Using exec Command in Bash Shell Scripts
The exec command in shell scripts is super useful for logging, reading from files and running commands by replacing the current process.

The shell built-in exec command is used for executing commands in shell scripts.
Wait! Don't shell scripts execute Linux commands already? They do. But exec runs the Linux commands without starting a new process. It replaces the shell process with the specified command.
Seems complex? Let me give you some examples of using the exec command in shell scripts:
- Process replacement
- Logging within the shell script
- Change standard input to read a file
- Change file descriptors
So let's start with the first one.
1. Use the exec command to replace process in shell script
Replacing the process is one of the most known implementations of exec in the shell script.
So here, I will be using a simple script that will display how the exec command in the script can be used to replace the current shell process.
First, use the following command to use the nano to create and edit a new script:
nano process_replacement.sh
And paste the following:
#!/bin/bash
echo "Before exec: This is the original script"
exec ls -l
echo "After exec: This line will not be executed"
Once done, save the changes and exit from the nano text editor.
Now, let me explain what this script will do.
Here are three command statements. First will print the basic text indicating the original script which is meant to be replaced.
The second statement involves the usage of the exec command with the ls command to list the contents of the current working directory and it will replace the previous process too!
Now, the third statement won't be executed as the process was replaced by the exec previously, and there were no additional arguments to support the execution of the third command statement.
Simply put, the process will be replaced by the 2nd command argument, and the 3rd command won't be executed.
And here's the output if you execute the shown script:
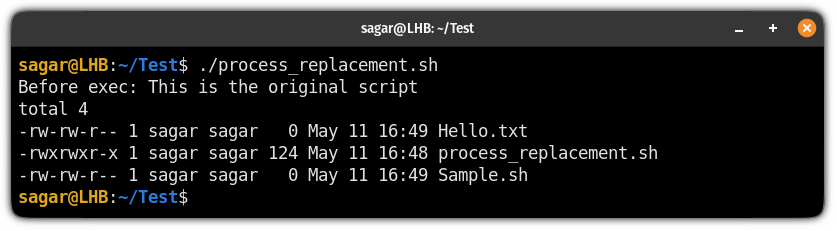
And as you can see, the 3rd command statement which was supposed to print "After exec: This line will not be executed" is shown here.
That does not seem very practical? Here's another example.
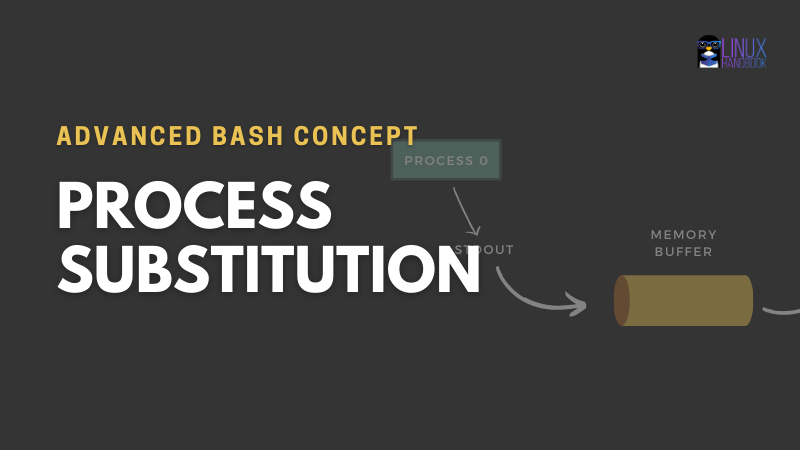
2. Use exec command in shell scripts for logging
Yet another interesting and easy implementation of the exec is where you can redirect the output to a file.
Here, I will be using 3 arguments, two for the standard output and one for standard error.
