How to Make Docker-Compose to Always Use the Latest Image
Learn various methods for getting the latest images in Docker Compose.

By default, docker compose does not always pull the latest version of an image from a registry.
This can lead to using outdated images and potentially missing bug fixes or new features.
This tutorial will discuss various methods to make Docker Compose always use the latest image.
Method 1. The --pull
Flag
The simplest way to ensure Docker Compose pulls the latest image is to use the --pull
flag when running docker-compose up
:
docker-compose up --pull always
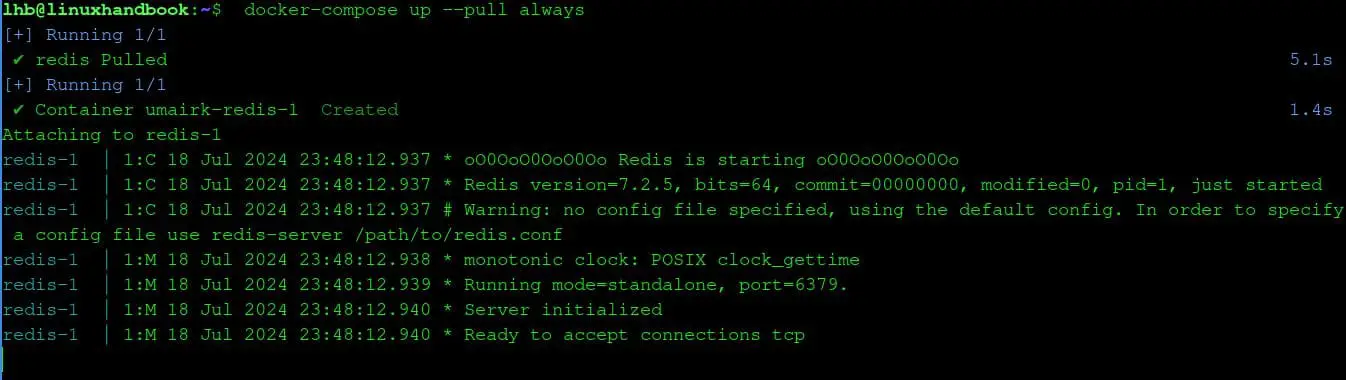
This flag forces Docker Compose to attempt to pull the latest versions of all images specified in your docker-compose.yml
file before starting or restarting services.
Method 2. The image: tag
Strategy
In your docker-compose.yml file, you can specify the image you want to use along with the latest
tag:
services:
redis:
image: redis:latest
ports:
- "6379:6379"
- "16379:16379"
volumes:
- redis-data:/data
- ./redis.conf:/usr/local/etc/redis/redis.conf
volumes:
redis-data:
This seems very easy but it's important to note that using latest
doesn't always guarantee that you will get the absolute latest image.
For example, if you had previously pulled an image tagged as latest
, Docker might use the cached version unless you explicitly tell it to pull again (using the --pull
flag).
The best way to handle this is by first stopping and removing existing containers and images, then pull the latest images from the registry, and finally starts the containers in detached mode (-d), rebuilding them if there are changes in the Dockerfile (--build):
docker-compose down --rmi all
docker-compose pull
docker-compose up -d --build
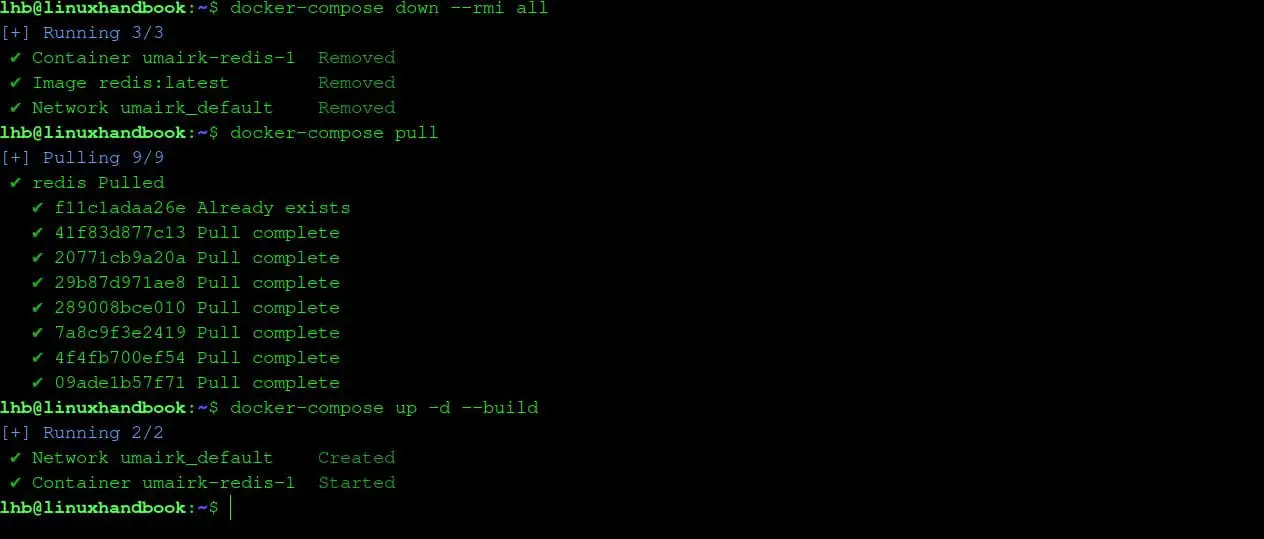
Method 3. Build Images Locally
Another comman way to make docker-compose use the latest image is by building images locally using a Dockerfile. This way you are using the latest code by rebuilding the image before running docker-compose up
:
docker-compose build --no-cache
docker-compose up
The --no-cache
flag tells Docker to rebuild the image from scratch, incorporating any changes you have made.
