gcc vs g++: What's the Difference?
gcc and g++ compilers work quite similar when it comes to compiling C programs in Linux. Here's how they are different from each other.

Have you ever thought about the difference between GCC and G++? Well, if I were to answer this in one sentence, it would be "GCC is used to compile C programs whereas G++ is for C++".
But there's a lot more to talk about, such as how you install and use them.
So let's start with the basic introduction.
What is the difference between GCC and G++?
The GCC compiler is used to compile the C programs, whereas the G++ is used for C++ programs. While you could still use GCC to compile C++ program (by using it in gcc -xc++ -lstdc++ -shared-libgcc
format, g++ provides a simpler syntax.
Actually, GCC (GNU Compiler Collection) is a super set of various compilers. It has g++ for C++ and GNAT for Ada programming language.
While gcc is capable of compiling C++ programs with help of special flags, you should use g++ instead. The g++ compiler links to standard C++ libraries by default. GCC can also do that but not by default.
Now, let's have a look at the installation.
Installation
Being one of the most essential tools for compiling C and C++ programs in Linux, they both are available in the repositories of all Linux distributions.
Here's how you install it on various Linux distributions:
For Ubuntu/Debian base:
sudo apt install gcc g++
For Fedora/RHEL:
sudo dnf instal gcc g++
For Arch Linux:
sudo pacman -S gcc
(Arch includes G++ in the GCC package itself)
Usage
In this section, I will walk you through how you can compile C and C++ programs using GCC and G++.
To make this guide accessible, I will be using the following C programming code:
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
And here's the C++ code:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
They both should print a simple Hello, World!
line.
Compile and run C code
To compile a C program, you can use gcc or g++. If you want to use gcc, here's the syntax that you need to follow:
gcc -o my_program my_program.c
Here,
-o
is used to specify the output filemy_program
is where you specify the name of the output filemy_program.c
is where you append the name of the input file
For example, here's how I compiled my hello.c
file using the GCC compiler:
gcc -o hello hello.c
To execute any compiled file, add ./
before the name of the compiled file:
./hello

Similarly, if you want to use G++ to compile the C program, use the following:
g++ -o cpp_program cpp_program.c
Here's how I compiled and ran the same hello.c
program with G++:

The &&
operator is used to run multiple commands but the compilation code will be executed first and then the execution will be done.
Compile and run C++ code
To compile C++ code, you have to use the G++ compiler in the following manner:
g++ -o my_code my_code.cpp
Once done, you can run the code using the executable file:
./my_code
For example, here's how I compiled hello.cpp
file using the G++ compiler:
g++ -o hello hello.cpp && ./hello

Next: Here are some important GCC flags
If you are just getting started with the C programming, you are about to use GCC a lot but there are multiple flags that you can use while compiling code.
And here's a detailed guide on important GCC flags:
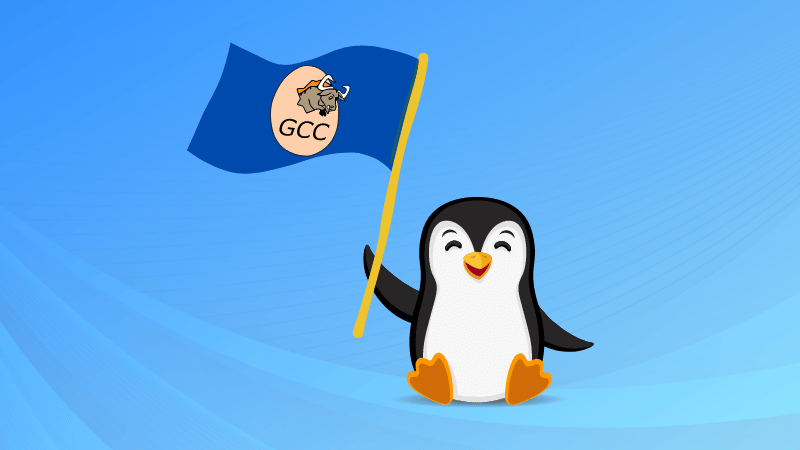
I hope you will find this guide helpful.
A software engineer who loves to tinker with hardware till it gets crashed. While reviving my crashed system, you can find me reading literature, manga, or watering my plants.