How to Evaluate Strings as Numbers in Bash
Learn how to evaluate bash strings as integers to perform successful arithmetic operations.
Like everything is file in Linux, everything is string in bash.
Yes! Technically, there are no data types in Bash. Essentially, Bash variables are just character strings.
And that creates a problem when you are trying to do arithmetic operations in bash. The numbers you try to add give you weird results.
avimanyu@linuxhandbook:~$ sum=3+6
avimanyu@linuxhandbook:~$ echo $sum
3+6
To convert strings to integers in bash, wrap them in $((X)). Like this:
$((string))
If the string has an identifiable number, it will be treated as a number. The example I showed above can be corrected as:
avimanyu@linuxhandbook:~$ sum=$((3+6))
avimanyu@linuxhandbook:~$ echo $sum
9
Let's see the conversion of strings into numbers in a bit more detail so that you can use arithmetic calculations in bash.
Converting string variables to numbers
Let's declare some "numbers" which are basically strings of characters.
avimanyu@linuxhandbook:~$ a=11
avimanyu@linuxhandbook:~$ b=3
You can check the assigned number:
avimanyu@linuxhandbook:~$ echo $a
11
vimanyu@linuxhandbook:~$ echo $b
3
Let's now try to add both numbers and store the value in a third variable c
:
avimanyu@linuxhandbook:~$ c=$a+$b
avimanyu@linuxhandbook:~$ echo $c
11+3
As you can see on the output above, c is also being treated as a string.
To make the addition successful, you need to “convert” it through an arithmetic expansion:
avimanyu@linuxhandbook:~$ c=$(($a+$b))
avimanyu@linuxhandbook:~$ echo $c
14
Do note that c
is still actually a string, until you implicitly use the same arithmetic expansion again for another operation that I'll discuss in a while.
avimanyu@linuxhandbook:~$ c=$((5))
The above is equivalent to:
avimanyu@linuxhandbook:~$ c=5
Let's use a third variable d
for another operation:
avimanyu@linuxhandbook:~$ d=10
All these implicit declarations are still strings. It is same as how I had defined a
and b
earlier.
Now for the second operation:
avimanyu@linuxhandbook:~$ e=$(($a+$b*$c-$d))
avimanyu@linuxhandbook:~$ echo $e
16
In the above expression, the product of b and c is calculated first and finally the addition and subtraction follow.
As I mentioned previously, anything you do between $((...)) is considered to be an arithmetic operation.
Try mixing actual strings and numbers
Mixing strings and integers is still safer than mixing coke and mint. It does produce a weird result, though.
avimanyu@linuxhandbook:~$ sum=$((3+hello))
avimanyu@linuxhandbook:~$ echo $sum
3
It will only work when there are numbers (as string). If it is pure character string, it won't be magically converted into some numbers. Instead, it is ignored as you can see in the example above.
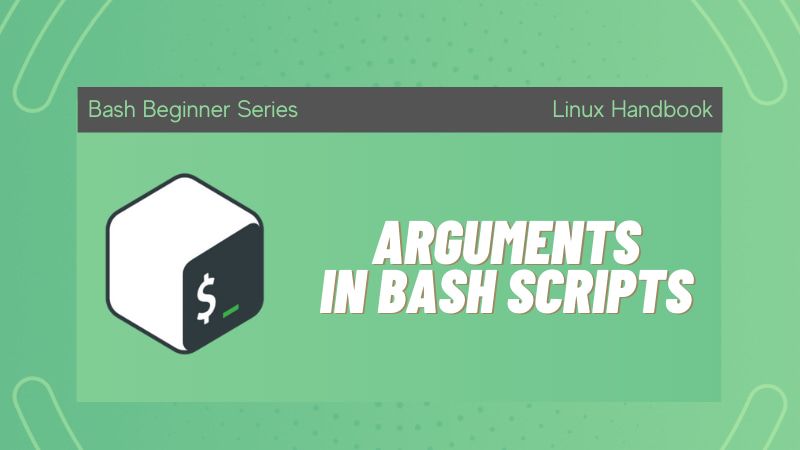
Alternate method: use expr
You can also use the expr
tool to do the evaluation, but do note that it is not a “native” Bash procedure, as you need to have coreutils
installed (by default on Ubuntu) as a separate package.
avimanyu@linuxhandbook:~$ expr $a + $b \* $c - $d
16
I hope this quick little tutorial helped you in evaluating bash strings as numbers. If you have questions or suggestions, feel free to leave a comment below.
DevOps Geek at Linux Handbook | Doctoral Researcher on GPU-based Bioinformatics & author of 'Hands-On GPU Computing with Python' | Strong Believer in the role of Linux & Decentralization in Science