Docker Interview Questions: From Beginners to Advanced
Quickly revise the Docker concepts before your job interview with this set of more than 50 questions and their answers.
— Team LHB

After years of training DevOps students and taking interviews for various positions, I have compiled this list of Docker interview ques tions (with answers) that are generally asked in the technical round.
I have categorized them into various levels:
- Entry level (very basic Docker questions)
- Mid-level (slightly deep in Docker)
- Senior-level (advanced level Docker knowledge)
- Common for all (generic Docker stuff for all)
- Practice Dockerfile examples with optimization challenge (you should love this)
If you are absolutely new to Docker, I highly recommend our Docker course for beginners.
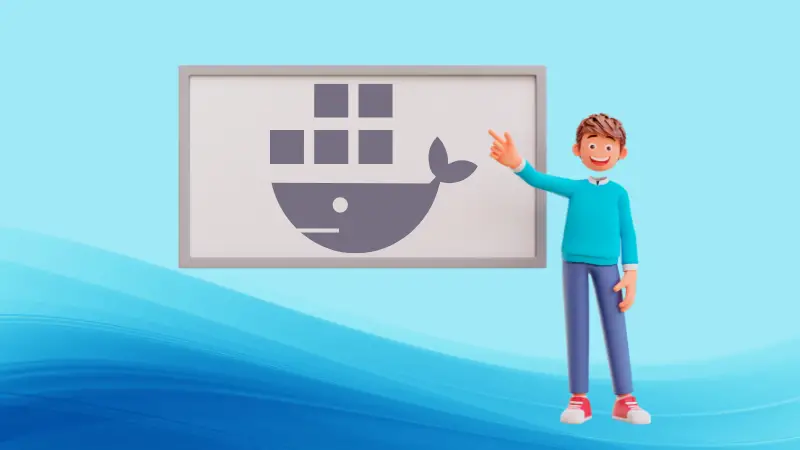
Let's go.
Entry level Docker questions
What is Docker?
Docker is a containerization platform that allows you to package an application and its dependencies into a container. Unlike virtualization, Docker containers share the host OS kernel, making them more lightweight and efficient.
What is Containerization?
It’s a way to package software in a format that can run isolated on a shared OS.
What are Containers?
Containers are packages which contains application with all its needs such as libraries and dependencies
What is Docker image?
- Docker image is a read-only template that contains a set of instructions for creating a container that can run on the Docker platform.
- It provides a convenient way to package up applications and preconfigured server environments, which you can use for your own private use or share publicly with other Docker users.
What is Docker Compose?
It is a tool for defining and running multi-container Docker applications.
What’s the difference between virtualization and containerization?
Virtualization abstracts the entire machine with separate VMs, while containerization abstracts the application with lightweight containers sharing the host OS.
Describe a Docker container’s lifecycle
Create | Run | Pause | Unpause | Start | Stop | Restart | Kill | Destroy
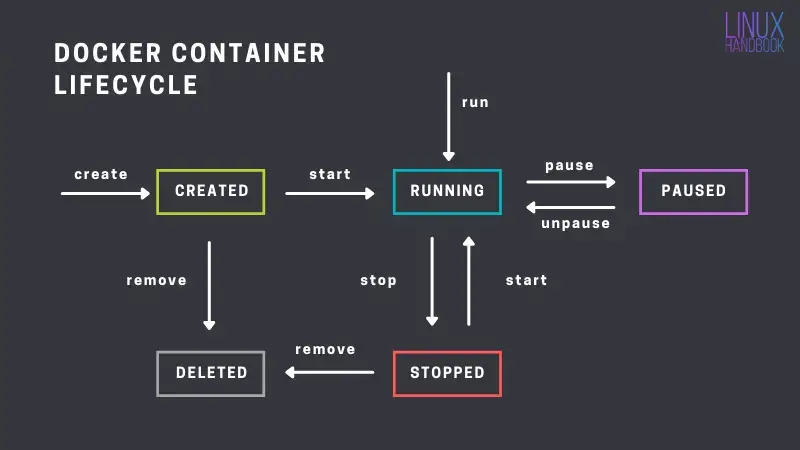
What is a volume in Docker, and which command do you use to create it?
- A volume in Docker is a persistent storage mechanism that allows data to be stored and accessed independently of the container's lifecycle.
- Volumes enable you to share data between containers or persist data even after a container is stopped or removed.
docker volume create <volume_name>
TO KNOW : example :- docker run -v data_volume:/var/lib/mysql mysql
What is Docker Swarm?
Docker Swarm is a tool for clustering & managing containers across multiple hosts.
How do you remove unused data in Docker?
Use docker system prune
to remove unused data, including stopped containers, unused networks, and dangling images.
Mid-level Docker Questions
What command retrieves detailed information about a Docker container?
Use docker inspect <container_id>
to get detailed JSON information about a specific Docker container.
How do the Docker Daemon and Docker Client interact?
The Docker Client communicates with the Docker Daemon through a REST API over a Unix socket or TCP/IP
How can you set CPU and memory limits for a Docker container?
Use docker run --memory="512m" --cpus="1.5" <image>
to set memory and CPU limits.
