Calculate Factorial of a Number
Here are a few sample example bash scripts to display the Fibonacci sequence based on a given input.
Time to practice your Bash scripting.
Exercise
In this Bash practice exercise, write a shell script that accepts a non-negative number and shows its factorial as output.
💡Hint: Factorial is calculated in this fashion:
N! = N x (N-1) x (N-2) x (N-3) x ... x 3 x 2 1
Remember that the factorial of 0 is 1. And you cannot have a factorial of negative numbers.
Test data
If you input 5, you should get the following (5 x 4 x 3 x 2 x 1):
120
If you input 7, you should get the following:
5040
Solution 1: Factorial bash script using recursive function
Here's a sample bash scripting for getting factorial of a given number using only a for loop.
I have added the option step for checking that non-negative numbers are not enetered.
#!/bin/bash
read -p "Enter a non-negative number: " num
if [ $num -lt 0 ]; then
echo "You entered a negative number"
exit
fi
fact=1
for (( i=1; i<=$num; i++ )); do
fact=$(($fact*$i))
done
echo "$num! is: $fact"
Solution 2: Factorial script using factorial in bash
Here's a bash shell script that uses the concept of recursion and function:
#!/bin/bash
read -p "Enter a non-negative number: " num
if [ $num -lt 0 ]; then
echo "You entered a negative number"
exit
fi
factorial () {
if [ $1 -le 1 ]; then
echo 1
else
last=$(factorial $(( $1 -1)))
echo $(( $1 * last ))
fi
}
echo -n "$num! is: "
factorial $num
-n
? It is used to stop echo from automatically adding a newline.📖 Concepts to revise
The solutions discussed here use some terms, commands and concepts and if you are not familiar with them, you should learn more about them.
📚 Further reading
If you are new to bash scripting, we have a streamlined tutorial series on Bash that you can use to learn it from scratch or use it to brush up the basics of bash shell scripting.
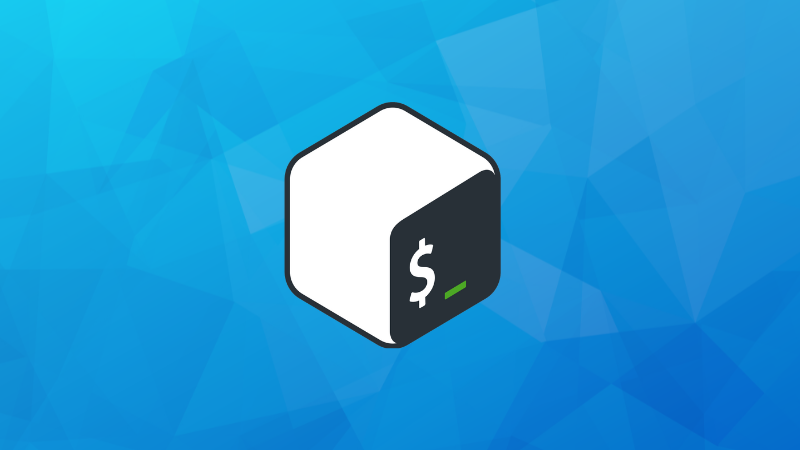
Creator of Linux Handbook and It's FOSS. An ardent Linux user & open source promoter. Huge fan of classic detective mysteries from Agatha Christie and Sherlock Holmes to Columbo & Ellery Queen.