Restart a Single Container with Docker Compose
Restarting single container within a multi-container Docker Compose application helps during development or troubleshooting.

The docker-compose restart
command is designed to restart one or more containers defined in your docker-compose.yml
file.
By default, if you run docker-compose restart
without specifying any container names, it will restart all the containers in your application. You may not always want that.
During development, you may want to quickly restart a container to test code changes without waiting for the entire application stack to restart.
Similarly, there are situations when a specific container is causing performance issues and restarting it without restarting others can help you isolate the source of the problem.
The good thing is that docker compose allows you to restart specific containers and I am going to discuss this scenario in this tutorial.
Restarting a specific container with Docker Compose
To restart a single container, you simply append the name of the container to the end of the command:
docker-compose restart <container_name>
Replace <container_name>
with the actual name of the container you want to restart. This name is usually the same as the service name you defined in your docker-compose.yml
file.
Let's say you have a web application with the following docker-compose.yml
file:
services:
web:
image: nginx:latest
ports:
- "8000:8000"
db:
image: postgres:latest
cache:
image: redis:latest
In this setup, you have three containers: web
, db
, and cache
(Redis). If you want to restart only the web
container, you will run:
docker-compose restart web
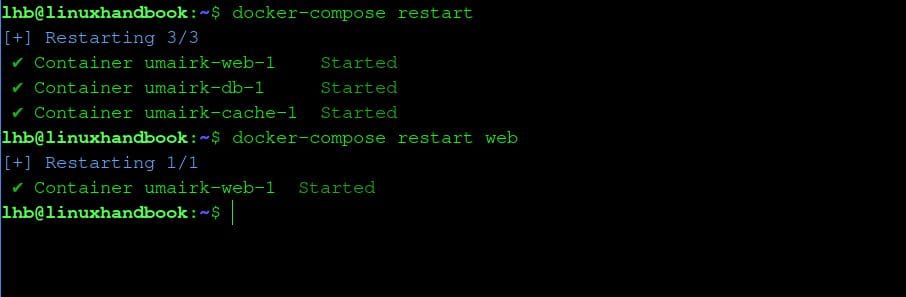
Restarting individual containers and dependency management
Imagine you have a web application that relies on a database. If you restart the web
container using docker-compose restart web
, the db
container won't be automatically restarted, even though the web
container depends on it.
This happens because Docker Compose focuses on managing the lifecycle of individual containers.
It doesn't inherently understand the runtime dependencies between them. So, if you need to restart a container and its dependencies, you can:
Restart them individually:
docker-compose restart web && docker-compose restart db
Or, restart them together:
docker-compose restart web db
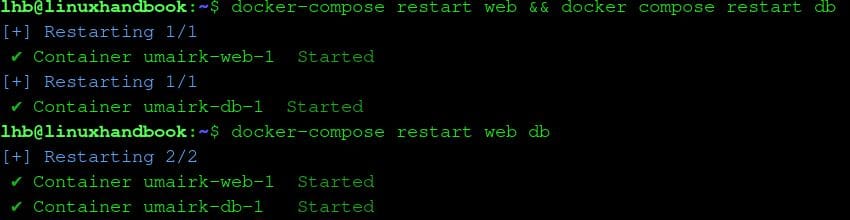
💡 You may also use docker-compose up
command which recreates all containers, ensuring dependencies are also restarted. But if you are recreating a container, you'll lose any data that is not stored in Docker Volumes.
In your docker-compose.yml
file, you should define a volume and mount it to the appropriate directory within the container.
volumes:
- ./postgres-data:/var/lib/postgresql/data
Restart specific container without restarting dependencies
In some cases, you might want to restart/recreate a container without affecting any of its dependencies.
You can achieve this using the docker-compose up
command with the --no-deps
flag:
docker-compose up --no-deps -d <container_name>
The -d
flag here is used to run the command in detached mode (in the background).
Consider the previous example with the web
, db
, and cache
containers. If you only want to restart the web
container without affecting the database or cache, you can run:
docker-compose up --no-deps -d web
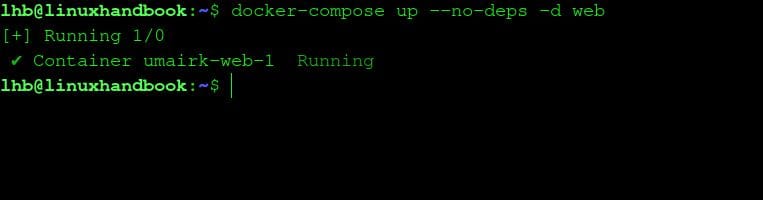
This will recreate the web
container without restarting the db
or cache
containers, even though the web
container might depend on them.
Conclusion
If you make any modifications in the docker-compose.yml
file, like change port mappings or the Dockerfile used to build an image, then restart isn't enough as docker-compose restart
only restarts the existing container. It doesn't rebuild the image or re-read the configuration from the docker-compose.yml
file.
In this case, you will have to use docker-compose up
which will rebuild the image if the Dockerfile has changed and recreate the container with the updated configuration.
I hope you find this tutorial helpful in your Docker endeavours.
A developer who is also passionate about everything homelabs, sysadmin, and DevOps.