21 Essential Commands Every Docker User Should Know
A compilation of 21 executable and informative Docker commands for your quick reference.

Docker has an extensive set of commands (subcommands to be more precise). You cannot possibly use all of them and there is no need to go for that achievement as well.
Most of the time you'll be using a certain subset of commands for managing containers and images.
I am going to list such common but essential Docker commands that are extremely useful for day to day use by Docker users and admins.
I have divided these Docker commands into two categories:
- Executable: Commands that are used for running and managing containers
- Informative: Commands that are used for extracting information about containers, images and layers.
Sounds good? Let's see these must know Docker commands along with practical examples.
Docker execute commands [for running and managing containers]
These Docker commands are used for managing containers and building images. They execute a specific task and hence I termed them 'Docker execute commands'.
At this point, I recommend that you refresh your basic of the Docker container lifecycle with this image:
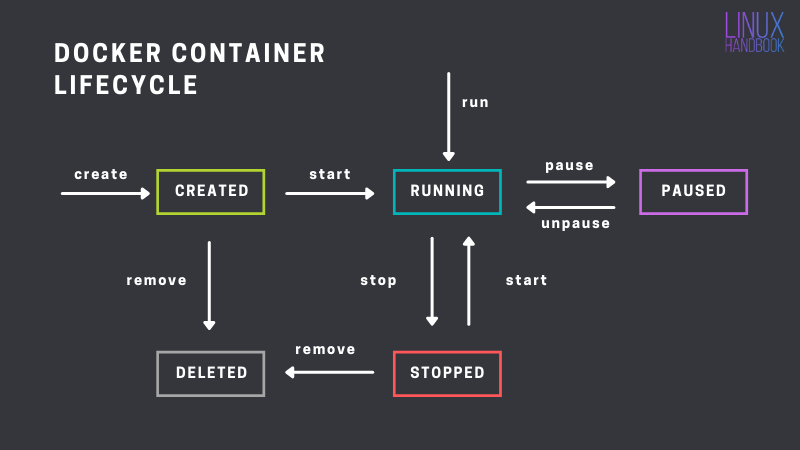
With that aside, let's see the commands and their examples.
1. Docker run
Docker run is used to create a container and start it immediately.
This command first looks for an existing image for the container to be run and if not found, pulls it from the repository, creates a container from the image and then starts running it immediately.
Let's run a Nginx container from the official repository.
avimanyu@localhost:~$ docker run --name nginx-root -p 80:80 -d nginx
8411c3df0fd7f57395b0732b1b1963c215040eed669dc8327200db197ff6099b
avimanyu@localhost:~$
With the --name
flag, you customize its local name and also map the host port to the container port. -d
or --detach
helps in running the container in the background in detached mode.
2. Docker stop
This command is used to stop containers that are already running. The syntax is simple:
docker stop container-name-or-id
Let’s stop the container that you started in the first command.
avimanyu@localhost:~$ docker stop nginx-root
nginx-root
avimanyu@localhost:~$
In case you did not customize a name when you ran it, you can check the randomly generated name with docker ps command (discussed later).
When you use the docker stop command, it sends SIGTERM signal requesting termination after which the SIGKILL signal is administered depending upon the termination situation.
3. Docker start
This is used to start containers that have been stopped. The syntax is simple:
docker start container-name-or-id
Let’s start the Nginx container you just stopped in the previous example.
avimanyu@localhost:~$ docker start nginx-root
nginx-root
avimanyu@localhost:~$
Please note that unlike docker run, docker start cannot create new containers from images. It can only 'run' the containers that are in stopped state.
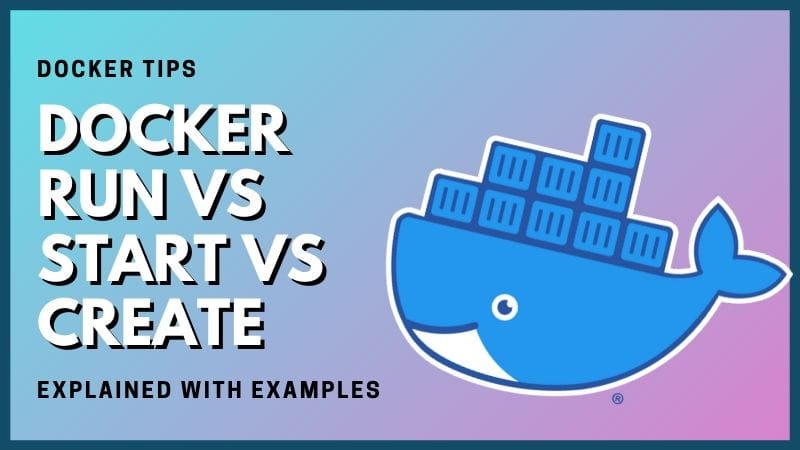
4. Docker exec
docker exec
, as the name suggests, is used for the execution of new commands within a container that’s already running.