Method 4. Use Watchtower
Watchtower is a utility that runs as a Docker container itself. Its primary function is to monitor other Docker containers on the same host and automatically update them to newer image versions when they become available.
Watchtower is easy to set up. You can either run it as a standalone container:
docker run -d \
--name watchtower \
-v /var/run/docker.sock:/var/run/docker.sock \
containrrr/watchtower
Or integrate it into your docker-compose.yml file:
services:
redis:
image: redis:latest
ports:
- "6379:6379"
- "16379:16379"
volumes:
- redis-data:/data
- ./redis.conf:/usr/local/etc/redis/redis.conf
watchtower:
image: containrrr/watchtower:latest
volumes:
- /var/run/docker.sock:/var/run/docker.sock
command: --schedule "0 4 * * *" --cleanup --stop-timeout 300s
volumes:
redis-data:
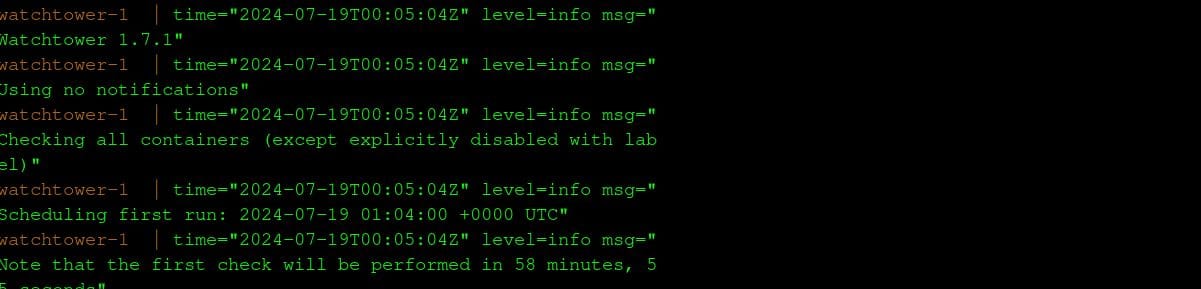
Using the latest image is not always the best practice
While using the latest image might seem ideal, it's important to understand that it is not always the best approach as latest
tag can be unpredictable.
The image it refers to might change unexpectedly, introducing unforeseen issues into your environment or new image versions might contain breaking changes.
That's why it's important to follow the best practices:
- Specific Tags: Instead of relying on
latest
tag, read the changenotes and use specific image tags. For example, redis:7.2.5. This gives you more control and predictability. - Regular Updates: Establish a schedule for updating your images to benefit from bug fixes and new features while minimizing the risk of unexpected issues.
- Testing Environments: Always test new image versions in a staging environment before deploying them to production especially when you are using a tool like Watchtower or any other tool which automaically updates the images.
Wrapping Up
It's quiet easy to make Docker Compose use the the latest image easier, however, it's important to follow the best practices to avoid breaking things and maintain a balance between staying up-to-date and maintaining the stability.
A developer who is also passionate about everything homelabs, sysadmin, and DevOps.