Various Ways to Increment or Decrement Counters in Bash
Working with loops in bash? Here are various ways of incrementing and decrementing counters.

Incrementing/decrementing is mostly used in a loop where the user has instructed the script/program to increase/decrease value based on the given condition.
And bash allows you to use various ways to increment or decrement the value.
So in this tutorial, I will explain three ways to do so:
- Using
+
and-
operators - Using
+=
and-=
operators - Using
++
and--
operators
Each works the same so you may choose the best possible method for your workflow.
How to increment or decrement variable in bash
Incrementing and decrementing are arithmetic operations that are performed using double parentheses ((...))
and $((...))
or you can also use the built-in let
command.
I know it sounds too complex so I will be sharing syntax for both so you can choose the best for you.
1. Using + and - operators
In my opinion, this is the most straightforward way to increment and decrement the variable in bash.
So let's start with the syntax. If you want to use double parentheses, here's how you do it.
For increment:
((i=i+1))
For decrement:
((i=i-1))
Using the let command
If you want to use the let command instead of double parentheses, here's the syntax to do so.
For increment:
let "i=i+1"
For decrement:
let "i=i-1"
Example of using + and - operators
In this section, I will be sharing examples of both incrementing and decrementing a variable.
Here, I will be using double parentheses syntax for the increment example whereas let command for decrement.
Incrementing a variable:
In this example, I have used an until loop which will increment the value of the variable till gets 3 and print the value at each iteration:
i=1
until [ $i -gt 3 ]
do
echo i: $i
((i=i+1))
done
And the output should look like this:
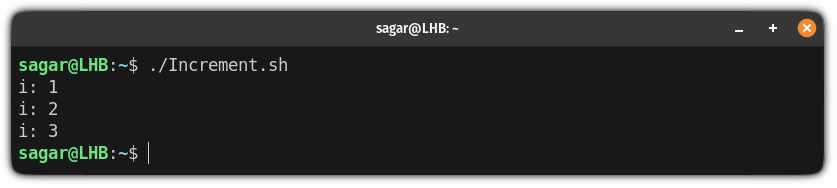
Decrementing a variable:
Here, I will be using the let command instead of the double parentheses so you can have a rough idea of how you use both.
In this example, I have used them until the loop which will iterate till the value of the i
variable gets 5 or less and till then, the value of i
will be decremented by two in each variable:
i=20
until [ $i -le 5 ]
do
echo i: $i
let "i=i-2"
done
And here's the output:
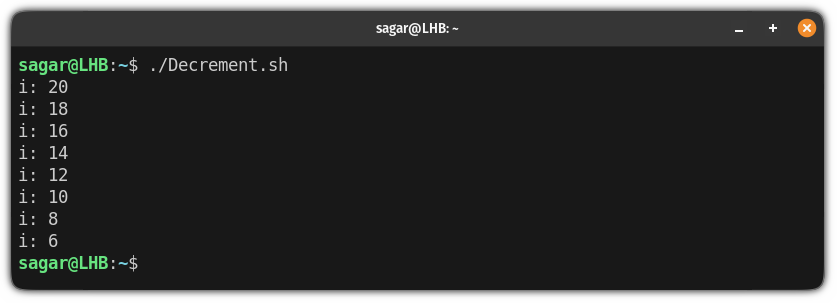
2. Using += and -= operators
So if you want to use +=
and -=
operators to increment and decrement the values of a variable, in this section, I will show you syntax and examples for both.
Let's start with the syntax for the double parentheses.
For increment:
((i+=1))
For decrement:
((i-=1))
Using the let command
If you prefer to use the let instead of the double parentheses, here's the syntax for both increment and decrement:
For increment:
let "i+=1"
For decrement:
let "i-=1"
Now, let's jump to the examples part.
Examples of using += and -= operators
In this section, I will be sharing an example of how you can use the +=
and -=
operators.
Also, I will be using double parentheses for the decrement and let command for the increment example.
Incrementing a variable:
In this example, I have used a while loop which will iterate will the value of the i
the variable is less or equal to 5:
i=1
while [ $i -lt 5 ]
do
echo i: $i
let "i+=1"
done
And here's the output:
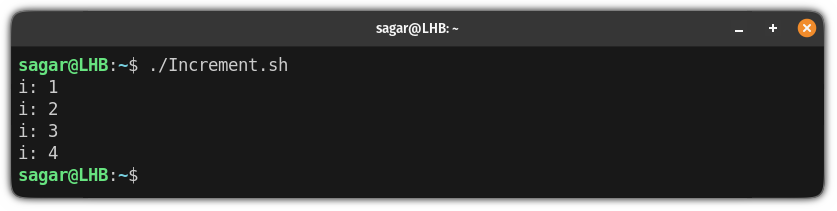
Decrementing a variable:
In the example of decrement, I have used the while loop which will reduce 5 and run till the value is less than 2:
i=20
while [ $i -ge 2 ]
do
echo i: $i
((i-=5))
done
Here's the output after running the loop as a script:
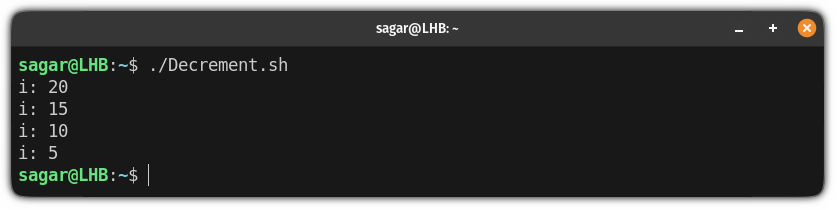
3. Using ++ and -- operators
You can use the operators in prefix and postfix manner so let's have a look at the difference between them:
- Prefix: If you use the operator as a prefix, like
++var
, the value of the variable will be increased and then it returns the value. - Postfix: If you use the operator as a postfix, like
var++
, the value of the variable will be returned first and then incremented.
