Python Basics: How To Print in Python?
See how to print something in python. Also learn how to avoid some common mistakes while printing in Python.

It’s quite common to make mistakes when you try to print something using Python considering you’re new to Python scripting.
No matter what program you write, you will always need to print something or the other (most of the time).
So, in this article, I’ll be explaining how to print something in Python and list out some common mistakes that you can avoid.
How to print in Python
Basically, this is how you call the print function in Python:
print()
Inside the parenthesis, you will have to pass the arguments (values that you normally want to pass through a function or method).
For instance, if you want to display a text, you need to pass a string value. Here’s how it looks like:
print ("I am a programmer")
As you can see, you will have to pass the string values using quotes (single or double usually).
It’s best to use double quotes because you might end up using single quotes within the string, so to avoid confusion and error, double quote should be preferred.
As per the technical documentation, the syntax for using print function is:
print(*objects, sep=' ', end='\n', file=sys.stdout, flush=True)
Here, the possible parameters/arguments are:
- *Objects – You can have one more objects
- Sep=’ ‘ – Refers to a separator parameter
- end=’\n’ – Refers to the end parameter
- file=sys.stdout – A file in write mode that prints the string inside it
- flush=True – Helps you to clean the internal buffer
Well, that’s about how you print something in Python – easy, right?
However, you will have to be a little bit more careful when passing different types of arguments (or objects). I’ll list some examples below for you to know more about it.
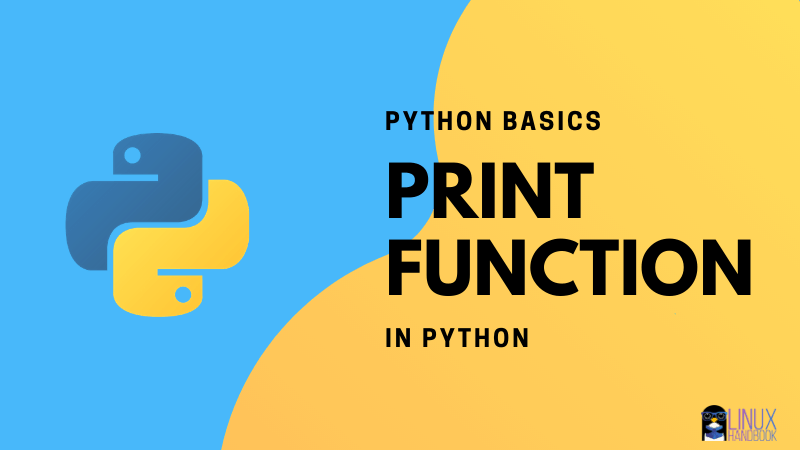
1. Passing objects with print function
Let’s take a simple example:
a = 2
print ("The value of a is", a)
Output for this will be:
The value of a is 2
Similarly, you can expand the same program with another variable and print it using the print function as follows:
a = 2
print ("The value of a is", a)
b = a
print ("a =",b,"= b")
The output for this will be:
The value of a is 2
a = 2 = b
Here, in the above example, you can notice that when you separate the objects you pass in the print function using the , (comma) – that automatically adds a space in the output, also known as the softspace feature.
But, what if you do not want the space between them or want to format the output – what else can you do?
Fret not, you can use the separator and the end parameter to achieve that. Let’s take a look at it below.
2. Print using the separator in Python
The separator (sep=’separator’) argument in Python helps you to format your output and potentially remove the softspace feature as mentioned above.
Here’s what you have to do:
a = 2
print ("The value of a is",a,sep='')
So, when you add sep=’ ‘ as another argument in the print function, it disables the softspace feature and the output will look like this:
The value of a is2
As you can observe, there’s no space after the string and number 2.
Separators could come in handy to print dates as well, here’s how can do that:
print('12','02','2020', sep='-')
The output for this will be:
12-02-2020
Useful, right? Similarly, you can utilize the separator parameter to format the output further. Now, in addition to the separator, you can also use the end parameter to print the next output in a new line.
3. Print using separator & end parameter
It is worth noting that the end parameter helps to specify what to print at the end. In other words, it introduces a new line for the next output.
Here’s a sample code snippet for that:
print('12','02','2020', sep='-', end='\n\n\n')
print("Hi!")
As you can notice, I’ve added three new lines at the end of the first output. So, the output looks like:
12-02-2020
Hi!
Let me try something else instead of adding a three new lines. Take a look:
print('12','02','2020', sep='-', end='\nto the future')
print("Hi!")
The output for this will be:
12-02-2020
to the futureHi!
As you can observe, I’ve added a new line after the date gets printed and then the next output continues in the same line.
4. Print with file parameter
If you know about the File I/O operations, you can easily pass on a file parameter with print function as follows:
print("This is a sample string", file = sourceFile)
You can also add the flush=True argument to the above code before closing the file – in case you want it. But, it isn’t necessary.
Wrapping Up
You can explore more about its usage and variations by checking out some of my recommended free Python resources.
Did I miss anything here? Or, did you explore something interesting to use the print function in Python? Let me know in the comments below.
A passionate technophile who also happens to be a Computer Science graduate. He has had bylines at a variety of publications that include Ubergizmo & Tech Cocktail. You will usually see cats danc