avimanyu@localhost:~$ docker exec -ti nginx-root /bin/bash
root@72acb924f667:/#
Now you’re running a bash shell inside the container.
In -ti
, t denotes a "terminal" and i denotes "interactive" to keep STDIN (standard input) open even if not attached.
To exit the container shell, just use the exit command to return to your host console.
root@72acb924f667:/# exit
exit
avimanyu@localhost:~$
5. Docker rename
To rename a container, you use the following syntax:
docker rename old_container_name new_container_name
Let’s rename the nginx-root container to nginx-toor. Note that you can do this even when the container is up and running!
avimanyu@localhost:~$ docker rename nginx-root nginx-toor
6. Docker pause/unpause
With docker pause
, you can suspend all processes in a specified container. The SIGSTOP signal is used, which is noticed by the process under suspension.
avimanyu@localhost:~$ docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
72acb924f667 nginx "nginx -g 'daemon of…" 4 hours ago Up 4 hours 0.0.0.0:80->80/tcp nginx-toor
e2dd68fdd220 wordpress:5.3.0-php7.1-apache "docker-entrypoint.s…" 3 days ago Up 3 days 0.0.0.0:8088->80/tcp wp2_wordpress_1
016709c05add wordpress:5.3.0-php7.1-apache "docker-entrypoint.s…" 3 days ago Up 3 days 0.0.0.0:8080->80/tcp wp1_wordpress_1
656f6761ce0d mariadb:10.4.10-bionic "docker-entrypoint.s…" 8 days ago Up 3 days 3306/tcp wp2_mysql_1
ee953bb14483 mariadb:10.4.10-bionic "docker-entrypoint.s…" 8 days ago Up 3 days 3306/tcp wp1_mysql_1
Let’s pause the nginx-toor container:
avimanyu@localhost:~$ docker pause nginx-toor
nginx-toor
Now you can check whether it really got paused with the docker ps
command again:
avimanyu@localhost:~$ docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
72acb924f667 nginx "nginx -g 'daemon of…" 4 hours ago Up 4 hours (Paused) 0.0.0.0:80->80/tcp nginx-toor
e2dd68fdd220 wordpress:5.3.0-php7.1-apache "docker-entrypoint.s…" 3 days ago Up 3 days 0.0.0.0:8088->80/tcp wp2_wordpress_1
016709c05add wordpress:5.3.0-php7.1-apache "docker-entrypoint.s…" 3 days ago Up 3 days 0.0.0.0:8080->80/tcp wp1_wordpress_1
656f6761ce0d mariadb:10.4.10-bionic "docker-entrypoint.s…" 8 days ago Up 3 days 3306/tcp wp2_mysql_1
ee953bb14483 mariadb:10.4.10-bionic "docker-entrypoint.s…" 8 days ago Up 3 days 3306/tcp wp1_mysql_1
To revert, simply use unpause:
avimanyu@localhost:~$ docker unpause nginx-toor
nginx-toor
7. Docker kill
docker kill
is used to send the default KILL signal to a container. Unlike docker stop
, it directly sends a SIGKILL signal without requesting termination with the SIGTERM signal.
It is an abrupt way to force termination based on typical emergency situations and therefore it's always recommended to use docker stop
first.
avimanyu@localhost:~$ docker kill nginx-root
nginx-root
avimanyu@localhost:~$
8. Docker build
If you are modifying Docker image, you need to build your custom image before you can use it to deploy new containers.
docker build
is the command to build such modified images.
I recommend reading this detailed guide on using Dockerfile for creating custom Docker images. Here, I'll just show a quick example.