So let's start with how these operators can be evaluated in double parentheses syntax.
For increment:
((i++)) #For postfix
((++i)) #For prefix
For decrement:
((i--)) #For postfix
((--i)) #For prefix
For let command syntax
If you're someone like me who prefers using the let instead of double parenthesis, here's the syntax for increment and decrement.
For increment:
let "i++" #For postfix
let "++i" #For prefix
For decrement:
let "i--" #For postfix
let "--i" #For prefix
Now, let's jump to the examples part.
Examples of using ++ and -- operators
Here, I will be using the double parentheses syntax for the increment and let command syntax for the decrement example.
Incrementing a variable:
Here, I will be using until the loop which will increase the value till the value reaches 5:
i=1
until [ $i -gt 5 ]
do
echo i: $i
((i++))
done
And here's the output:
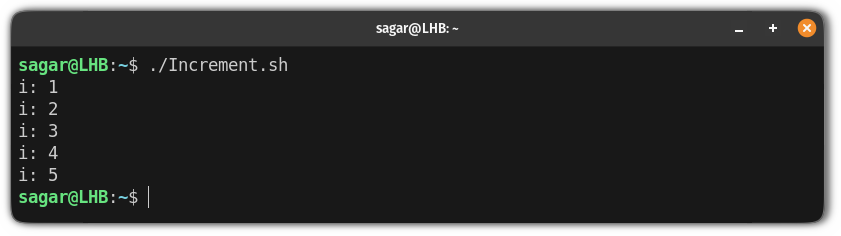
Decrementing a variable:
Here, I will be using the let command with the while loop which will iterate till the value of the variable becomes 5:
i=10
while [ $i -ge 5 ]
do
echo i: $i
let "--i"
done
And here's the output:
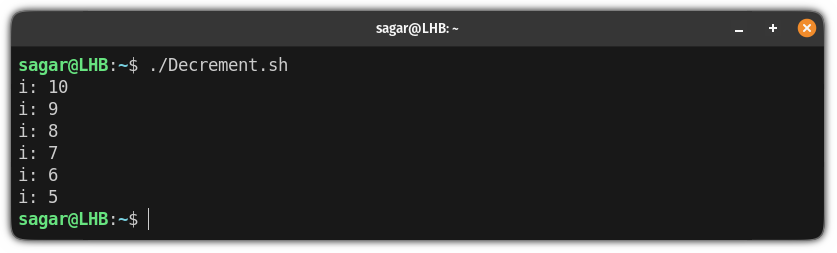
Want to learn bash from the scratch?
If you want to learn the bash scripting from scratch, we have a dedicated series of articles that will clear all the basics and give you a strong foundation for advancement:
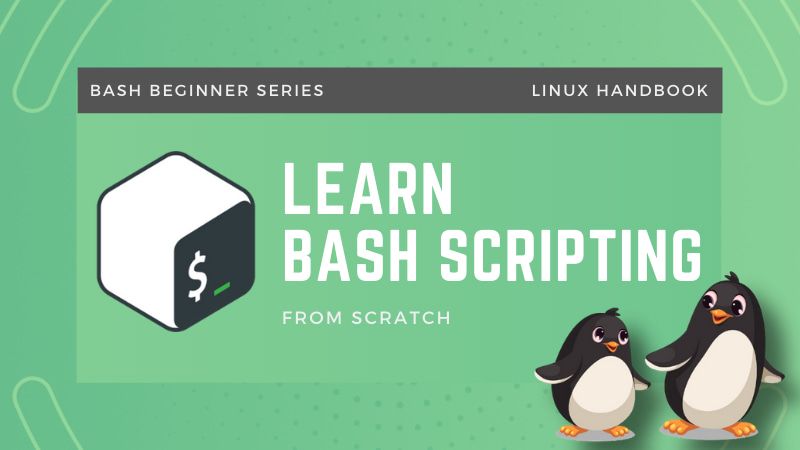
I hope you will find this guide helpful. And if you have any doubts, feel free to ask in the comments.
A software engineer who loves to tinker with hardware till it gets crashed. While reviving my crashed system, you can find me reading literature, manga, or watering my plants.