Introduction to Ansible Modules
Learn all about modules in Ansible. What are modules, why, and how they are used? Also learn about some popular Ansible commands.

Ansible is a powerful automation tool that simplifies the management and configuration of systems.
At the heart of Ansible's functionality are modules, which are reusable scripts designed to perform specific tasks on remote hosts.
These modules allow users to automate a wide range of tasks, from installing packages to managing services, all with the aim of maintaining their systems' desired state.
This article will explain what Ansible modules are, how to use them, and provide real-world examples to demonstrate their effectiveness.
What is an Ansible Module?
An Ansible module is a reusable, standalone script that performs a specific task or operation on a remote host. Modules can manage system resources like packages, services, files, and users, among other things. They are the building blocks for creating Ansible playbooks, which define the automation workflows for configuring and managing systems.
Ansible modules are designed to be idempotent, meaning they ensure that the system reaches a desired state without applying changes that are unnecessary if the system is already in the correct state. This makes Ansible operations predictable and repeatable.
Modules can be written in any programming language, but most are in Python. Ansible ships with a large number of built-in modules, and there are also many community-contributed modules available.
Additionally, you can write custom modules to meet specific needs.
Here's a simple syntax to get you started:
---
- name: My task name
hosts: group_name # Group of hosts to run the task on
become: true # Gain root privileges (if needed)
module_name:
arguments: # Module specific arguments
This is a basic template for defining tasks in your Ansible playbooks.
Ansible Modules - Real-world examples
Let's examine some real-world examples to understand how modules work in action.
Example 1: Installing a package
Let's use the yum
module to install the Apache web server on a RockyLinux.
---
- name: Install Apache web server
hosts: webservers
tasks:
- name: Install httpd package
yum:
name: httpd
state: present
In this playbook:
- The
hosts
directive specifies that this playbook will run on hosts in thewebservers
group. - The
yum
module is used to ensure that thehttpd
package is installed.
Let's run the above playbook:
ansible-playbook playbook.yml
After the successful playbook execution, you will see the following output:
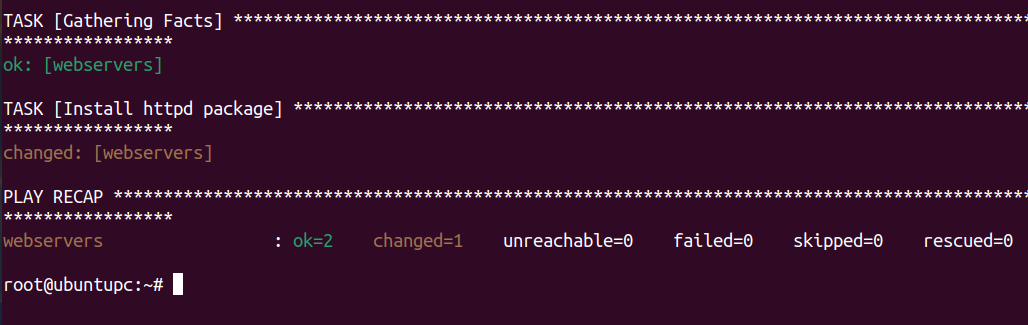
Example 2: Managing services
Now, let's use the service
module to ensure that the Apache web server is started and enabled to start on boot.
---
- name: Ensure Apache is running and enabled
hosts: webservers
tasks:
- name: Start and enable httpd service
service:
name: httpd
state: started
enabled: yes
In this playbook:
- The
service
module is used to start thehttpd
service and enable it to start at boot.
