Check the Number of Arguments in Bash
Working on a bash script that accepts arguments and you need to count them? Here's how to check the number of arguments supplied to your script.
β Sagar Sharma

There are times when you want to get the exact numbers of the arguments passed to the script while executing.
And for that purpose, the bash has a special variable ($#
) which prints the count of the total number of arguments passes to the shell script appended while execution.
So in this guide, I will walk you through various examples of how you can use the $#
variable to get the total number of arguments passed in bash.
How to check the number of arguments in bash
To check the number of arguments, all you have to do is append is following line to your bash script:
echo $#
But to make it more readable, you can print additional lines to your reference such as:
echo "The total number of arguments are:"
echo $#
For your reference, here, I created a sample hello world script named Hello.sh
which will be counting the number of arguments:
#!/bin/bash
echo " Hello World!"
#The argument counter
echo "The total number of arguments are:"
echo $#
Once done, make the file executable using the chmod command:
chmod +x Hello.sh
And now, you can execute the script withthe Β desired number of arguments:
./Hello.sh -arg1 -arg2 -arg3
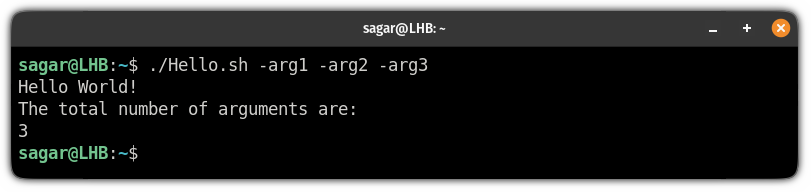
You may of course combine the two:
echo "The total number of arguments are: $#"
But what if you want to print the passed arguments too? Well, you can refer to the three ways I'm about to show you.
Check number of arguments and which arguments were passed in bash
So if you want to find the number of arguments including which arguments were passed to the script, here you have it.
And I will walk you through three ways to do see the arguments passed to the bash script:
- Using a variable to store the passed argument (has a major downside)
- Using the
@
variable - Using the
*
variable
1. Using a variable to store the passed argument
In this method, you will have to assign variables that will store the given arguments to the bash script.
So if you want to use this method, all you have to do is echo various variables in the following manner:
echo $1 $2 $3
Here, I've used 3 variables so if the arguments are more than 3, anything more than 3 will be stripped down.
So the final script would look like this:
#!/bin/bash
echo "Hello World!"
echo $1 $2 $3
# The argument counter
echo "The total number of arguments are:"
echo $#
And now, if you try to execute the script with the desired number of arguments, it will print the passed arguments too:
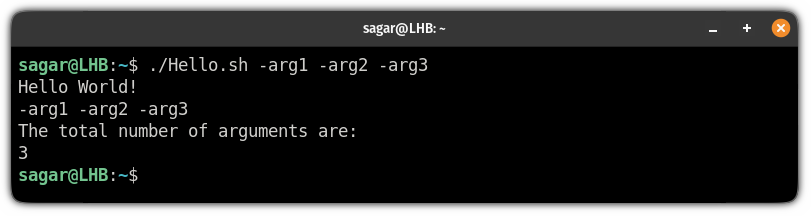
But if I pass more than 3 arguments, it will still show the 3 arguments only as there are only 3 variables to store (sure, you can use more variables):
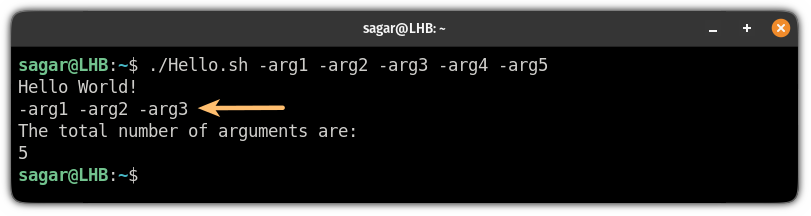
So if you want to get away with allocating numerous variables to be on the safe side, you can refer to the next 2 ways which won't require you to use variables.
2. Using the @ variable
Using this method, you no longer have to manually add variables to store the arguments. The special bash variable $@ stores all the arguments.
