Add Multiline Comments in Python
Python does not support multiline comments by default. However, you can use the docstring feature to achieve that.

When you are new to Python and trying to figure your way around, you may wonder how to add comments in Python.
That's not too difficult. Just put a hash #
sign and write the comment text after it in a single line. That's the single line comment.
The problem comes with multiline comments. Python does not support multiline comments. At least not directly.
There are two ways to achieve multiline comment in Python:
- Use # at the beginning of each line of the code block you want to comment (achievable with the keyboard shortcut of your code editor or IDE).
- Use docstrings (
"""
) to comment out a block of code
Most likely, you are interested in the latter part, so I'll discuss that in detail.
Add multiline comments in Python using docstrings """
Just like what you do in Bash for multiline comment, you'll have to resort to a trickery here as well.
Python has the concept of document strings (docstrings in short). It is used in the beginning of the function, modules and classes to describe their use. The same text is used in the automatically generated documentation.
You can use this feature to achieve multiline commenting in Python by adding a block of codes/text between two """ (3 double inverted commas).
Look at the example code below, it also showcases some basic use cases of both comments:-
def multiply_numbers(num1, num2):
""" This is a Multi-Line Comment used for describing the function
This function multiplies two numbers and returns the result.
Arguments:
num1: The first number.
num2: The second number.
Returns:
The product of num1 and num2.
"""
product = num1 * num2 # Store the product of num1 & num2 in product
return product
# Example usage:
num1 = 5
num2 = 3
result = multiply_numbers(num1, num2) # Calling the function
print(result) # Output: 15
Remember! Docstrings are not comments
Docstrings are used for automatically generating text for documentation. They give the description of modules, functions and classes.
Unlike the single comments (#), they are not ignored by the Python interpreter and can be accessed by __doc__
attribute.
In fact, in the previous example, you can print the docstrings like this:
print(multiply_numbers.__doc__)
Where multiply_numbers
is the function from where you are getting this doc string.
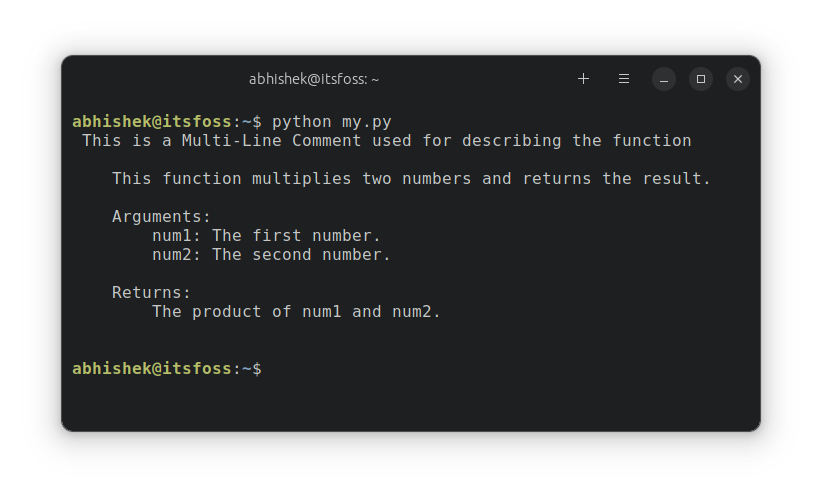
If interested, you can check the official Python documentation on Docstring.
Commenting as a good developer habit
If you keep the readability and ease of understanding of the code for other developers in mind, you will develop a really good habit that will be helpful in the future in a developer job, open source or even for yourself to understand your own code.
I mean be honest, who remembers why we wrote that big complex function, and how it works, or an even bigger question, why it works 😜
Thanks for reading, I hope you got something to learn from the article, keep developing!