Now, run the above playbook:
ansible-playbook playbook.yml
Output:
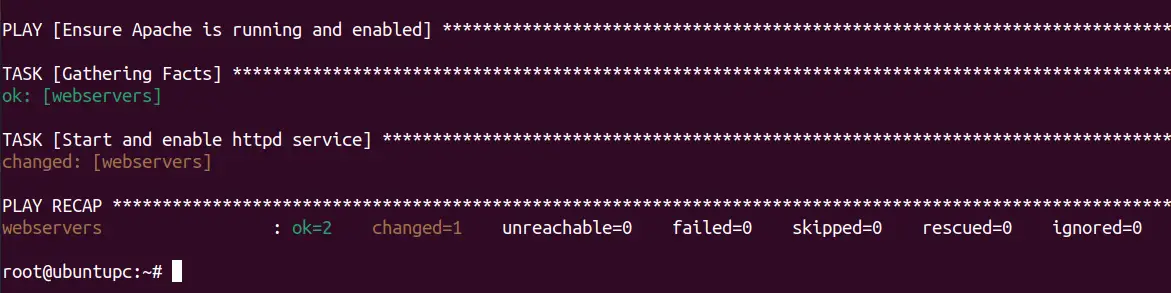
10 common Ansible modules and their usage
In this section, I'll show you some of the most commonly used Ansible modules and their usage.
1. ping
The ping module is used to test the connection to the target hosts. It is often used to ensure that the target hosts are reachable and responsive. This module is particularly useful for troubleshooting connectivity issues.
---
- name: Test connectivity
hosts: all
tasks:
- name: Ping all hosts
ping:
This Ansible playbook named Test connectivity
checks the network connectivity of all hosts in the inventory. It does so by running a single task:
sending a ping request to each host. The task, named Ping all hosts
uses the built-in ping
module to ensure that every host is reachable and responding to network requests.
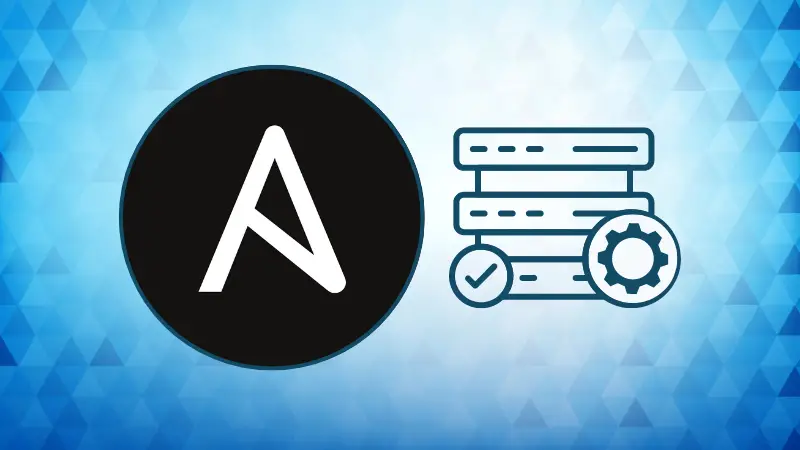
2. copy
The copy module copies files from the local machine to the remote host. It is used to transfer configuration files, scripts, or any other files that need to be present on the remote system. This module simplifies file distribution across multiple hosts.
---
- name: Copy a file to remote host
hosts: webservers
tasks:
- name: Copy index.html
copy:
src: /tmp/index.html
dest: /var/www/html/index.html
The above playbook targets hosts in the webservers
group and includes a single task. This task uses the copy
module to transfer a file named index.html
from the local source path /tmp/index.html
to the destination path /var/www/html/index.html
on each remote host.
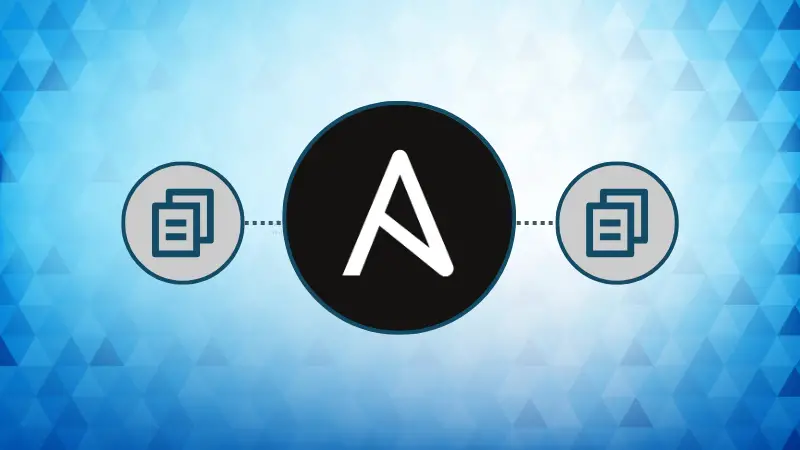
3. user
The user
module manages user accounts. It can create, delete, and manage the properties of user accounts on the remote system. This module is essential for ensuring that the correct users are present on the system with the appropriate permissions.
