How to Use echo Command Without Newline
Every time you use echo, it adds a newline character at the end. Here's what you can do if you want to use echo without newline.
— Team LHB

The echo command is a handy way of printing anything in shell scripts.
By default, it always adds a new line character. So if you run multiple echo commands, each output is displayed on a new line.
But this could be inconvenient in situations where you need to print with echo command but without the newline.
Imagine yourself trying to print the Fibonacci series: 1, 1, 2, 3, 5, 8, 13, 21, 34, 55
You can use the -n
flag of the echo command to print without newline:
echo -n "Hello World"
You'll notice that the prompt is displayed in the same line immediately after the Hello World now.
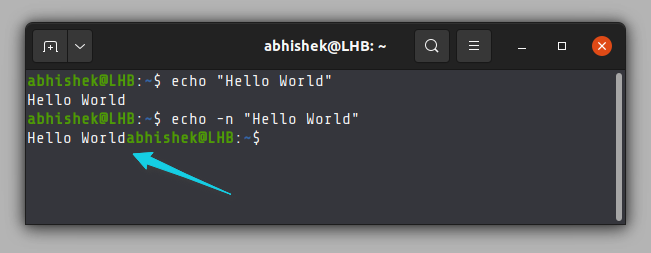
Let's take a more in-depth look at it.
Printing echo command without new line
Let's take a simple for loop that prints the contents of an array in bash script and check it's output.
$ bash_array=(1 2 3 4)
$ for i in ${bash_array[@]}; do
echo $i
done
1
2
3
4
As you can see, each time the echo command is executed, the output is displayed on a newline. Sometimes that is what you want, other times, you want the output to continue on the same line without starting on the newline.
Meet the "-n" flag
The bash implementation of the echo command usually appends a "\n" (an escape character to signify a newline) to the output. The "-n" flag does not append a "\n" to the output.
$ bash_array=(1 2 3 4 5 6 7 8)
$ for i in ${bash_array[@]}; do
echo -n $i
done
12345678
As you can see, all the numbers are now displayed in a single line. This means each time that the echo command was run, it did not output to a newline.
echo -n didn't work. Now what?
In some cases, using echo -n
also prints the -n
character instead of printing the text without newline.
That's possible if there is a separate implementation of the echo command by the shell you are using.
Confused? Some distributions and shells may have their own implementation of some built-in shell commands. echo is one such command.
Here are a few things you may try.
Add the shebang character followed by /bin/bash to ensure that your shell script uses bash shell.
#!/bin/bash
If that doesn't work, you may try using the printf command instead of echo.
By default, printf doesn't add the newline character:
printf "Something without newline"
You have to explicitly provide the newline character \n:
printf "Something with newline\n"
The printf command behaves like the printf command in C and you can use it to print more complicated formatted outputs.
The printf command has much more consistent behavior. echo is fine for simple things, but I advise using printf for anything more complicated.
I hope this simple tutorial helps you in controlling the behavior of new line in bash scripts.
Team LHB indicates the effort of a single or multiple members of the core Linux Handbook team.