Check Prime Number
Here are a couple of bash scripts example to check whether a given number is prime or not.
Time to practice your Bash scripting.
Exercise
In this Bash practice exercise, write a shell script that accepts an integer and then checks whether the provided number is prime number or not.
💡Hint: A prime number is the one that is only divisible by itself.
Some sample prime numbers are:
2 3 5 7 11 13 17 19 23 ...
Remember that the zero and one are not prime number.
Test data
If you input 827, it should show:
827 is a prime number
If you input 21, you should get:
21 is not a prime number
Solution 1: Interactive bash script to check prime number
Here's a sample bash scripting for checking if a given number is prime or not.
Note that I used $num/2
even though it won't generate a floating point number (21/2 will result in 10, not 10.5) but it will still work the same as we don't need exact division here.
#!/bin/bash
# Zero and one are not prime number
read -p "Enter a number greater than 1: " num
if [ "$num" -lt 2 ]; then
echo "Number must be greater than 1"
exit
fi
# Set a flag
flag=0
# Loop to find if number is divisible by 2 or higher
# Loop until num/2 only as full loop is not required
# to check if num is divisible or not
# For example, 20 can only be divisible by 10, not 11
for ((i = 2; i <= $(($num/2)); ++i)); do
if [ $(($num % $i)) -eq 0 ]; then
flag=1
break
fi
done
if [ $flag -eq 0 ]; then
echo "$num is a prime number"
else
echo "$num is not a prime number"
fi
Solution 2: Non-interactive bash script to check prime number
The same bash script can be modified to use arguments supplied with the script (prime.sh N
) instead of using read command that makes it interactive.
#!/bin/bash
num=$1
if [ "$num" -lt 2 ]; then
echo "Number must be greater than 1"
exit
fi
# Set a flag
flag=0
# Loop to find if number is divisible by 2 or higher
# Loop until num/2 only as full loop is not required
# to check if num is divisible or not
# For example, 20 can only be divisible by 10, not 11
for ((i = 2; i <= $(($num/2)); ++i)); do
if [ $(($num % $i)) -eq 0 ]; then
flag=1
break
fi
done
if [ $flag -eq 0 ]; then
echo "$num is a prime number"
else
echo "$num is not a prime number"
fi
📖 Concepts to revise
The solutions discussed here use some terms, commands and concepts and if you are not familiar with them, you should learn more about them.
- read command
- arithmetic operation in bash
- if else in bash
- for loop in bash
📚 Further reading
If you are new to bash scripting, we have a streamlined tutorial series on Bash that you can use to learn it from scratch or use it to brush up the basics of bash shell scripting.
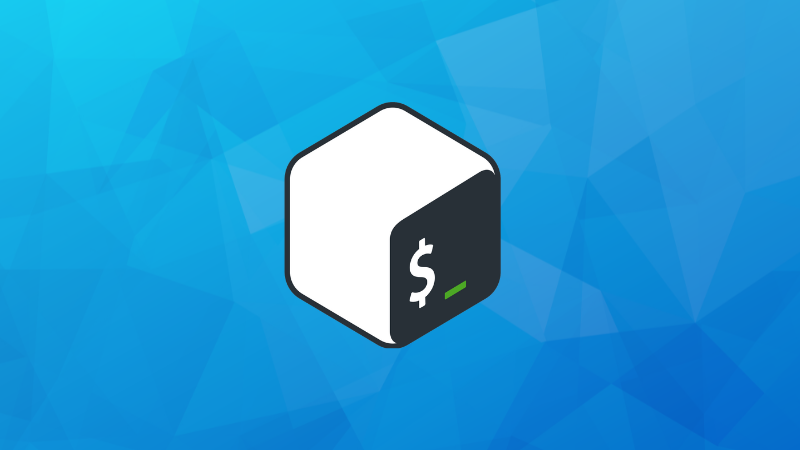
Creator of Linux Handbook and It's FOSS. An ardent Linux user & open source promoter. Huge fan of classic detective mysteries from Agatha Christie and Sherlock Holmes to Columbo & Ellery Queen.