Deleting lines with sed command is quite simple if you understand the trick.
And the trick is that it follows this pattern mostly:
sed 'nd' filename
Think of it as "delete n".
I know that doesn't give much information but it will be more relatable when you see the sed command examples for deleting lines I am going to share in this article.
I'll be using this sample file called lines.txt
in the examples. It will be easier to follow with this text content.
Line number 1
Line number 2
Line number 3
Line number 4
Line number 5
Line number 6
Line number 7
Line number 8
Line number 9
Line number 10
Delete the first line
This is simple. Just use 1d
like this:
sed '1d' filename
And here's an example of the command in action:
abhishek@LHB:~$ sed '1d' lines.txt
Line number 2
Line number 3
Line number 4
Line number 5
Line number 6
Line number 7
Line number 8
Line number 9
Line number 10
Delete a specific line number
To delete the nth line of a file, just provide the number in this manner:
sed 'nd' filename
Let's say I delete the 4th line in my example here:
abhishek@LHB:~$ sed '4d' lines.txt
Line number 1
Line number 2
Line number 3
Line number 5
Line number 6
Line number 7
Line number 8
Line number 9
Line number 10
If the number exceeds the total lines in the file, no line is deleted.
Delete the last line
In the previous examples. you specified the line number. Deleting the first line was easier as you used 1d
but you won't always know the last line number. You can get that info for sure but there is a much easier way to remove the last line with sed:
sed '$d' filename
Here, $
represents the last line and d
is for delete (and you know that already).
abhishek@LHB:~$ sed '$d' lines.txt
Line number 1
Line number 2
Line number 3
Line number 4
Line number 5
Line number 6
Line number 7
Line number 8
Line number 9
Delete range of lines
If you want to delete a specific range of lines from the text file, that can be easily done with sed like this.
To remove lines starting from line number x and going till line number y, use sed command like this:
sed 'x,yd' filename
In the example below, the command removes the line from 4 to 6:
abhishek@LHB:~$ sed '4,6d' lines.txt
Line number 1
Line number 2
Line number 3
Line number 7
Line number 8
Line number 9
Line number 10
Needless to say that smaller number should be used first and bigger later.
Delete selective lines
Not everything can be grouped into range. What if you want to delete a selected few lines? You can use their line numbers in this manner:
sed 'xd,yd,zd' filename
Let's say, I want to delete lines 5,7 and 9:
abhishek@LHB:~$ sed '5d;7d;9d' lines.txt
Line number 1
Line number 2
Line number 3
Line number 4
Line number 6
Line number 8
Line number 10
Delete all lines except specified lines
Sed command also supports negation and you can use it to remove all lines except the Nth line or the given range of lines.
To delete all lines except 1st, use:
sed '1!d' filename
To delete all lines except last, use:
sed '$!d' filename
To delete all lines except 5th line, use:
sed '5!d' filename
To delete all lines except lines from 4 to 6, use:
sed '4,6!d' filename
Delete all empty lines
To delete all blank lines, use the sed command in this manner:
sed '/^$/d' filename
Keep in mind that if a line only contains single space, it won't be deleted with the above command.
Delete all lines starting or ending with a certain letter
You can bring simple regex in the play and use it to delete lines based on pattern.
For example, to delete all lines start with letter L, use:
sed '/^L/d' filename
Yes, it is case sensitive.
Similarly, you can delete all lines that end with a certain character/word:
sed '/L$/d' filename
Delete all lines containing a word or pattern
Actually, the above few examples could be grouped in this one because all of them are deleting lines that follow a pattern.
sed '/pattern/d' filename
In our example, let's delete lines containing Line number 1
:
abhishek@LHB:~$ sed '/Line number 1/d' lines.txt
Line number 2
Line number 3
Line number 4
Line number 5
Line number 6
Line number 7
Line number 8
Line number 9
The possibilities are endless. Once you understand the pattern, it becomes easy.
If you are new to SED, I recommend this guide to get started with it.
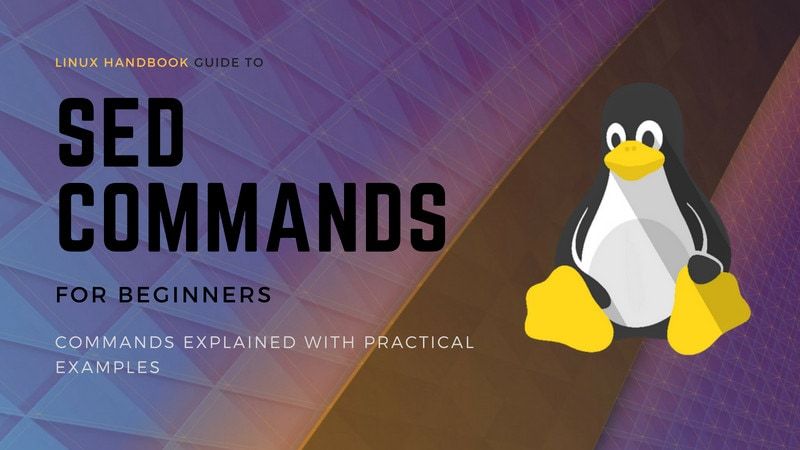
Creator of Linux Handbook and It's FOSS. An ardent Linux user & open source promoter. Huge fan of classic detective mysteries from Agatha Christie and Sherlock Holmes to Columbo & Ellery Queen.