Can a Docker container be configured to restart automatically?
Yes, a Docker container can be configured to restart automatically using restart policies such as --restart always or --restart unless-stopped.
What methods can you use to debug issues in a Docker container?
- Inspect logs with
docker logs <container_id>
to view output and error messages. - Execute commands interactively using
docker exec -it <container_id> /bin/bash
to access the container's shell. - Check container status and configuration with
docker inspect <container_id>
. -
Monitor resource usage with
docker stats
to view real-time performance metrics. - Use Docker's built-in debugging tools and third-party monitoring solutions for deeper analysis.
What is the purpose of Docker Secrets?
Docker Secrets securely manage sensitive data like passwords for Docker services. Use docker secret create <secret_name> <file>
to add secrets.
What are the different types of networks in Docker, and how do they differ?
Docker provides several types of networks to manage how containers communicate with each other and with external systems.
Here are the main types:
- Bridge
- None
- Host
- Overlay Network
- Macvlan Network
- IPvlan Network
bridge: This is the default network mode. Each container connected to a bridge network gets its own IP address and can communicate with other containers on the same bridge network using this IP
docker run ubuntu
Useful for scenarios where you want isolated containers to communicate through a shared internal network.
none: Containers attached to the none network are not connected to any network. They don't have any network interfaces except the loopback interface (lo).
docker run ubuntu --network=none
Useful when you want to create a container with no external network access for security reasons.
host: The container shares the network stack of the Docker host, which means it has direct access to the host's network interfaces. There's no isolation between the container and the host network.
docker run ubuntu --network=host
Useful when you need the highest possible network performance, or when you need the container to use a service on the host system.
Overlay Network : Overlay networks connect multiple Docker daemons together, enabling swarm services to communicate with each other. It's used in Docker Swarm mode for multi-host networking.
docker network create -d overlay my_overlay_network
Useful for distributed applications that span multiple hosts in a Docker Swarm.
Macvlan Network : Macvlan networks allow you to assign a MAC address to a container, making it appear as a physical device on the network. The container can communicate directly with the physical network using its own IP address.
docker network create -d macvlan --subnet=192.168.1.0/24 --gateway=192.168.1.1 -o parent=eth0 my_macvlan_network
Useful when you need containers to appear as physical devices on the network and need full control over the network configuration.
IPvlan Network: Similar to Macvlan, but uses different methods to route packets. It's more lightweight and provides better performance by leveraging the Linux kernel's built-in network functionalities.
docker network create -d ipvlan --subnet=192.168.1.0/24 --gateway=192.168.1.1 -o parent=eth0 my_ipvlan_network
Useful for scenarios where you need low-latency, high-throughput networking with minimal overhead.
Explain the main components of Docker architecture
Docker consists of the Docker Host, Docker Daemon, Docker Client, and Docker Registry.
- The Docker Host is the computer (or server) where Docker is installed and running. It's like the home for Docker containers, where they live and run.
- The Docker Daemon is a background service that manages Docker containers on the Docker Host. It's like the manager of the Docker Host, responsible for creating, running, and monitoring containers based on instructions it receives.
- The Docker Client communicates with the Docker Daemon, which manages containers.
- The Docker Registry stores and distributes Docker images.
How does a Docker container differ from an image?
A Docker image is a static, read-only blueprint, while a container is a running instance of that image. Containers are dynamic and can be modified or deleted without affecting the original image.
Explain the purpose of a Dockerfile.
Dockerfile is a script containing instructions to build a Docker image. It specifies the base image, sets up the environment, installs dependencies, and defines how the application should run.
How do you link containers in Docker?
Docker provides network options to enable communication between containers. Docker Compose can also be used to define and manage multi-container applications.
How can you secure a Docker container?
Container security involves using official base images, minimizing the number of running processes, implementing least privilege principles, regularly updating images, and utilizing Docker Security Scanning tools. ex. Docker vulnerability scanning.
Difference between ARG & ENV?
- ARG is for build-time variables, and its scope is limited to the build process.
- ENV is for environment variables, and its scope extends to both the build process and the running container.
Difference between RUN, ENTRYPOINT & CMD?
- RUN : Executes a command during the image build process, creating a new image layer.
- ENTRYPOINT : Defines a fixed command that always runs when the container starts. Note : using --entrypoint we can overridden at runtime.
- CMD : Specifies a default command or arguments that can be overridden at runtime.
Difference between COPY & ADD?
- If you are just copying local files, it's often better to use COPY for simplicity.
- Use ADD when you need additional features like extracting compressed archives or pulling resources from URLs.
How do you drop the MAC_ADMIN capability when running a Docker container?
Use the --cap-drop flag with the docker run command:
docker run --cap-drop MAC_ADMIN ubuntu
How do you add the NET_BIND_SERVICE capability when running a Docker container?
Use the --cap-drop flag with the docker run command:
docker run --cap-add NET_BIND_SERVICE ubuntu
How do you run a Docker container with all privileges enabled?
Use the --privileged flag with the docker run command:
docker run --privileged ubuntu
Team LHB indicates the effort of a single or multiple members of the core Linux Handbook team.