---
- name: Ensure a user exists
hosts: all
tasks:
- name: Create a user
user:
name: johndoe
state: present
groups: sudo
This playbook contains a single task, which uses the user
module to ensure that a user named johndoe
exists on each host. Additionally, it assigns this user to the sudo
group, granting administrative privileges.
4. package
The package
module is a generic way to manage packages across different package managers. It abstracts the differences between package managers like yum
, apt
, and dnf
, providing a consistent interface for package management tasks. This module helps streamline the installation and management of software packages.
---
- name: Install packages
hosts: all
tasks:
- name: Ensure curl is installed
package:
name: curl
state: present
The above playbook uses the package
module to ensure that the curl
package is installed on each host. The desired state of the curl
package is set to present
meaning it will be installed if it is not already available on the host.
5. shell
The shell
module is used to execute commands on the remote hosts. It allows for running shell commands with the full capabilities of the shell. They are useful for executing ad-hoc commands and scripts on remote systems.
---
- name: Run shell commands
hosts: all
tasks:
- name: Run a shell command
shell: echo "Hello, World!"
This playbook uses the shell module to execute the command echo "Hello, World!"
on each host. This command will output the text Hello, World!
in the shell of each remote host.
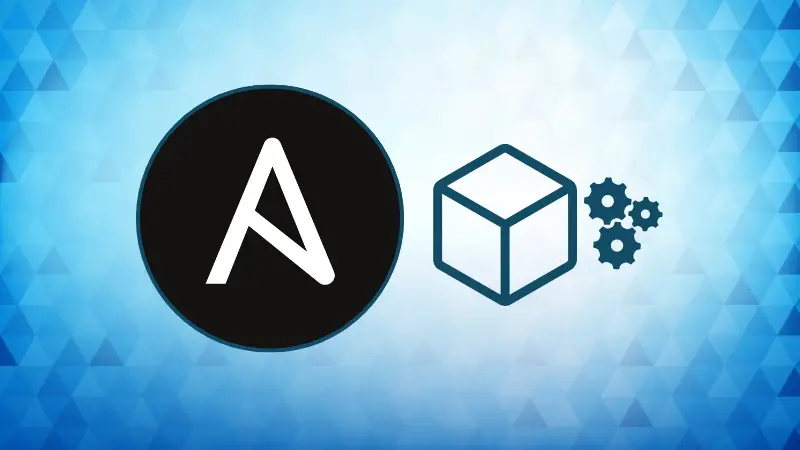
6. git
The git
module manages Git repositories. It can clone repositories, check out specific branches or commits, and update the repositories. This module is essential for deploying code and configuration managed in Git repositories.
---
- name: Clone a Git repository
hosts: all
tasks:
- name: Clone a repository
git:
repo: 'https://github.com/example/repo.git'
dest: /tmp
This playbook uses the git
module to clone the repository from https://github.com/example/repo.git
into the /tmp
directory on each host.
7. template
The template
module is used to copy and render Jinja2 templates. It allows for the dynamic creation of configuration files and scripts based on template files and variables. This module is crucial for creating customized and dynamic configuration files.
---
- name: Deploy a configuration file from template
hosts: all
tasks:
- name: Copy template file
template:
src: /tmp/template.j2
dest: /etc/nginx/nginx.conf
This playbook uses the template
module to deploy a configuration file by copying the template file located at /tmp/template.j2
on the control machine to /etc/nginx/nginx.conf
on each host. The template file can contain Jinja2 variables that are rendered with the appropriate values during the copy process.
8. file
The file
module manages file and directory properties. It can create, delete, and manage the properties of files and directories. This module ensures that the correct file system structure and permissions are in place.