To use this method, all you have to do is echo the @
variable as shown:
echo $@
So the final script will look like this:
#!/bin/bash
echo "Hello World!"
echo $@
# The argument counter
echo "The total number of arguments are:"
echo $#
Once done, you can pass the desired amount of arguments and it will print all of them including numbers:
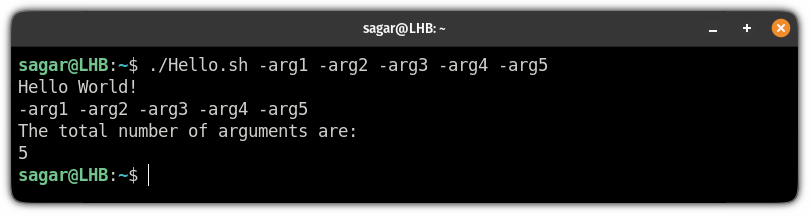
3. Using the *
variable
This is similar to the above method but uses the *
variable. The only difference is that it stores the entire parameter as a single string. It still gets you the same result in most cases though.
To use this method, you will have to echo the *
variable as shown:
echo $*
Once you do that, the script should look like this:
#!/bin/bash
echo "Hello World!"
echo $*
# The argument counter
echo "The total number of arguments are:"
echo $#
And now, if you execute the script with the desired amount of arguments, it will give you a number of arguments and which arguments were passed:
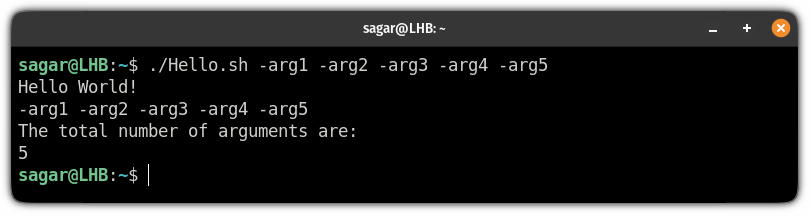
That's it!
Just getting started with bash?
There are many other variables with special meanings. You should check them out as well.
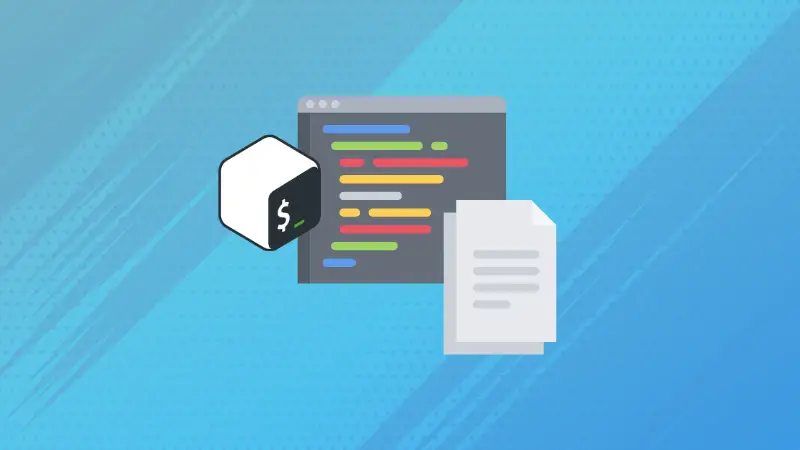
If you are just getting started with bash scripting, we have a dedicated series that covers all the basics that you'd require to become a better Linux user:
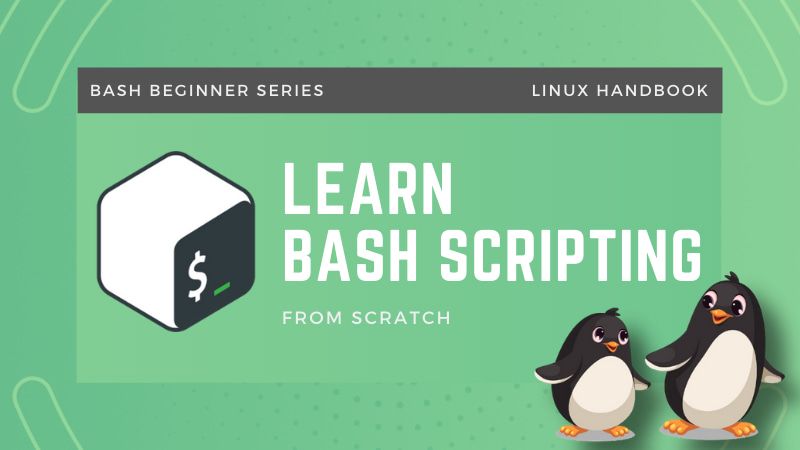
I hope you will find this guide helpful.
And if you have any doubts, let me know in the comments.
A software engineer who loves to tinker with hardware till it gets crashed. While reviving my crashed system, you can find me reading literature, manga, or watering my plants.