Using Until Loop in Bash
While for maybe the most popular bash loop, wait until you discover until. Pun intended :)

Loops are fundamentals of any programming language and so for the bash.
In bash, you are given three types of loops: for, while, and until loop. And in this tutorial, I will walk you through how to use the until loop with different examples.
So let's start with the syntax.
How to use the until loop in bash
It is necessary to learn the syntax before you apply your logic as having faulty syntax can give you multiple errors:
until [condition]
do
command
done
Here,
[condition]
is where you'd give the condition which should not be true initially so the loop will be iterated until the condition is false.do
: If the given condition is false, then, it will execute the given command given in the do... done frame.command
is what you want to iterate based on the given condition.done
is the closing part of the loop.
Sounds complex? Let me show you a simple example.
#!/bin/bash
counter=1
until [ $counter -gt 10 ]; do
echo $counter
((counter++))
done
The above script is nothing but a loop that will be iterated until the value of the counter
variable is greater than 10.
Here, the counter variable is initialized (had initial value) at one and the condition was the variable should be greater than 10!
This means the loop will be iterated 10 times.
And to change the value of the counter
variable after each variable, I used counter++
which will increment +1 after each loop iteration.
When I executed the above script, it gave me the following output:
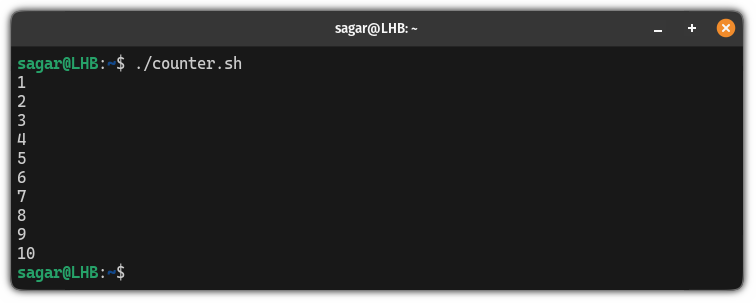
Want more examples? here you have it!
1. A simple user input loop
If you want the user to input a specific choice and then it prints the input, then, you can use the until loop for that case.
Here's what you can use:
#!/bin/bash
input=""
until [ "$input" = "yes" ] || [ "$input" = "no" ]; do
read -p "Please enter 'yes' or 'no': " input
done
echo "Valid input received: $input"
If you notice, there are two conditions that are used with the OR operator ||
and the loop will be iterated until you enter any of the two choices: yes or no.
The read
the command is used to take input from the user.
And once you enter any of the two choices, the loop will exit and the choice will be printed using the echo command.
When I executed the above script, it gave me the following results:
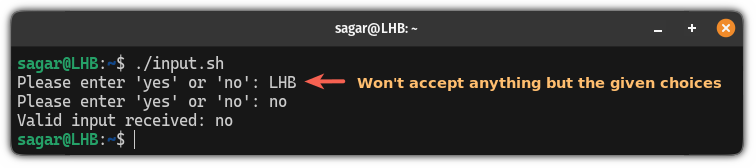
2. Simple guess the number game
Yep, you can use the loop to create simple games!
Let's have a look at the loop first:
#!/bin/bash
secret_number=42
guess=0
until [ $guess -eq $secret_number ]; do
read -p "Guess the secret number: " guess
if [ $guess -lt $secret_number ]; then
echo "Too low!"
elif [ $guess -gt $secret_number ]; then
echo "Too high!"
fi
done
echo "Congratulations! You guessed the secret number correctly."
Here, I have used two variables: secret_number which will store the actual number that needs to be guessed and the guess
variable that will store the user input.
Then, there are if and elseif statements that will be used if you guessed the number more or less!
And if you succeed in guessing the number, then, it will print the congratulations message.
Here's what you should be expecting if you executed the above script:
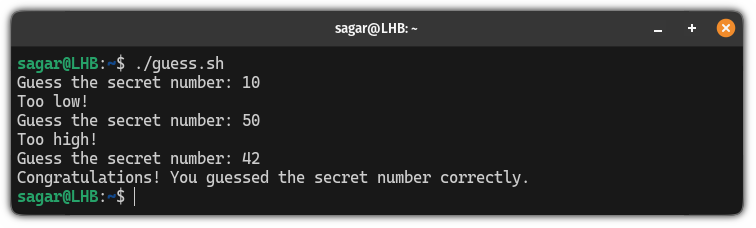
3. Create a simple timer
Yes, you can create a simple timer that will count in reverse!
Sounds fun? Here's the code:
#!/bin/bash
seconds=10
until [ $seconds -eq 0 ]; do
echo "Countdown: $seconds"
sleep 1
((seconds--))
done
echo "Time's up!"
Here, I have used a seconds
variable that is initialized at 10.
Then, it will print the current value of the variable, sleep for one second, and then reduce one number from the seconds
variable.
Once the value of the seconds
variable is reduced to 0
, it will print the Time's up!
message.
Curious to know how it will work in actual life? Here' the live execution of the above script:
Learn bash from scratch
If you're looking for a way to kick start the bash journey, we made a dedicated tutorial series that should cover all the basics:
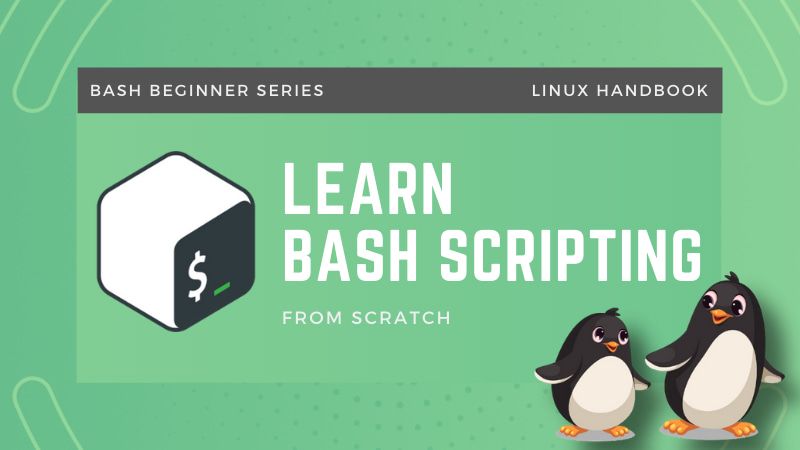
I hope you will find this guide helpful.
A software engineer who loves to tinker with hardware till it gets crashed. While reviving my crashed system, you can find me reading literature, manga, or watering my plants.