There are several reasons why one would want to check whether the variable is empty, such as checking for input validation.
And in this tutorial, I will show you 4 ways to check how to check if the variable is empty in bash:
- By checking the length of the variable
- Using non-empty check
- By comparing a variable to an empty string
- Using double square brackets
[[]]
So let's start with the first one.
1. By checking the length of the variable
In this method, I will be using the -z
flag with the []
operator to check for the empty variable.
First, use the following command to create and open an empty file:
nano length.sh
Now, paste the following lines of code into the file:
#!/bin/bash
# Set the variable
variable=""
# Check if the variable is empty
if [ -z "$variable" ]; then
echo "Variable is empty."
else
echo "Variable is not empty."
fi
Save changes and exit from the nano text editor.
Finally, use the chmod command to make the file executable as shown:
chmod +x length.sh
Now, you can execute the script, which should get you the output by saying the Variable is empty:

2. Using a non-empty check
In this method, I will be using the -n
flag to check whether the variable is empty or not.
Here's the simple script which will tell me whether the variable is empty or non-empty:
#!/bin/bash
variable=""
if [ -n "$variable" ]; then
echo "Variable is not empty."
else
echo "Variable is empty."
fi
As you can see, it uses a simple if-else statement it two echo statements.
And if you execute the above script, you should expect the following result:

3. By comparing a variable to an empty string
As the name suggests, in this method, I will be comparing the variable to an empty string using the []
operator.
Here's the simple script:
#!/bin/bash
if [ "$variable" = "" ]; then
echo "Variable is empty."
else
echo "Variable is not empty."
fi
If you notice, there's a condition inside []
which simply checks whether the $variable
is empty or not.
And on the basis of that, it gets the output.
If you use the shown script, you should expect the following output:

4. Using double square brackets
Using double square brackets, you get more flexibility like adding regular expressions, etc.
And here, I will be using the -z
flag which I used previously to check the length of the variable, and here too, it will have the same effect.
Here's the script:
#!/bin/bash
variable=""
if [[ -z $variable ]]; then
echo "Variable is empty."
else
echo "Variable is not empty."
fi
Once you execute the above script without any changes, you'd get the following results:

Pretty easy. Isn't it?
You may also like to learn about special variables in Bash.
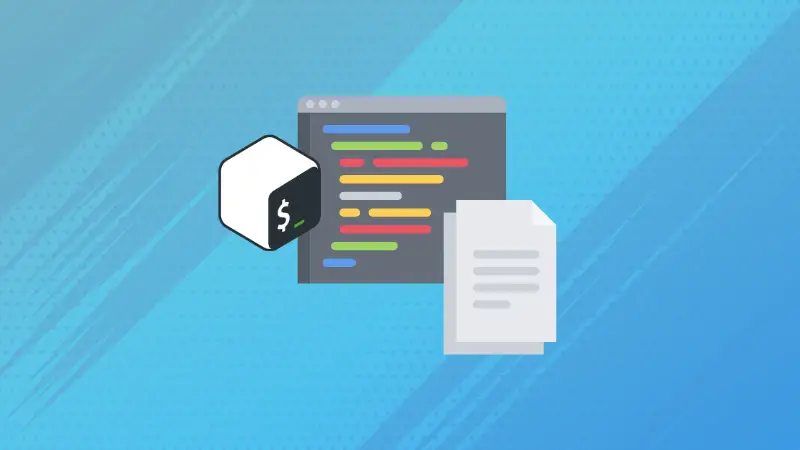
New to Bash? Start here
If you are new to Bash scripting, we have a good starting point for you.
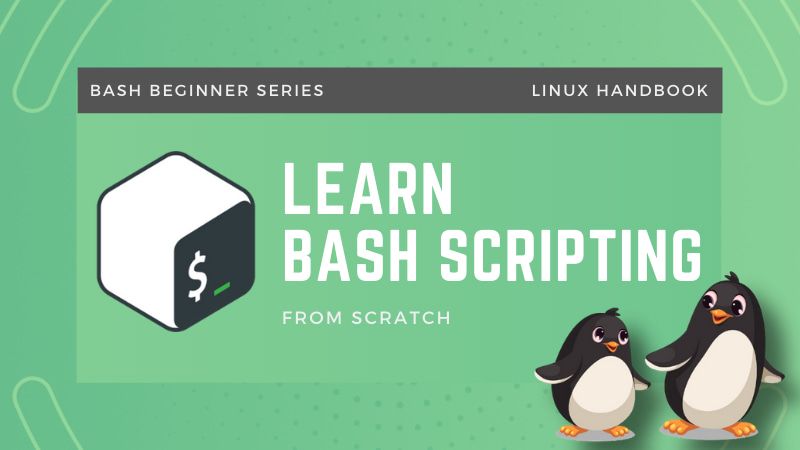
Let me know if you notice any issues with what I discussed here.
A software engineer who loves to tinker with hardware till it gets crashed. While reviving my crashed system, you can find me reading literature, manga, or watering my plants.