Here's a script:
#!/bin/bash
LOG_FILE="script.log"
# Redirect stdout and stderr to the log file
exec &> "$LOG_FILE"
# Start logging
echo "Script started at $(date)"
# Perform some operations
echo "Performing operation 1 (stdout)..."
ls -l /path/to/directory
echo "Performing operation 2 (stderr)..."
grep "search term" /path/to/nonexistentfile
echo "Performing operation 3 (stdout)..."
cat /path/to/file
# Log completion
echo "Script completed at $(date)"
I have created an empty file named script.log
in the same directory where the above script is located to store the logs.
Here, the exec command will redirect the output to the log file including when is started and ended.
When I ran the script, the file containing the log file looked like this:
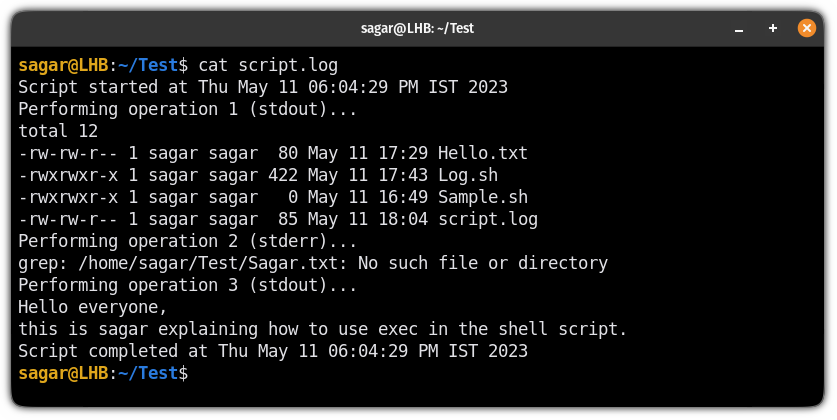
3. Change standard input to read files using exec
This can be very helpful when performing certain operations over the file.
Here, I will be using a simple text file named Hello.txt
that contains some random text lines:
Ubuntu, openSUSE, Arch, Debian, Fedora
+ - / *
2 4 6 1
4 6 1 2
And here's the script which will read from the file and output the contents to the standard output:
#!/bin/bash
INPUT_FILE="Hello.txt"
# Redirect stdin to read from a file
exec < "$INPUT_FILE"
# Read the entire file as a single input
content=$(cat)
# Process the input
echo "Read: $content"
Now, let me explain the script.
INPUT_FILE
specifies which file to read from.exec < "$INPUT_FILE"
is a redirection to read from the specified filecontent=$(cat)
will read the entire text file and assign it to thecontent
variable.echo "Read: $content"
will print the value of thecontent
variable.
And if you execute the script, the result will look like this:
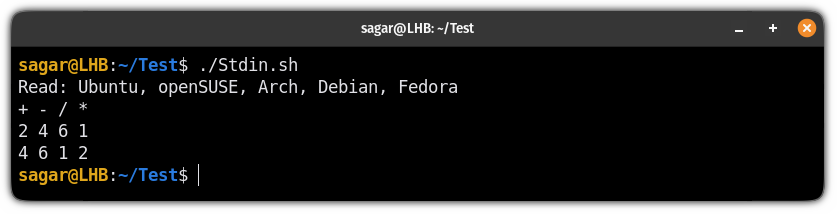
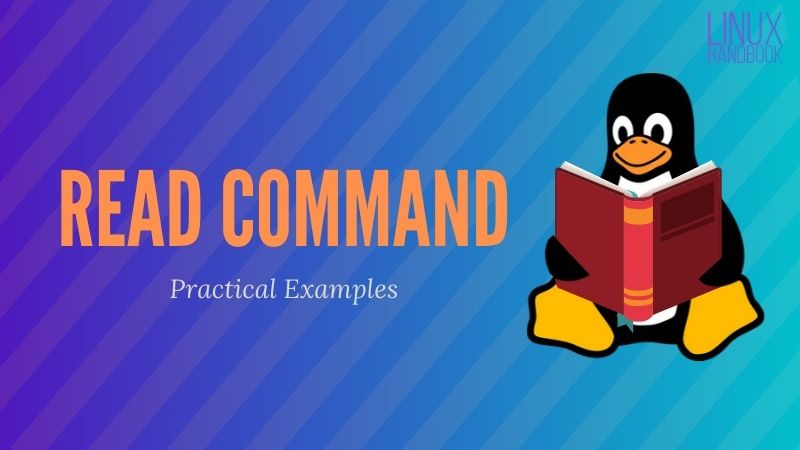
4. Change file descriptors using exec in the shell script (advanced)
There are three standard file descriptors in Linux:
- Standard input (stdin - file descriptor 0)
- Standard output (stdout - file descriptor 1)
- Standard error (stderr - file descriptor 2)
And using the exec command, you can change the descriptors. For example, you can use any number to use the preferred data stream, such as using 3 for stdin.
