Read File Line by Line in Bash
Here are a couple of ways for reading file line by line in the Bash shell.

You may find yourself in a situation where you want to use a shell script to read files line by line.
And in this tutorial, I will be covering multiple ways to read file line by line in the bash script.
Reading a file line by line in bash
In this tutorial, I will walk you through two ways to write a bash script by which you can read file line by line:
- Using read command with a while loop
- Using the cat command with the while loop
To make things easy to understand, I will be using a simple text file named LHB.txt
which contains the following:
1. Ubuntu
2. Arch
3. openSUSE
4. Fedora
5. Slackware
1. Using read command with while loop
As the method name suggests, here, I will be using the read command with the while loop inside the bash script.
First, create and open a simple script file using the following command:
nano read.sh
And paste the following lines (will explain in a moment):
#!/bin/bash
file="LHB.txt"
while read -r line; do
echo -e "$line\n"
done <$file
Here,
file="LHB.txt"
: Shows which file I want to work with and in my case, it's LHB.txt.while read -r line; do
: Starts while loop and reads lines one by one until there's no line to read and the-r
flag will prevent backslash escaping within the lines.echo -e "$line\n"
: Will print every line and each will be separated by one blank line.done < "$file"
: It redirects the input from the specified file to the while loop.
Once done, Save changes and exit from the nano text editor.
Make the script executable using the chmod command as shown:
chmod +x read.sh
And finally, execute the script:
./read.sh
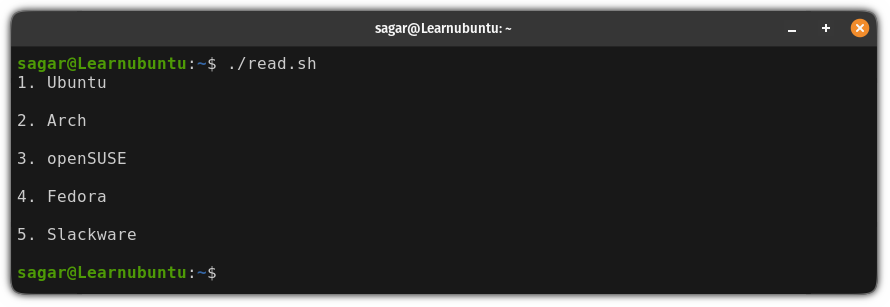
2. Using the cat command with the while loop
In this method, I will be using the cat command to read the file which will make the loop logic simple to understand.
This is the script I will be using:
#!/bin/bash
cat LHB.txt | while IFS= read -r line; do
echo "$line"
echo # Print a blank line
done
Here,
cat LHB.txt |
: Reads the file content of theLHB.txt
file and passes it to piped another argument.while IFS= read -r line; do
: It reads lines one by one of theLHB.txt
files and. IFS is used to preserve leading and trailing whitespace.echo "$line"
: Prints the line stored in theline
variable.
Once you execute the above command, you can expect the following results:
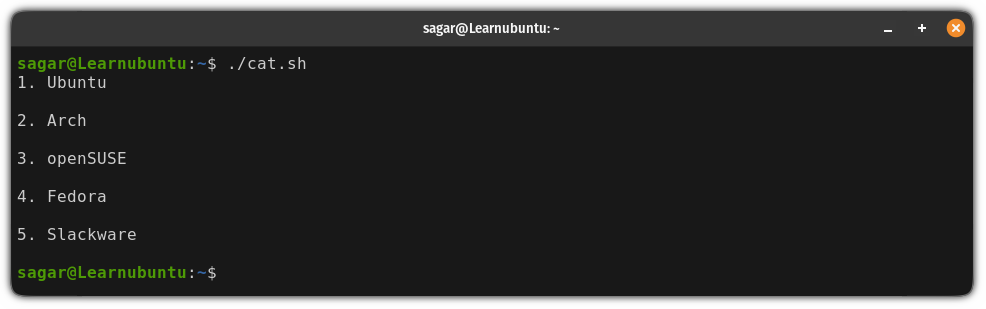
Learn bash for free
If you are just getting started or want to brush up on the basics, we have a dedicated series for you:
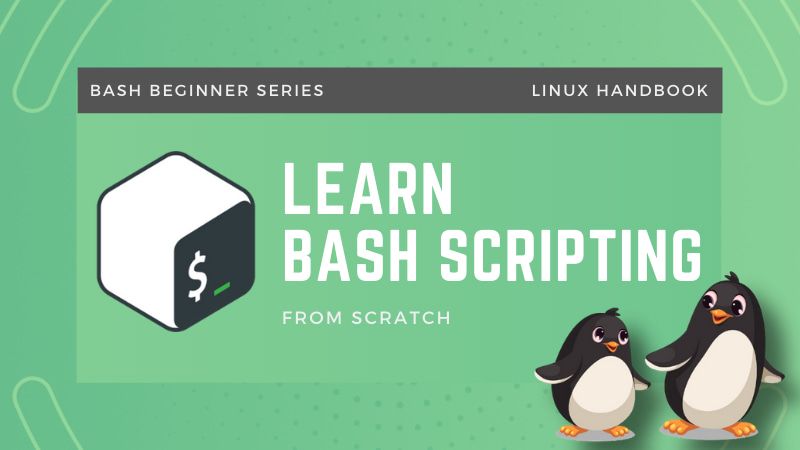
I hope you will find this guide helpful.
A software engineer who loves to tinker with hardware till it gets crashed. While reviving my crashed system, you can find me reading literature, manga, or watering my plants.