Using if else Statements in Awk
Learn to use conditional statements in AWK. With the use of if else, you can write advanced AWK scripts.
— Team LHB

AWK is a powerful scripting language that comes baked into the bash shell.
It is extremely versatile and can be used to write all kinds of data extraction scripts.
Conditional statements are an integral part of any programming or scripting language and AWK is no different.
In this tutorial, I'll show examples of using if-else statements in AWK. Here's the sample data on which I base my examples.
ID | Name | Age | Subject |
---|---|---|---|
111 | Farhaan | 18 | DevOps |
89 | Alex | 21 | SecOps |
92 | Ronn | 22 | IT |
100 | Robert | 23 | Commerce |
102 | Samantha | 20 | Cloud-Admin |
105 | Bob | 21 | Maths |
I store it in students.txt
. You can download it if you want to try the examples on your own.
1. The if statement in Awk
An if statement checks whether the condition is true or false. If the condition is true, then it executes the statements.
Here's a simple syntax for the if statement in awk:
awk '{if (condition) {statement} }' [input_file]
Now, let's use our sample data of the students.txt file and print the details of the student with ID 100 using if condition in AWK.
awk '{
if ($1=="100")
{
print "............... \n";
print "Name : " ,$2;
print "Age : ",$3;
print "Department : " ,$4;
}
}' students.txt
Here's the execution of the above awk command:
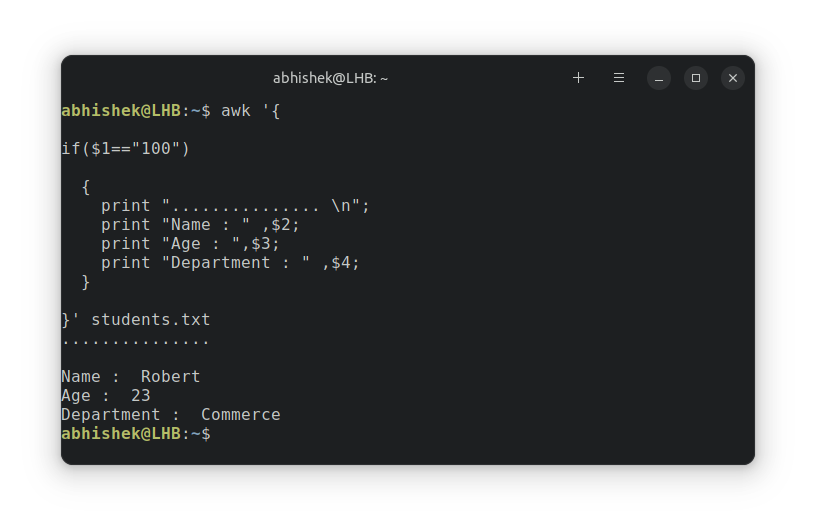
The entire above command can also be written in a single sentence like this:
awk '{ if ($1=="100") { print "............... \n"; print "Name : " ,$2; print "Age : ",$3; print "Department : " ,$4; } }' students.txt
But the first one is easier to read and understand.
2. The if else statement in Awk
In the previous example, there was only one condition and one action. The if else
statement is slightly different from the if
statement.
The general format for the if else
statement in awk is:
awk '{
if (condition)
{command1}
else
{command2}
}' [input_file]
Here, if the condition is true, then command1 will be executed, and if the condition is false, then command2 from the else part will be executed.
Let's again take the student.txt
data file.
Suppose you want to get all the student's name and their department whose age is less than or equal to 20 years.
