How to Concatenate Strings in Bash
Learn to concatenate strings in bash shell scripts. You'll also learn to append to existing strings and combine strings and integers.

Concatenating strings can be an important part of using any programming language for practical applications.
You can concatenate strings in bash as well. There is no concatenation operator here. Just write the strings one after another to join strings in Bash.
concat_string="$str1$str2"
Don't worry! Iβll show you various actual examples to concatenate strings in bash.
Assigning concatenated strings
There are no data types in Bash like you have in most programming languages. But you can still declare variables in Bash.
Here is how you assign strings in Bash:
avimanyu@linuxhandbook:~$ w='Welcome'
You can use printf command to print the value of this string variable:
avimanyu@linuxhandbook:~$ printf "$w\n"
Welcome
Let's create some more strings:
avimanyu@linuxhandbook:~$ t='To'
avimanyu@linuxhandbook:~$ l='Linux'
avimanyu@linuxhandbook:~$ h='Handbook!'
I want to combine all these string variables into a single one. How to do that?
avimanyu@linuxhandbook:~$ tony="${w} ${t} ${l} ${h}"
In this manner, I have concatenated all four strings into a single variable and named it tony
. Do note that I have added a space between the variables.
Let's quickly confirm that the strings have been combined:
avimanyu@linuxhandbook:~$ printf "$tony\n"
Welcome To Linux Handbook!
Here's all of it in a Bash Script:
#!/bin/bash
w='Welcome'
t='To'
l='Linux'
h='Handbook'
tony="${w} ${t} ${l} ${h}"
printf "${tony}\n"
Make it executable and run it as a script:
avimanyu@linuxhandbook:~$ chmod +x concat.sh
avimanyu@linuxhandbook:~$ ./concat.sh
Welcome To Linux Handbook!
Append to string in bash
The above example combines different strings into one.
Let's take another scenario. Say, you want to append to an already existing string. How to do that? You use the wonderful += operator.
str="iron"
str+="man"
Can you guess the new value of str
? Yes! It is ironman
.
avimanyu@linuxhandbook:~$ str="iron"
avimanyu@linuxhandbook:~$ str+="man"
avimanyu@linuxhandbook:~$ echo $str
ironman
This is helpful when you are using loops in bash. Take this for loop for example:
#!/bin/bash
var=""
for color in 'Black' 'White' 'Brown' 'Yellow'; do
var+="${color} "
done
echo "$var"
If you run the above script, it will append to the string after each iteration.
Black White Brown Yellow
Concatenate numbers and strings
As I mentioned previously, there are no data types in Bash. Strings and integers are the same and hence they can be easily joined in a single string.
Let us look at another example through a second script. This time, I'll use a number:
#!/bin/bash
we='We'
lv='Love'
y='You'
morgan=3000
stark="${we} ${lv} ${y} ${morgan}!!!"
printf "${stark}\n"
Execution:
avimanyu@linuxhandbook:~$ chmod +x morgan.sh
avimanyu@linuxhandbook:~$ ./morgan.sh
We Love You 3000!!!
Nested concatenation of strings
You can also store those two concatenated strings inside a third one through nested concatenation:
#!/bin/bash
w='Welcome'
t='To'
l='Linux'
h='Handbook'
tony="${w} ${t} ${l} ${h}"
we='We'
lv='Love'
y='You'
morgan=3000
stark="${we} ${lv} ${y} ${morgan}!!!"
ironman="${tony}..${stark}"
printf "${ironman} Forever!\n"
When you run this shell script, you'll see this output:
Welcome To Linux Handbook..We Love You 3000!!! Forever!
Conclusion
If you are new to shell scripting, I highly recommend our Bash tutorial series for beginners.
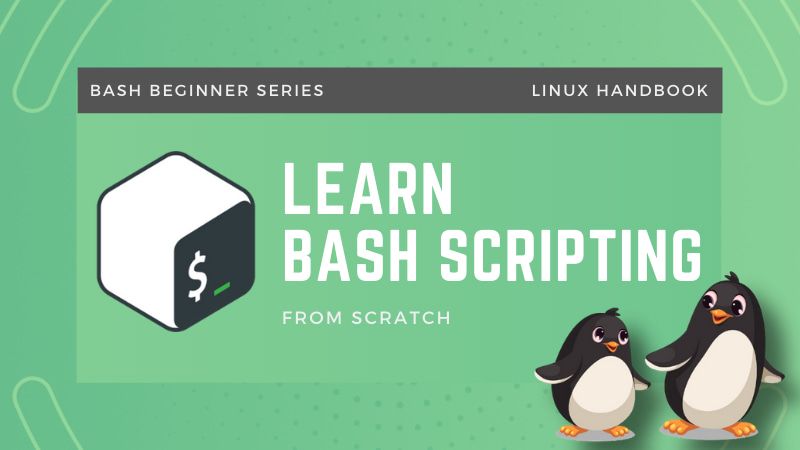
I hope this quick little tutorial helped you in concatenating bash strings. If you have questions or suggestions, feel free to leave a comment below.
DevOps Geek at Linux Handbook | Doctoral Researcher on GPU-based Bioinformatics & author of 'Hands-On GPU Computing with Python' | Strong Believer in the role of Linux & Decentralization in Science