How to Split String in Bash Script
In this quick tip, you'll learn to split a string into an array in Bash script.

Let’s say you have a long string with several words separated by a comma or underscore. You want to split this string and extract the individual words.
You can split strings in bash using the Internal Field Separator (IFS) and read command or you can use the tr command. Let me show you how to do that with examples.
Method 1: Split string using read command in Bash
Here’s my sample script for splitting the string using read command:
#!/bin/bash
#
# Script to split a string based on the delimiter
my_string="Ubuntu;Linux Mint;Debian;Arch;Fedora"
IFS=';' read -ra my_array <<< "$my_string"
#Print the split string
for i in "${my_array[@]}"
do
echo $i
done
The part that split the string is here:
IFS=';' read -ra my_array <<< "$my_string"
Let me explain it to you. IFS determines the delimiter on which you want to split the string. In my case, it’s a semi colon. It could be anything you want like space, tab, comma or even a letter.
The IFS in the read command splits the input at the delimiter. The read command reads the raw input (option -r) thus interprets the backslashes literally instead of treating them as escape character. The option -a with read command stores the word read into an array in bash.
In simpler words, the long string is split into several words separated by the delimiter and these words are stored in an array.
Now you can access the array to get any word you desire or use the for loop in bash to print all the words one by one as I have done in the above script.
Here’s the output of the above script:
Ubuntu
Linux Mint
Debian
Arch
Fedora
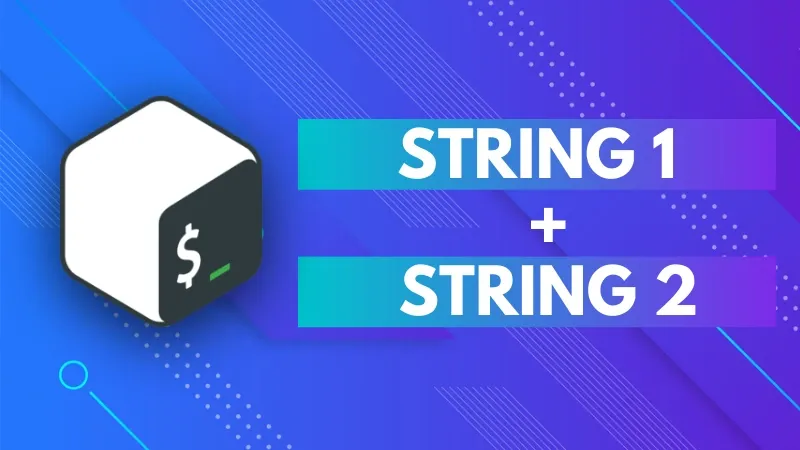
Method 2: Split string using tr command in Bash
This is the bash split string example using tr (translate) command:
#!/bin/bash
#
# Script to split a string based on the delimiter
my_string="Ubuntu;Linux Mint;Debian;Arch;Fedora"
my_array=($(echo $my_string | tr ";" "\n"))
#Print the split string
for i in "${my_array[@]}"
do
echo $i
done
This example is pretty much the same as the previous one. Instead of the read command, the tr command is used to split the string on the delimiter.
The problem with this approach is that the array element are divided on ‘space delimiter’. Because of that, elements like ‘Linux Mint’ will be treated as two words.
Here’s the output of the above script:
Ubuntu
Linux
Mint
Debian
Arch
Fedora
That’s the reason why I prefer the first method to split string in bash.
I hope this quick bash tutorial helped you in splitting the string. In a related post, you may also want to read about string comparison in bash.
And if you are absolutely new to Bash, read our Bash beginner tutorial series.
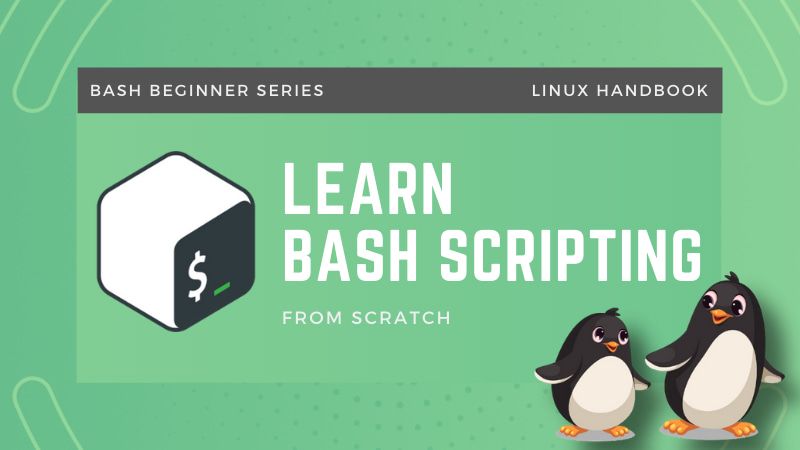
Creator of Linux Handbook and It's FOSS. An ardent Linux user & open source promoter. Huge fan of classic detective mysteries from Agatha Christie and Sherlock Holmes to Columbo & Ellery Queen.