Run Python Scripts in Linux Command Line
This quick tip shows how to run Python programs from the Linux command line.

If you want to do something, it is pretty much guaranteed that there is a Python script for it. So, when you have a Python script, how do you run it?
The simplest way is to use the python
or python3
command in the following manner:
python <file-name>.py
If you see the python command not found error, try python3:
python3 <file-name>.py
python
and have an explicit command python3
for python3. So if you want Python 3 to be guaranteed, use python3
.Method 1: Run it using python
The easiest method to run a Python script on any Linux distribution is by invoking the python
command and provide it with the name of your Python script.
The syntax is as below:
python3 <script-name>.py
This will ensure that if the file's contents are valid, it will be executed without any problems.
Method 2: Make Python script executable
You may have encountered executing bash scripts simply by typing out their relative/absolute path in the terminal. i.e. you never had to do a bash <script-name>.sh
like you do with Python scripts by typing python <script-name>.py
In order to achieve this, a few things should be done first.
If you might have noticed, the bash scripts that you can execute by simply typing out their relative/absolute path, they are "executable" files.
To make your Python script executable, run the following command in your terminal:
chmod +x <script-name>.py
This should be it. Right? I have a file hello.py
, let's try running it.
$ cat hello.py
print("Hello Linux Handbook!")
$ chmod +x hello.py
$ ./hello.py
./hello.py: 1: Syntax error: word unexpected (expecting ")")
Uh oh... What? Syntax error? But it is a valid Python program/script.
The reason why we got this error is because by making hello.py
an executable file, all we did was tell the shell that "You can execute it", but not what interpreter/program to use when it is being executed.
My shell (bash) thought that it was a bash script. And hence, we got this error.
The solution to overcome this is pretty simple. Add the following line to the first line of your Python script.
#!/usr/bin/env python
The #!
syntax is used mostly in scripts (where you need an interpreter). Now, the /usr/bin/env
part means that we are calling env
to find the python
command from $PATH
and execute it for us.
This essentially starts Python for us, instead of the user needing to invoke it manually every time. And, if you don't automate these things, are you even scripting?
Another reason to use env
in shebang is to make it portable. Some systems have the Python executable interpreter stored as /usr/local/bin/python
, while some might have /usr/bin/python
.
Now, let's try executing hello.py
once again, but with the necessary changes made to it.
$ sed -i '1 i\#!/usr/bin/env python\n' hello.py
$ cat hello.py
#!/usr/bin/env python
print("Hello Linux Handbook!")
$ ./hello.py
Hello Linux Handbook!
Oh hey! It runs flawlessly now.
Now that you know how to run Python programs from the terminal, how about learning to use Linux commands from Python scripts?
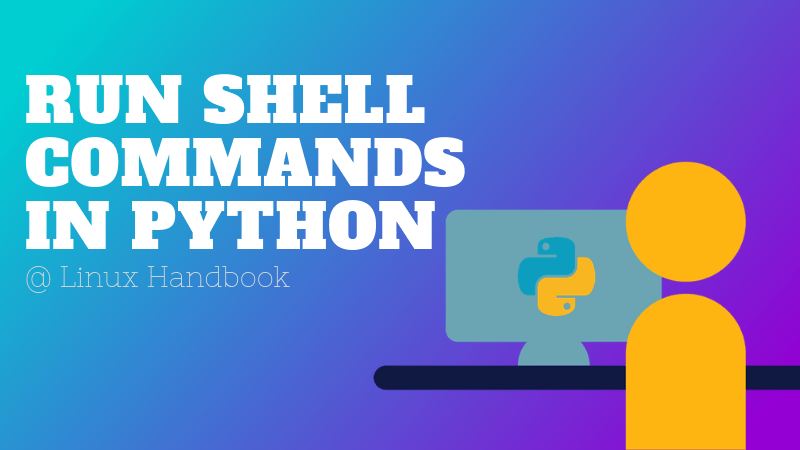
Navigating through the world of Rust, RISC-V, Podman. Learning by doing it and sharing by writing it.