5 Practical Examples of the Read Command in Linux
With read command, you can make your bash script interactive by accepting user inputs. Learn to use the read command in Linux with these practical examples.

What is the read command in Linux?
The read command in Linux is a way for the users to interact with input taken from the keyboard, which you might see referred to as stdin (standard input) or other similar descriptions.
In other words, if you want that your bash script takes input from the user, you’ll have to use the read command.
I am going to write some simple bash scripts to show you the practical usage of the read command.
Read command examples
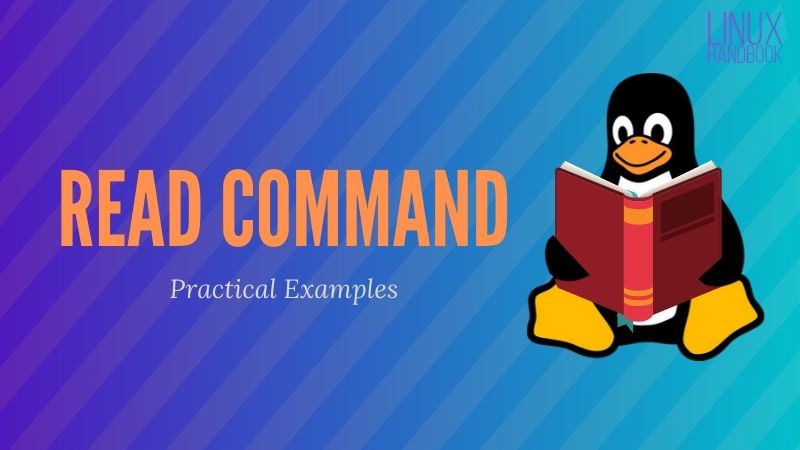
The read command can be confusing to get started with, especially for those who are new to shell scripting. The scripts I am going to use here are very simple to understand and should be easy to follow, especially if you are practicing along with the tutorial.
Basic Programming Concepts
With almost every program or script, you want to take information from a user (input) and tell the computer what to do with that information (output).
When you use read, you are communicating to the bash terminal that you want to capture the input from the user. By default, the command will create a variable to save that input to.
read [options] variable_name
Now let’s see some examples of read command to understand how you can use it in different situations.
1. Read command without options
When you type read without any additional options, you will need to hit enter to start the capture. The system will capture input until you hit enter again.
By default this information will be stored in a variable named $REPLY
.
To make things easier to follow for the first example, I will use the ↵
symbol will show when the enter key is pressed.
read ↵
hello world ↵
echo $REPLY ↵
hello world
More About Variables
As I mentioned earlier, the $REPLY
variable is built into read
, so you don’t have to declare it.
That might be fine if you have only one application in mind, but more than likely you’ll want to use your own variables. When you declare the variable with read, you don’t need to do anything other than type the name of the variable.
When you want to call the variable, you will use a $
in front of the name. Here’s an example where I create the variable Linux_Handbook
and assign it the value of the input.
You can use echo command to verify that the read command did its magic:
read Linux_Handbook ↵
for easy to follow Linux tutorials.
echo $Linux_Handbook ↵
for easy to follow Linux tutorials.
Reminder: Variable names are case-senstive.
2. Prompt option -p
If you’re writing a script and you want to capture user input, there is a read option to create a prompt that can simplify your code. Coding is all about efficiency, right?
Instead of using additional lines and echo commands, you can simply use the -p
option flag. The text you type in quotes will display as intended and the user will not need to hit enter to begin capturing input.
So instead of writing two lines of code like this:
echo "What is your desired username? "
read username
You can use the -p
option with read command like this:
read -p "What is your desired username? " username
The input will be saved to the variable $username.
3. “Secret”/Silent option -s
I wrote a simpe bash script to demonstrate the next flag. First take a look at the output.
bash secret.sh
What is your desired username? tuxy_boy
Your username will be tuxy_boy.
Please enter the password you would like to use:
You entered Pass123 for your password.
Masking what's entered does not obscure the data in anyway.
Here is the content of secret.sh
if you’d like to recreate it.
#!/bin/bash
read -p "What is your desired username? " username
echo "Your username will be" $username"."
read -s -p "Please enter the password you would like to use: " password
echo
echo "You entered" $password "for your password."
echo "Masking what's entered does not obscure the data in anyway."
As you can see, the -s
option masked the input when the password was entered. However, this is a superficial technique and doesn’t offer a real security.
4. Using a character limit with read option -n
You can add a constraint to the input and limit it to n number of characters in length.
Let’s use the same script from before but modify it so that inputs are limited to 5 characters.
read -n 5 -p "What is your desired username? " username
Simply add -n N
where N is the number of your choice.
I’ve done the same thing for our password.
bash secret.sh
What is your desired username? tuxy_Your username will be tuxy_.
Please enter the password you would like to use:
You entered boy for your password.
As you can see the program stopped collecting input after 5 characters for the username.
However, I could still write LESS than 5 characters as long as I hit ↵
after the input.
If you want to restrict that, you can use -N
(instead of -n) This modification makes it so that exactly 5 characters are required, neither less, nor more.
5. Storing information in an array -a
You can also use read command in Linux to create your own arrays. This means we can assign chunks of input to elements in an array. By default, the space key will separate elements.
christopher@pop-os:~$ read -a array
abc def 123 x y z
christopher@pop-os:~$ echo ${array[@]}
abc def 123 x y z
christopher@pop-os:~$ echo ${array[@]:0:3}
abc def 123
christopher@pop-os:~$ echo ${array[0]}
abc
christopher@pop-os:~$ echo ${array[5]}
z
If you’re new to arrays, or seeing how them in bash for the first time, I’ll break down what’s happening.
- Enter desired elements, separated by spaces.
- If we put only the @ variable, it will iterate and print the entire loop.
- The @ symbol represents the element number and with the colons after, we can tell iterate from index 0 to index 3 (as written here).
- Prints element at index 0.
- Similar to above, but demonstrates that the elements are seperated by the space
Bonus Tip: Adding a timeout function
You can also add a timeout to our read. If no input is captured in the allotted time, the program will move on or end.
christopher@pop-os:~$ read -t 3
christopher@pop-os:~$
It may not be obvious looking at the output, but the terminal waited three seconds before timing out and ending the read program.
Conclusion
I hope this tutorial was helpful in getting you started with the read command in Linux. As always, we love to hear from our readers about content they’re interested in. Leave a comment below and share your thoughts with us!
Christopher works as a Software Developer in Orlando, FL. He loves open source, Taco Bell, and a Chi-weenie named Max.