Using test Command in Bash Scripts
Learn to use the test command in bash for testing conditions and making comparisons.
β Team LHB

The bash test command is used to check the validity of an expression. It checks whether a command or an expression is true or false. Also, it can be used to check the type and permissions of a file.
If the command or expression is valid, the test
command returns a 0
else it returns 1
.
Using the test command
The test command has a basic syntax like this:
test βvar1β operator βvar2β
When using variables in the test command, use double quotes with the variable's name.
Let's use the test
command to check whether 10 equals 20 and 10 equals 10:
$ test 10 -eq 20 && echo "true" || echo "false"
In the above example:
test
- test command10
- the first variable-eq
- operator for comparison20
- second variable
If the given expression is valid, the first command is executed, or else the second command is executed.
π‘Instead of using the test
command keyword, you can also use the brackets []
. But remember, there is space between the [
mark and the variables to compare:
[ 10 -eq 20 ] && echo "true" || echo "false"

Not only integers; you can also compare strings in bash with the test command. Let me share some examples.
String comparison with test Command
Here are some string comparison examples using the test command.
Check if the string is not empty
The -n
flag checks if the string length is non-zero. It returns true if the string is non-empty, else it returns false if the string is empty:
$ [ -n "sam" ] && echo "True" || echo "False"

Check if the string is empty
The -z
flag checks whether the string length is zero. If the string length is zero, it returns true, else it returns false:
$ [ -z "sam" ] && echo "True" || echo "False"
Check if strings are equals
The '=' operator checks if string1 equals string2. If the two strings are equal, then it returns 0; if the two strings are not equal, then it returns 1:
$ [ "sam" = "SAM" ] && echo $? || echo $?
In this case, the expression is slightly different, instead of typing true or false, the stdout
variable is printed using $?
.
Check if strings are not equals
The !=
operator checks if String1 is not equal to String2. If the two strings are not equal, then it returns 0. If two strings are equal, then it returns 1:
[ "abc" != "ABC" ] && echo $? || echo $?
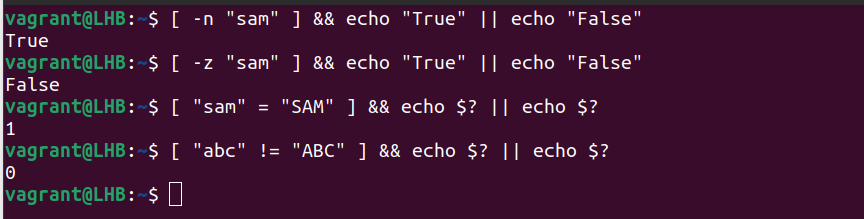
Integer comparison with test command
Let's make some number comparisons with bash test.
Check if numbers are equal
The -eq
operator checks if Integer1 equals Integer2. If integer1 equals integer2, then it returns 0, else it returns 1:
[ 10 -eq 20 ] && echo $? || echo $?
Check if the numbers are not equal
Case 2. The -ne
operator checks if integer1 is not equal to integer2. If Integer1 is not equal to integer2, then it returns 0, else it returns 1:
[ 10 -ne 20 ] && echo $? || echo $?
Check if a number is equal or greater than the other
The -ge
operator checks that integer1 is greater than or equal to integer2. If integer 1 is greater than or equal to integer 2 it returns 0; if not, then it returns 1.
[ 100 -ge 10 ] && echo $? || echo $?
The -gt
operator checks if integer1 is greater than integer2. If yes, then it returns 0. Otherwise, it returns 1:
[ 20 -gt 10 ] && echo $? || echo $?
Check if a number is equal or less than the other
The -le
operator checks if the integer1 is less than or equal to integer2. If true it returns 0, else it returns 1:
[ 5 -le 10 ] && echo $? || echo $?
The -lt
operator checks if integer1 is less than integer2. If integer 1 is less than integer2, then it returns 0, else it returns 1:
[ 5 -lt 10 ] && echo $? || echo $?
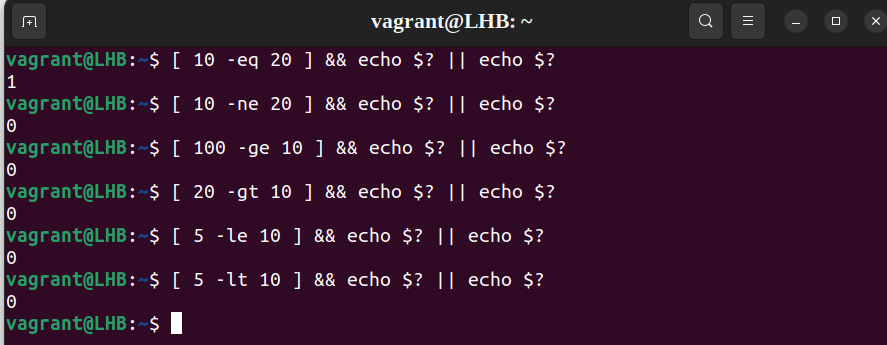
File and directory Operations with test
The test command can be used with files and directories.
This checks whether a file is executable (by the current user) or not. If a file is executable, then it returns 0, else it returns 1:
[ test -x filename ] && echo executable || echo non-executable
You can use other file permissions like r and w in the same fashion. Other common parameters you can use are:
| Command | Description |
|---------|--------------------------|
| -e | File/directory exists |
| -f | is a file |
| -d | is a directory |
| -s | File size greater than 0 |
| -L | is a link |
| -S | is a socket |
Using test command in bash scripts
You have seen the one-liners of the test command so far. You can also use the test condition with the if-else condition in the bash scripts.
Let me share a simple example of comparing two numbers passed as arguments to the shell script:
#!/bin/bash
## Check if the numbers are equal or not
read -p "Enter the first number: " num1
read -p "Enter the second number: " num2
if test "$num1" -eq "$num2"
then
echo "$num1 is equal to $num2"
else
echo "$num1 is not equal to $num2"
fi
You can run the bash script with various numbers:
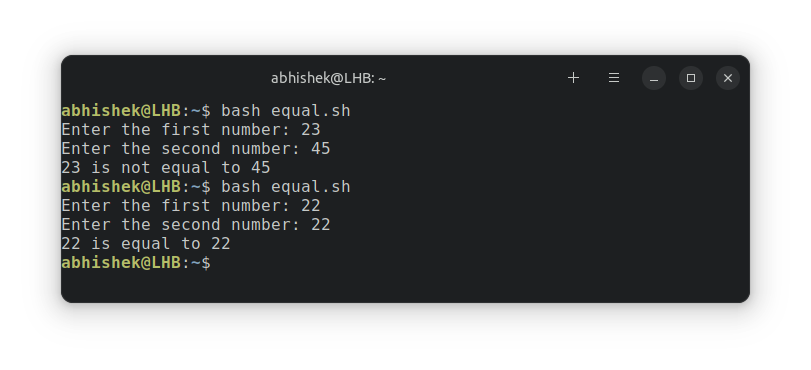
I hope this tutorial helps you to understand the basics of the test command in the bash.
Team LHB indicates the effort of a single or multiple members of the core Linux Handbook team.