Here's a sample Dockerfile:
FROM alpine:latest
RUN apk update
RUN apk add vim
First it downloads an Alpine Linux image from the official repository. Then it updates the package cache and then additionally install the Vim editor into it.
This means that the container running from this image will have Vim editor preinstalled.
Build the image in the following manner:
avimanyu@localhost:~/docker-build-test$ docker image build -t docker-build-test-image .
Sending build context to Docker daemon 2.048kB
Step 1/3 : FROM alpine:latest
---> 965ea09ff2eb
Step 2/3 : RUN apk update
---> Using cache
---> b16ea26b03aa
Step 3/3 : RUN apk add vim
---> Using cache
---> b283eaf606c1
Successfully built b283eaf606c1
Successfully tagged docker-build-test-image:latest
The modified image is named docker-build-test-image and the command looks for the Dockerfile in the current directory.
9. Docker cp
The cp command is already well-known with experienced Linux users to copy files and directories between different locations.
Similarly, docker container cp
, or simply docker cp
is used to copy files and directories between the container and host.
avimanyu@localhost:~/nginx-root$ docker cp nginx-root:/etc/nginx/nginx.conf ./config
The above command will copy the nginx.conf file from the container nginx-root to a directory named config that must already be residing in the present working directory (also named nginx-root). Note the similar file system structure(/etc/nginx) of the container just as a regular Linux system would be like.
10. Docker rm
Similar to the removal of files and directories through the Linux terminal, Docker also uses a similar syntax to remove both images and containers.
docker rm container_or_image_name_or_id
Try removing the nginx-root container that you have created previously in this tutorial:
avimanyu@localhost:~$ docker rm nginx-root
nginx-root
The output won't say container removed or anything of that sort, but it is removed nonetheless.
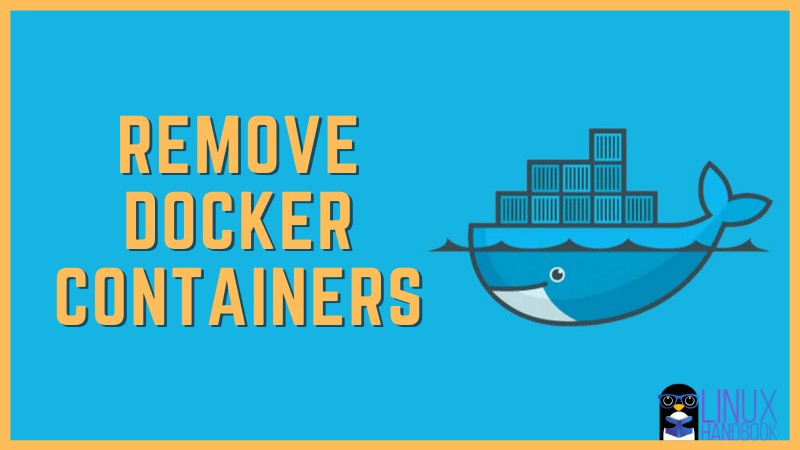
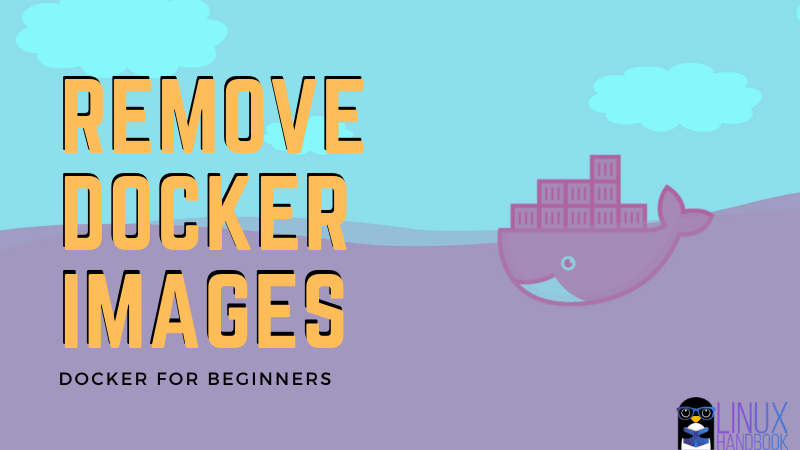
Let's summarize all the above commands:
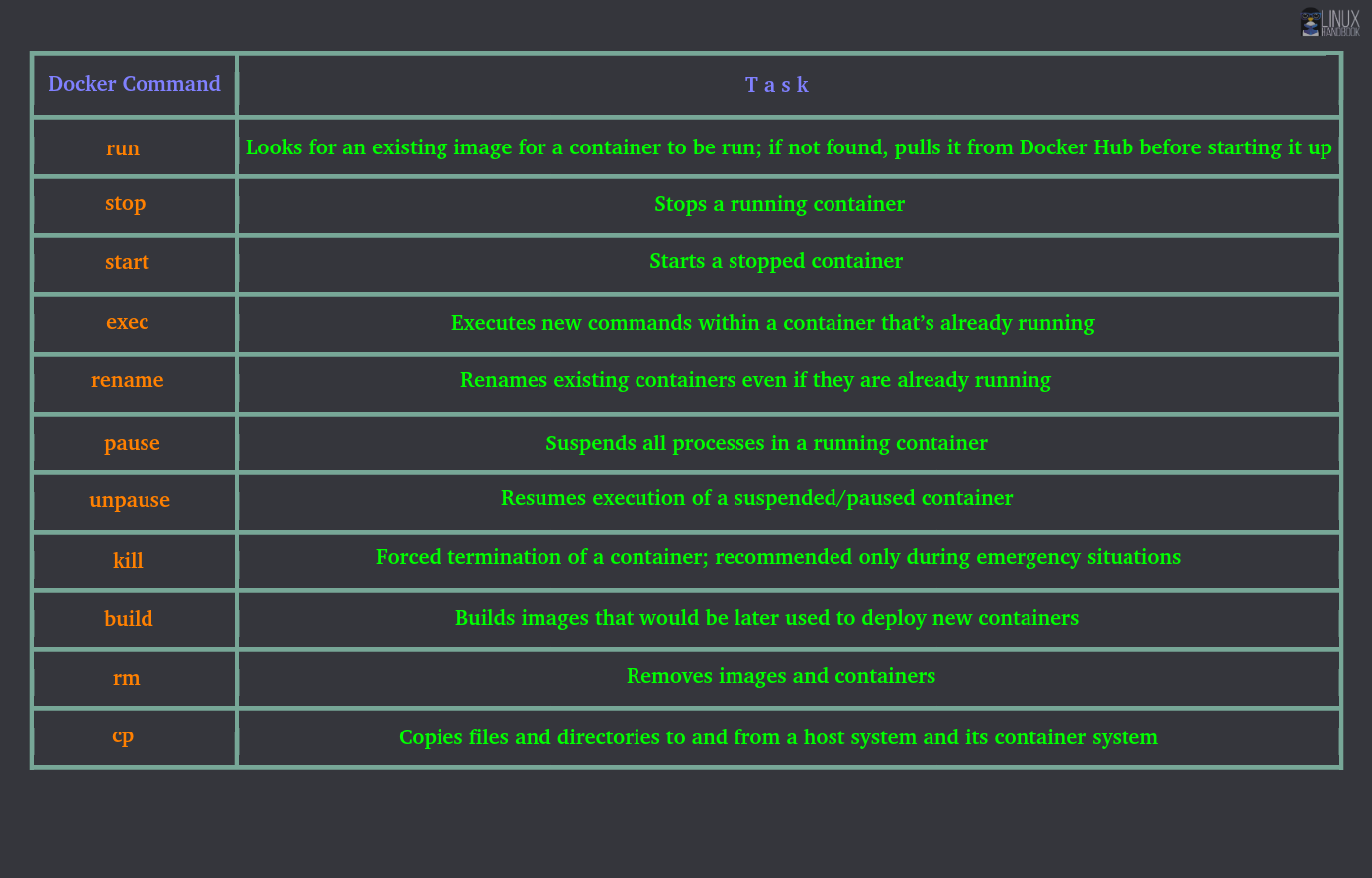
I'll create nginx-root container again to use it in some other command examples.
Docker informative commands [for getting information about containers and images]
Docker commands that provide you information related to specific tasks and existing readable parameters can be called as informative docker commands.