The AWK command could be like this:
awk '{
if ($3<20)
{
print "Student "$2,"of department", $4, "is less than 20 years old"
}
else
{
print "Student "$2,"of department", $4, "is more than 20 years old"
}
Here's the execution and output of the if else example:
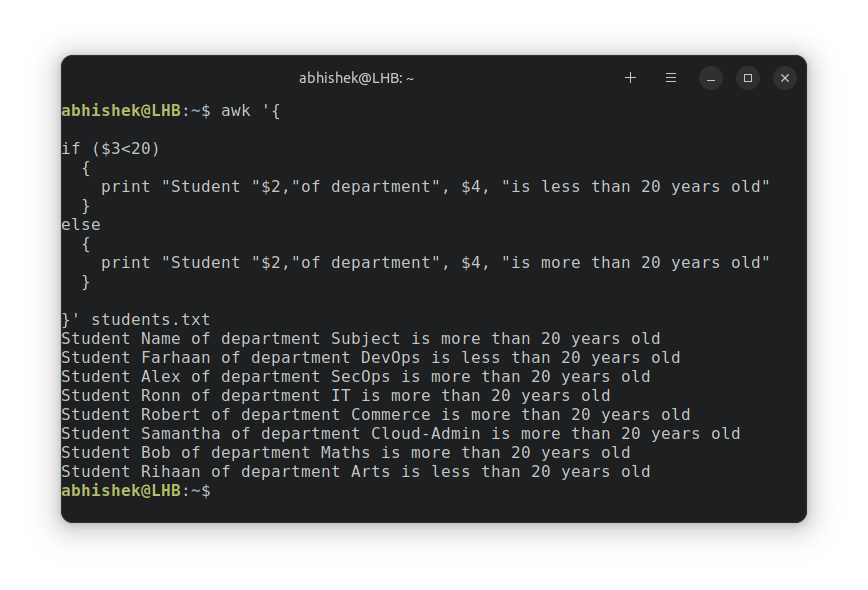
As you can see, there are only two students aged less than 20 years. The rest of the students are more than 20 years old.
But there is a problem in the above example. It also considers the description of the table as real data and tells us that Student Name of age Age is more than 20 years old.
Let's rectify this with an if else-if statement.
3. The if else-if statement in Awk
With the if else if statements, the awk command checks for multiple conditions.
If the first condition is false then it checks for the second condition. If the second condition is also false then it checks for the third condition and so on. It checks for all the conditions until one of the conditions is true. If no condition is satisfied, the else part is executed.
if(condition1){
command1
}
else if(condition2) {
command2
}
else if(condition3) {
command3
}
.
.
.
else{
commandN
}
In the previous example, the output also considered the first line of the data which is just the description.
Let's put a condition to check if the age is a number or not. I am using a regex /[0-9]+$/
which means numeric values. The ~ means the argument has a number. And the negation operator before them means that the field should not have a number.
awk '{
if (! ($3 ~ /[0-9]+$/))
{
print "Age is just a number but you do not have a number"
}
else if ($3<20)
{
print "Student "$2,"of department", $4, "is less than 20 years old"
}
else
{
print "Student "$2,"of department", $4, "is more than 20 years old"
}
}' students.txt
Let's run it and focus on the first line of the output.
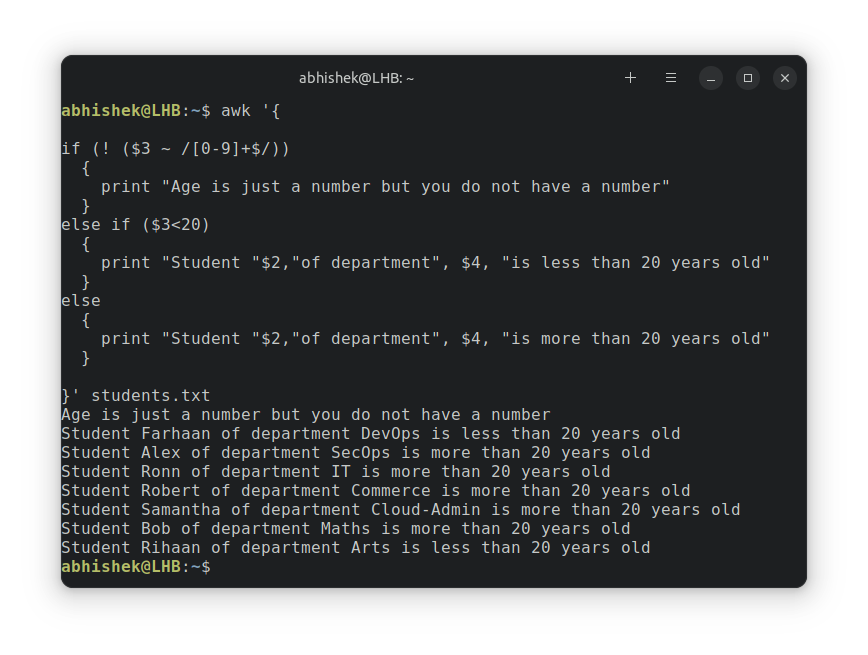
Bonus Tip: Use awk program file
If you find writing long awk programs in the terminal directly troublesome, use awk files.
