Use While Loop in Bash
Learn to use the while loop in bash with practical examples in this tutorial.

While running bash scripts, you'll come across times when you want to run tasks repeatedly such as printing the value of a variable in a specific pattern multiple times.
In this tutorial, I'm going to walk you through the following:
- The syntax of the while loop
- Multiple examples of the while loop
So let's start with the first one.
How to use the while loop in bash
Like any other loop, while loop does have a condition statement and the condition is the crucial factor to iterate the loop.
See, whenever you start the loop, you have to give a condition to the loop, which will only be iterated until the condition is valid.
Such as you have one variable a
having value 2. And you want to use the loop till the value is increased to 10.
So in that case, what you'll do is specify a condition where the value of the variable should be equal to 10
, and in the code block section, you can use the incremental function which will increase the value in each interaction.
And to use the while loop, you can refer to the following syntax:
while condition
do
#code-block
done
Let me break down the syntax for you.
condition
is a condition statement that will be checked every time before iterating the loop and if the condition is still true, then the loop will be iterated.do
indicated the starting point of the loop body.#code-block
is where you put the commands or code blocks which you want to execute until the specified condition is false.done
marks the end of the loop body.
Still confused? Let's have a look at one simple example.
Here's a simple loop that prints from 1 to 10:
#!/bin/bash
counter=1
while [ $counter -le 10 ];
do
echo $counter
counter=$((counter+1))
done
Let's break it down.
counter=1
: It is a variable namedcounter
that is initialized at 1.[ $counter -le 10 ]
: It is a condition that means the loop will be iterated as long as the value of thecounter
variable is less or equal to 10.echo $counter
: Prints the current value of thecounter
variable.counter=$((counter+1))
: Increments the value of thecounter
variable by 1.
When executed, you can expect the following result:
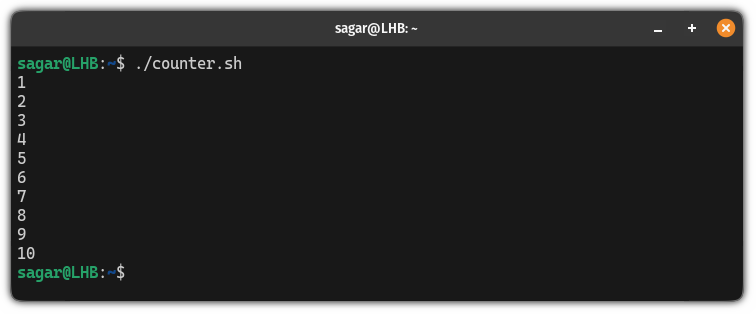
Want more such examples? Here you have it!
1. Create a timer using the while loop
Yep, you can create a timer using the while loop.
Let me share the code first and then, I will explain it:
#!/bin/bash
seconds=10
while [ $seconds -gt 0 ]; do
clear
echo "Timer: $seconds seconds"
sleep 1
seconds=$((seconds-1))
done
echo "Timer up!"
First, I initialized the value of the seconds
variable = 10.
[ $seconds -gt 0 ]
is a condition for a loop that checks whether theseconds
variable is greater than 0 or not. If yes, then, it will initiate the loop.clear
: A clear command is used to clear the terminal console.sleep 1
: Sleep for one second.seconds=$((seconds-1))
: Reduces the value of theseconds
variable by 1.echo
: Prints the given text or variable.
And if you were to execute the above script, you can expect the following result:
2. Read files line by line
There are times when you want to read the content of the file line by line and in that case, you can use the while loop as shown:
#!/bin/bash
filename="data.txt"
while IFS= read -r line
do
echo "$line"
done < "$filename"
Here, the filename
variable stores the name of the file which you want to read line by line.
IFS= read -r line
read the file line by line and store the data in theline
variable. Read more about IFS here.echo $line
prints the value of theline
variable.done
marks the end statements of the loop.< "$filename"
instructs loop to use the file from thefilename
variable.
data.txt
and to place it in the same directory as the script.When executed, you can expect the following results:
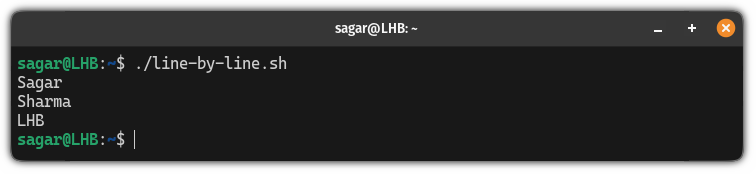
3. File line counter
There are times when you have a file having thousands of lines but what if you want to count the number of the lines? You can use the while loop in that case:
#!/bin/bash
filename="example.txt"
line_count=0
while IFS= read -r line; do
line_count=$((line_count + 1))
done < "$filename"
echo "Total lines: $line_count"
Here,
filename="example.txt"
means thefilename
variable stored the filename of the text file which you want to proceed for counting lines (example.txt in my case).line_count=0
: Theline_count
variable was initialized at 0.read -r line
reads each line from the file and stores it to theline
variable.line_count=$((line_count + 1))
: It will increase the value of theline_count
variable each time the loop iterates.< "$filename"
: It instructs the loop to use the file stored in the$filename
variable.
And if you were to use the same script, you can expect the following:
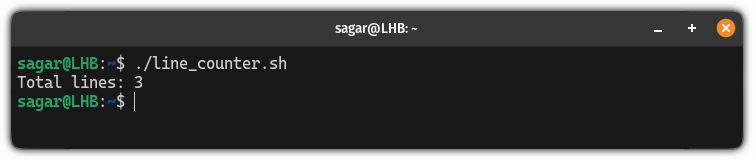
Like you, there are special variables too!
Did you know that there are special variables in bash?
Yep, there are 9 types of special variables in bash. Want to know what they are and how to use them? Here you go:
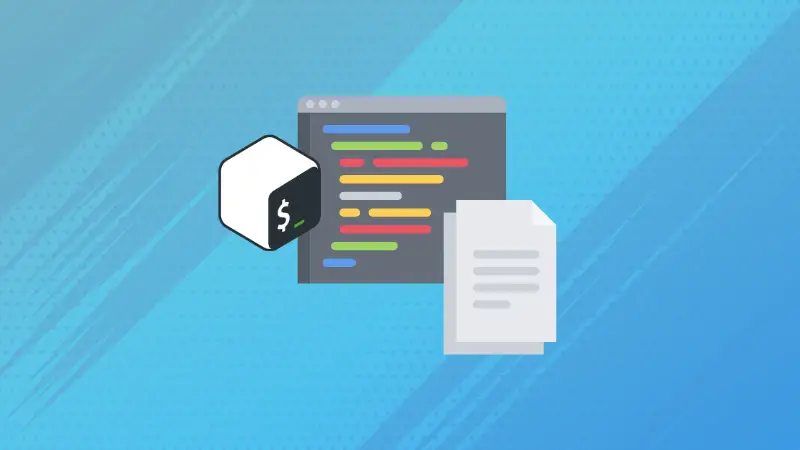
I hope you will find this guide helpful. And if you still have any doubts related to the while loop, feel free to ask in the comments.
A software engineer who loves to tinker with hardware till it gets crashed. While reviving my crashed system, you can find me reading literature, manga, or watering my plants.