Here, I'm enlisting 11 Docker commands for this purpose:
11. Docker ps/container ls
Short for docker container ls
, docker ps can be used to list all the containers that are currently running on a system and also check their status with additional statistics.
avimanyu@localhost:~$ docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
e2dd68fdd220 wordpress:5.3.0-php7.1-apache "docker-entrypoint.s…" 3 days ago Up 3 days 0.0.0.0:8088->80/tcp wp2_wordpress_1
016709c05add wordpress:5.3.0-php7.1-apache "docker-entrypoint.s…" 3 days ago Up 3 days 0.0.0.0:8080->80/tcp wp1_wordpress_1
80e12e4a431c nginx "nginx -g 'daemon of…" 3 days ago Up 3 days 0.0.0.0:80->80/tcp nginx-root
656f6761ce0d mariadb:10.4.10-bionic "docker-entrypoint.s…" 7 days ago Up 3 days 3306/tcp wp2_mysql_1
ee953bb14483 mariadb:10.4.10-bionic "docker-entrypoint.s…" 7 days ago Up 3 days 3306/tcp wp1_mysql_1
avimanyu@localhost:~$
12. Docker images/image ls
If you are a seasoned Linux user, you might already be familiar with the ls
command that is used for checking directory contents with the Linux terminal.
Similarly, in Docker, docker image ls
or simply docker images
is used to check the list of all Docker images existing within a system. These images may be official or custom depending on how they were built.
avimanyu@localhost:~$ docker images
REPOSITORY TAG IMAGE ID CREATED SIZE
nginx latest 4152a9608752 10 days ago 126MB
avimanyu786/flask-test latest f8e2d6f524ef 2 weeks ago 122MB
avimanyu786/nginx-test latest 5fe5e88b9eaa 2 weeks ago 23MB
wordpress 5.3.0-php7.1-apache f0985bcc2ffb 2 weeks ago 530MB
mariadb 10.4.10-bionic c1c9e6fba07a 2 weeks ago 355MB
avimanyu786/alpine-with-vim latest b283eaf606c1 2 weeks ago 34.7MB
jwilder/nginx-proxy latest d1c0beda6804 3 weeks ago 161MB
nginx alpine b6753551581f 5 weeks ago 21.4MB
python alpine 204216b3821e 5 weeks ago 111MB
alpine latest 965ea09ff2eb 5 weeks ago 5.55MB
mysql latest c8ee894bd2bd 6 weeks ago 456MB
hello-world latest fce289e99eb9 11 months ago 1.84kB
avimanyu@localhost:~$
Apart from containers and images, ls
is also used for similar purposes on Docker to manage networks, volumes, nodes and swarm services.
13. Docker logs
This command is very helpful on production servers in investigating and resolving issues. You can fetch the logs of any container with help of the docker logs command.
docker logs container_name_or_id
For example:
avimanyu@localhost:~$ docker logs nginx-toor
178.130.157.61 - - [30/Nov/2019:07:56:14 +0000] "GET / HTTP/1.1" 200 612 "-" "Mozilla/5.0 (Windows NT 10.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/51.0.2704.103 Safari/537.36" "-"
178.130.157.61 - - [30/Nov/2019:07:56:14 +0000] "GET / HTTP/1.1" 200 612 "-" "Mozilla/5.0 (Windows NT 6.1; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/52.0.2743.116 Safari/537.36" "-"
.
.
.
.
