replace part of a string with another, this is how you do it:
${main_string/search_term/replace_term}
Create a string variable consisting of the line: “I am writing a line today” without the quotes and then replace today
with now
:
iborg@iborg-desktop:~$ line="I am writing a line today"
iborg@iborg-desktop:~$ echo "${line/today/now}"
I am writing a line now
Did you understand what just happened? In the syntax "${line/today/now}"
, line is the name of the variable where I've just stored the entire sentence. Here, I'm instructing it to replace the first occurrence of the word today
with now
. So instead of displaying the contents of the original variable, it showed you the line with the changed word.
Hence, the line
variable hasn't actually changed. It is still the same:
iborg@iborg-desktop:~$ echo $line
I am writing a line today
But you can definitely replace the word you want to and modify the same variable to make the changes permanent:
iborg@iborg-desktop:~$ line="${line/today/now}"
iborg@iborg-desktop:~$ echo $line
I am writing a line now
Now the changes have been made permanent and that's how you can permanently replace the first occurrence of a substring in a string.
You can also use other variables to store specific substrings that you wish to replace:
iborg@iborg-desktop:~$ replace="now"
iborg@iborg-desktop:~$ replacewith="today"
iborg@iborg-desktop:~$ line="${line/${replace}/${replacewith}}"
iborg@iborg-desktop:~$ echo $line
I am writing a line today
Here, I stored the word to be replaced in a variable called replace
and the word that it would be replaced with inside replacewith
. After that, I used the same method as discussed above to “revise” the line. Now, the changes I had made in the beginning of this tutorial have been reverted.
Let us look at another example:
iborg@iborg-desktop:~$ hbday="Happy Birthday! Many Many Happy Returns!"
iborg@iborg-desktop:~$ hbday="${hbday/Many/So Many}"
iborg@iborg-desktop:~$ echo $hbday
Happy Birthday! So Many Many Happy Returns!
Replacing all occurrences of a substring
You can also replace multiple occurrences of substrings inside strings. Let's see it through another example:
iborg@iborg-desktop:~$ hbday="${hbday//Many/So Many}"
iborg@iborg-desktop:~$ echo $hbday
Happy Birthday! So Many So Many Happy Returns!
That extra /
after hbday
made it replace all occurrences of Many
with So Many
inside the sentence.
Replace string using sed command (can work on files as well)
Here's another way for replacing substrings in a string in bash. Use the sed command in this manner:
Replace the first occurrence:
line=$(sed "s/$replace/s//$replacewith/" <<< "$line")
If I take the first example, it can be played as:
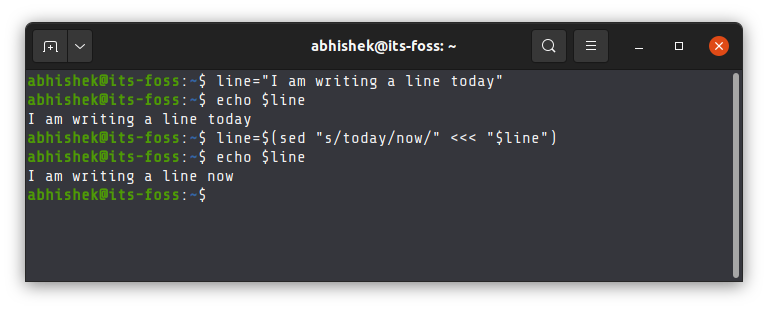
You can also replace all occurrences by adding g
at the end:
line=$(sed "s/$replace/$replacewith/g" <<< "$line")
Now, you may think this is more complicated than the native bash string method. Perhaps, but sed is very powerful and you can use it to replace all the occurrences of a string in a file.
sed -i 's/$replace/$replacewith/' filename
Here's an example of some of Agatha Christie's books with 'Murder' in the title:
iborg@iborg-desktop:~$ cat agatha.txt
The Murder in the Vicarage
Murder in Mesopotamia
Murder is Easy
Murder on the Orient Express
Murder In Retrospect
I am going to replace Murder with Marriage because some people think both are the same:
sed -i "s/Murder/Marriage/g" agatha.txt
And here's the changed file now:
The Marriage in the Vicarage
Marriage in Mesopotamia
Marriage is Easy
Marriage on the Orient Express
Marriage In Retrospect
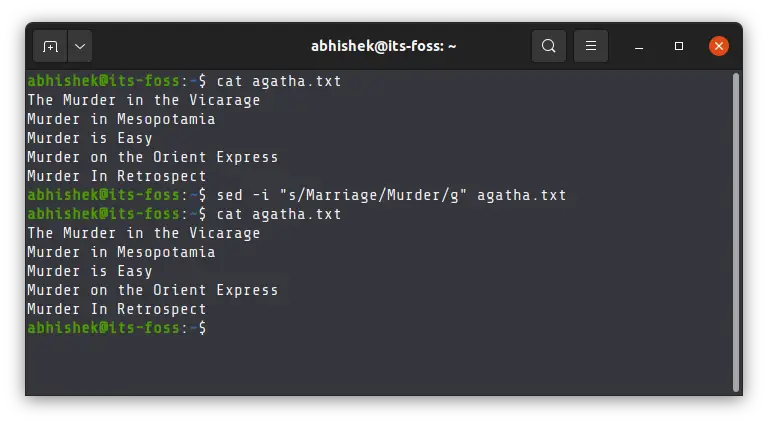
Sed is a very powerful tool for editing text files in Linux. You should at least learn its basics.
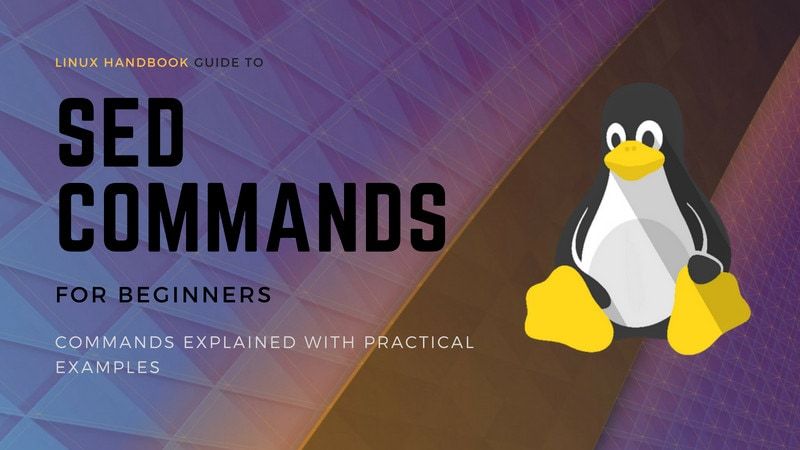
Now that you know about replacing substrings, how about splitting strings into substrings in bash?
Did you enjoy reading this article? If you have anything to share about replacing strings or this article, please do so in the comments below.
DevOps Geek at Linux Handbook | Doctoral Researcher on GPU-based Bioinformatics & author of 'Hands-On GPU Computing with Python' | Strong Believer in the role of Linux & Decentralization in Science