Let me share the script first and then explain how it functions:
#!/bin/bash
# Open a file for writing
exec 3> output.txt
# Redirect stdout to file descriptor 3
exec 1>&3
# Print some output
echo "This is a test message"
# Close the file descriptor
exec 3>&-
Here, I have opened a output.txt
file and assigned file descriptor 3 which means anything sent to file descriptor 3 will be written to the file.
Using exec 1>&3
, I have redirected the standard output (1) to the file descriptor 3 which means anything written to the standard output will be sent to the file descriptor 3 (to the output.txt in my case).
The echo statement prints the text to the standard output (which will be sent to the file as we changed the file descriptor earlier).
And exec 3>&-
kills the file descriptor 3 as it is no longer needed!
You can expect the following results after executing the above script:
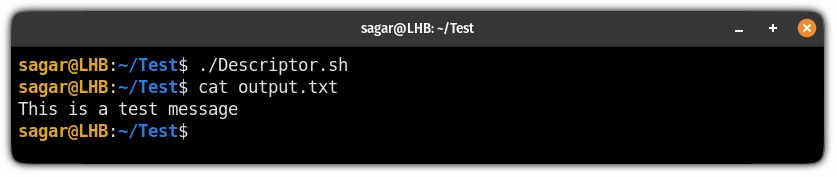
Do more by pairing exec with the find command
Did you know that you can use the find command with the exec and trust me, it makes a killer combination? It's not the same exec discussed here, though.
And if you want to learn how to, here's a detailed guide:
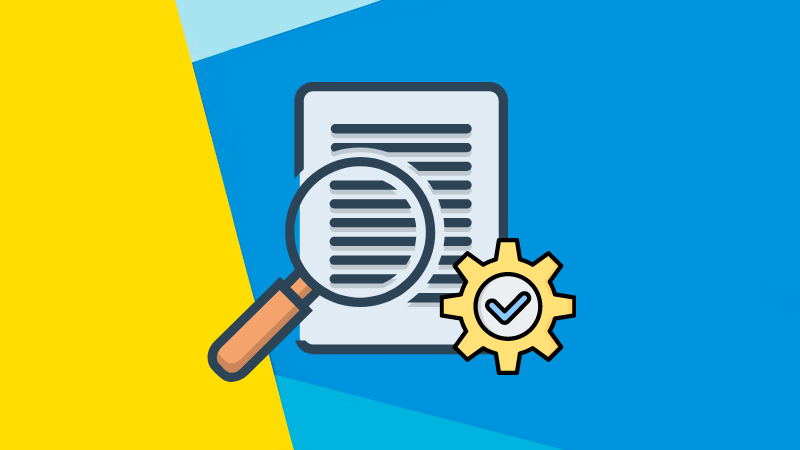
I hope you will find this guide helpful. And if you have doubts, leave a comment.
A software engineer who loves to tinker with hardware till it gets crashed. While reviving my crashed system, you can find me reading literature, manga, or watering my plants.