x64|'|'|No|'|'|0.7d|'|'|..|'|'|AA==|'|'|112.inf|'|'|SGFjS2VkDQoxOTIuMTY4LjkyLjIyMjo1NTUyDQpEZXNrdG9wDQpjbGllbnRhLmV4ZQ0KRmFsc2UNCkZhbHNlDQpUcnVlDQpGYWxzZQ==12.act|'|'|AA==" 400 157 "-" "-" "-"
8.36.123.216 - - [30/Nov/2019:10:32:20 +0000] "GET / HTTP/1.1" 200 612 "-" "Mozilla/4.0 (compatible; MSIE 6.0; Windows NT 5.1)" "-"
202.142.105.74 - - [30/Nov/2019:12:03:39 +0000] "GET /favicon.ico HTTP/1.1" 404 153 "-" "Mozilla/5.0 (X11; Ubuntu; Linux x86_64; rv:70.0) Gecko/20100101 Firefox/70.0" "-"
14. Docker version
To obtain detailed information on the Docker version installed on the system, simply use:
docker version
Here's what this information looks like:
avimanyu@localhost:~$ docker version
Client: Docker Engine - Community
Version: 19.03.4
API version: 1.40
Go version: go1.12.10
Git commit: 9013bf583a
Built: Fri Oct 18 15:54:09 2019
OS/Arch: linux/amd64
Experimental: false
Server: Docker Engine - Community
Engine:
Version: 19.03.4
API version: 1.40 (minimum version 1.12)
Go version: go1.12.10
Git commit: 9013bf583a
Built: Fri Oct 18 15:52:40 2019
OS/Arch: linux/amd64
Experimental: false
containerd:
Version: 1.2.10
GitCommit: b34a5c8af56e510852c35414db4c1f4fa6172339
runc:
Version: 1.0.0-rc8+dev
GitCommit: 3e425f80a8c931f88e6d94a8c831b9d5aa481657
docker-init:
Version: 0.18.0
GitCommit: fec3683
avimanyu@localhost:~$
15. Docker info
The following command will show you system wide information on the host system where Docker is installed:
avimanyu@localhost:~$ docker info
Client:
Debug Mode: false
Server:
Containers: 9
Running: 5
Paused: 0
Stopped: 4
Images: 17
Server Version: 19.03.4
Storage Driver: overlay2
Backing Filesystem: extfs
Supports d_type: true
Native Overlay Diff: true
Logging Driver: json-file
Cgroup Driver: cgroupfs
Plugins:
Volume: local
Network: bridge host ipvlan macvlan null overlay
Log: awslogs fluentd gcplogs gelf journald json-file local logentries splunk syslog
Swarm: inactive
Runtimes: runc
Default Runtime: runc
Init Binary: docker-init
containerd version: b34a5c8af56e510852c35414db4c1f4fa6172339
runc version: 3e425f80a8c931f88e6d94a8c831b9d5aa481657
init version: fec3683
Security Options:
apparmor
seccomp
Profile: default
Kernel Version: 4.15.0-50-generic
Operating System: Ubuntu 18.04.2 LTS
OSType: linux
Architecture: x86_64
CPUs: 1
Total Memory: 985.4MiB
Name: localhost
ID: MZTJ:L5UF:WMQ3:VOIO:NR3N:336Q:YX2T:MSOU:5Y2N:MA7V:F6BQ:6UDY
Docker Root Dir: /var/lib/docker
Debug Mode: false
Registry: https://index.docker.io/v1/
Labels:
Experimental: false
Insecure Registries:
127.0.0.0/8
Live Restore Enabled: false
WARNING: No swap limit support
avimanyu@localhost:~$
16. Docker inspect
The docker inspect command is used to reveal low-level information about both containers and images.
You can get information like the checksum of the image, layers, container IP address and other networking information and a lot of other information.
It is wise to use grep command to filter the output and get only the desired information.
In the following example, I inspect an existing Docker image named avimanyu786/alpine-with-vim:latest.
avimanyu@localhost:~$ docker inspect avimanyu786/alpine-with-vim:latest
[
{
"Id": "sha256:b283eaf606c18a4d0cc461ffdf86d53ecb173db5271129090a7421a64b1c514e",
"RepoTags": [
"avimanyu786/alpine-with-vim:latest"
],
"RepoDigests": [],
"Parent": "sha256:b16ea26b03aaf5d9bd84157fbef61670d2f318c1af854ae04f0dbc290afd4b04",
"Comment": "",
"Created": "2019-11-12T11:51:24.458552779Z",
"Container": "5b759e6f98e354a78dfca588eb65316b35f0cfb88c9119844471c8fcba362898",
"ContainerConfig": {
"Hostname": "",
"Domainname": "",
"User": "",
"AttachStdin": false,
"AttachStdout": false,
"AttachStderr": false,
"Tty": false,
"OpenStdin": false,
"StdinOnce": false,
"Env": [
"PATH=/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin"
],
"Cmd": [
"/bin/sh",
"-c",
"apk add vim"
],
"Image": "sha256:b16ea26b03aaf5d9bd84157fbef61670d2f318c1af854ae04f0dbc290afd4b04",
.
.
.
.
"Metadata": {
"LastTagTime": "2019-11-12T11:51:24.563369343Z"
}
}
avimanyu@localhost:~$
17. Docker history
Find out the history of any image existing on your Docker system with docker history image-name.