---
- name: Ensure a directory exists
hosts: all
tasks:
- name: Create a directory
file:
path: /tmp/mydir
state: directory
The above playbook uses the file
module to ensure that a directory at the path /tmp/mydir
exists on each host. If the directory does not already exist, it will be created.
9. service
The service
module manages system services. It can start, stop, restart, and enable services on the remote system. This module is essential for ensuring that the necessary services are running and configured to start at boot.
---
- name: Ensure a service is running
hosts: all
tasks:
- name: Start a service
service:
name: nginx
state: started
This playbook uses the Ansible service module to ensure that the nginx service is running on each host. If the service is not already started, it will be initiated.
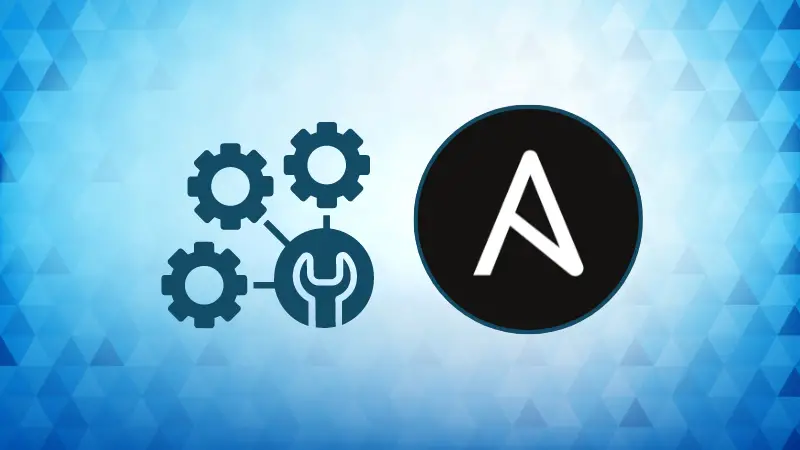
10. apt
The apt module manages packages using the apt package manager (for Debian-based systems). It handles package installation, removal, and updating. This module is vital for managing software on systems using the Debian package management system.
---
- name: Install a package using apt
hosts: all
tasks:
- name: Install nginx
apt:
name: nginx
state: present
This playbook uses the apt
module, which is specific to Debian-based systems like Ubuntu, to manage packages. It specifies that the package nginx should be installed, ensuring Nginx is present and available on all targeted hosts after the playbook is executed.
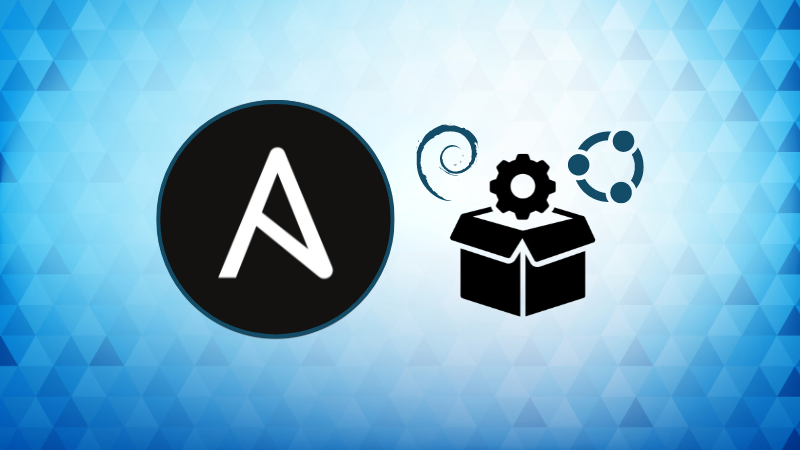
Conclusion
In this article, you explored the fundamental concept of Ansible modules, which are essential for automating tasks on remote hosts.
I showed the basic syntax for using Ansible modules and provided real-world examples of installing packages and managing services. Additionally, I listed and described common and popular Ansible modules, demonstrating their usage and importance in automating various system tasks.
To further enhance your skills, explore Ansible's extensive documentation and community resources to discover additional modules and advanced configurations.
LHB Community is made of readers like you who share their expertise by writing helpful tutorials. Contact us if you would like to contribute.