The history of the image will tell you the changes and commits made in reverse chronological order.
avimanyu@localhost:~$ docker history avimanyu786/alpine-with-vim:latest
IMAGE CREATED CREATED BY SIZE COMMENT
b283eaf606c1 2 weeks ago /bin/sh -c apk add vim 27.7MB
b16ea26b03aa 2 weeks ago /bin/sh -c apk update 1.42MB
965ea09ff2eb 5 weeks ago /bin/sh -c #(nop) CMD ["/bin/sh"] 0B
<missing> 5 weeks ago /bin/sh -c #(nop) ADD file:fe1f09249227e2da2… 5.55MB
avimanyu@localhost:~$
18. Docker port
This command can prove very useful when managing multiple containers because it reveals host to container port mappings very easily:
avimanyu@localhost:~$ docker port nginx-root
80/tcp -> 0.0.0.0:80
Here you can see that host port 80 is mapped to container port 80 for the container named nginx-root.
19. Docker diff
With docker diff, you can inspect changes to files or directories on a container’s file system. It lists the changed files and directories in a container᾿s filesystem since it was created.
Three different types of change are tracked (A/D/C):
- A: Addition of a file or directory
- D: Deletion of a file or directory
- C: Change of a file or directory
Let's check out the same for our nginx-root container:
avimanyu@localhost:~$ docker diff nginx-root
C /run
A /run/nginx.pid
C /var
C /var/cache
C /var/cache/nginx
A /var/cache/nginx/client_temp
A /var/cache/nginx/fastcgi_temp
A /var/cache/nginx/proxy_temp
A /var/cache/nginx/scgi_temp
A /var/cache/nginx/uwsgi_temp
avimanyu@localhost:~$
20. Docker top
Once again, top is a Linux command you might already be familiar with. It is used for listing running processes on the system.
With docker top, you list the processes running within a container.
docker top container_ID_or_name
If I do it with nginx-root in our exmaple, it shows the following value.
avimanyu@localhost:~$ docker top nginx-root
UID PID PPID C STIME TTY TIME CMD
root 10355 10330 0 07:54 ? 00:00:00 nginx: master process nginx -g daemon off;
systemd+ 10401 10355 0 07:54 ? 00:00:00 nginx: worker process
root 12054 10330 0 10:22 pts/0 00:00:00 /bin/bash
avimanyu@localhost:~$
21. Docker stats
The docker stats command can be used as is and also for specific containers. It shows you container details along-with system resource usage.
First, let’s look at its generic form:
avimanyu@localhost:~$ docker stats
CONTAINER ID NAME CPU % MEM USAGE / LIMIT MEM % NET I/O BLOCK I/O PIDS
72acb924f667 nginx-root 0.00% 2.621MiB / 985.4MiB 0.27% 8.02kB / 8.92kB 6.8MB / 0B 3
e2dd68fdd220 wp2_wordpress_1 0.00% 44.24MiB / 985.4MiB 4.49% 1.9MB / 1.62MB 53.3MB / 61.4kB 11
016709c05add wp1_wordpress_1 0.00% 68.52MiB / 985.4MiB 6.95% 12MB / 4.77MB 136MB / 61.4kB 11
656f6761ce0d wp2_mysql_1 0.05% 70.12MiB / 985.4MiB 7.12% 1.05MB / 1MB 16MB / 2.15MB 30
ee953bb14483 wp1_mysql_1 0.04% 31.73MiB / 985.4MiB 3.22% 1.16MB / 4.76MB 50.6MB / 91.8MB 31
The above output will be continuously generated in real time until you exit out with Ctrl+C.
Now, if you try it for a specific container, you’ll see the same output but for that container alone:
avimanyu@localhost:~$ docker stats nginx-root
CONTAINER ID NAME CPU % MEM USAGE / LIMIT MEM % NET I/O BLOCK I/O PIDS
72acb924f667 nginx-root 0.00% 2.621MiB / 985.4MiB 0.27% 8.02kB / 8.92kB 6.8MB / 0B 3
Let me summarize the information Docker commands as well:
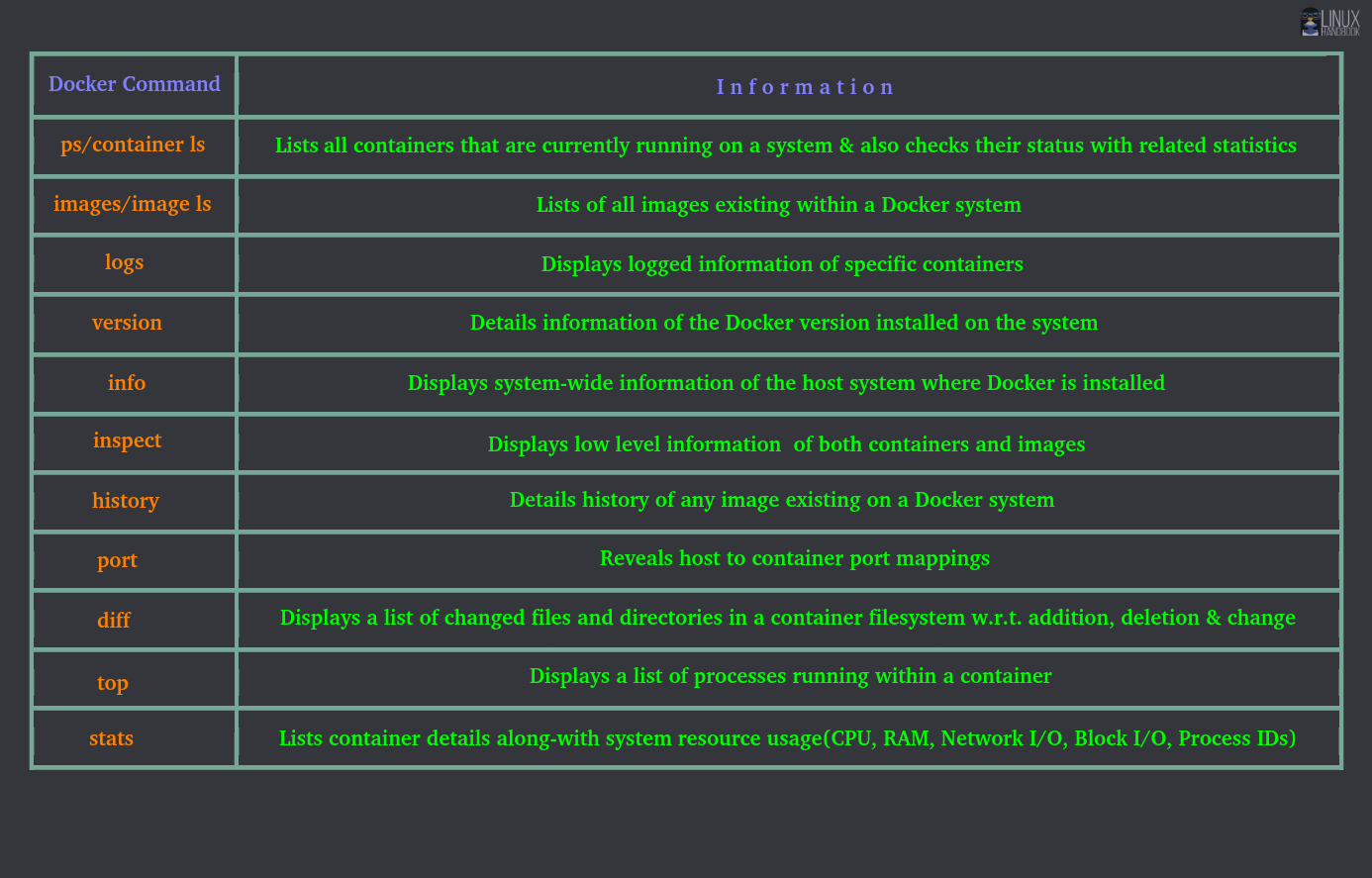
For an exhaustive and comprehensive resource of all Docker commands, you may always refer to Docker’s official documentation.
You'll notice a resemblance between the usual Linux commands and some Docker commands. I think it's deliberate to make it easier to memorize the Docker commands.
Hope you find this article useful in your day-to-day Docker usage. If you have more of such useful commands to share, please feel free to share them below. Feedback and suggestions are always welcome.
DevOps Geek at Linux Handbook | Doctoral Researcher on GPU-based Bioinformatics & author of 'Hands-On GPU Computing with Python' | Strong Believer in the role of Linux & Decentralization in Science