Create a new file and name it example.awk or anything you want. The extension doesn't matter here. It just makes it easy to understand that the file is an awk program file.
You put the content between the ''
that comes just after awk in the above examples.
{
if (! ($3 ~ /[0-9]+$/))
{
print "Age is just a number but you do not have a number"
}
else if ($3<20)
{
print "Student "$2,"of department", $4, "is less than 20 years old"
}
else
{
print "Student "$2,"of department", $4, "is more than 20 years old"
}
}
Now, use this awk file in the following manner:
awk -f example.awk students.txt
It will produce the same output that you saw previously.
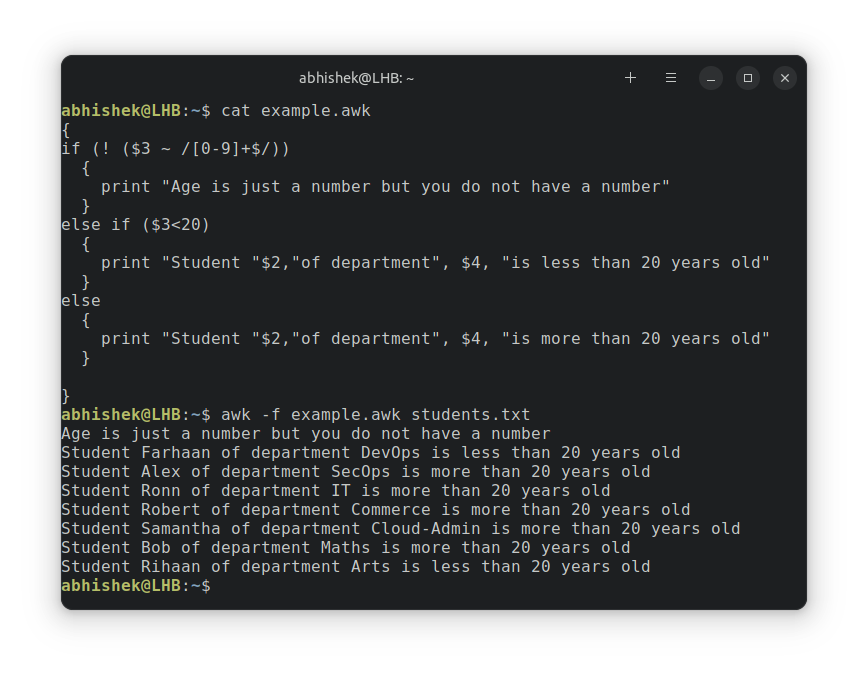
More Bonus Tip: Use the ternary operator instead
Instead of using if else statements in awk, you can also use the ternary operator. Ternary expressions are the shorthand version of the if-else statement of the Awk.
If the condition is true then command1 will execute; otherwise, if the condition is false then command2 is executed.
(condition) ? Command1:Command2
Consider the following example of the awk command:
awk '{print ($3 <=20)? "Age less than 20: " $2: "Age over 20: " $2}' students.txt
In this example $3
refers to the Age
field. If the age is less than or equal to 20, then it will print the first command that displays "Age is less than 20". If the first part is False, it will execute the second command, "Age is over 20 ".
abhishek@LHB:~$ awk '{print ($3 <=20)? "Age less than 20: " $2: "Age over 20: " $2}' students.txt
Age over 20: Name
Age less than 20: Farhaan
Age over 20: Alex
Age over 20: Ronn
Age over 20: Robert
Age less than 20: Samantha
Age over 20: Bob
Conclusion
In this guide, you learned different ways of using if-else
statements in the AWK programming language. Using these statements, you can control the flow of a program, evaluate a condition, extract data from a file, etc.
If you need a few more examples, this article will help you.
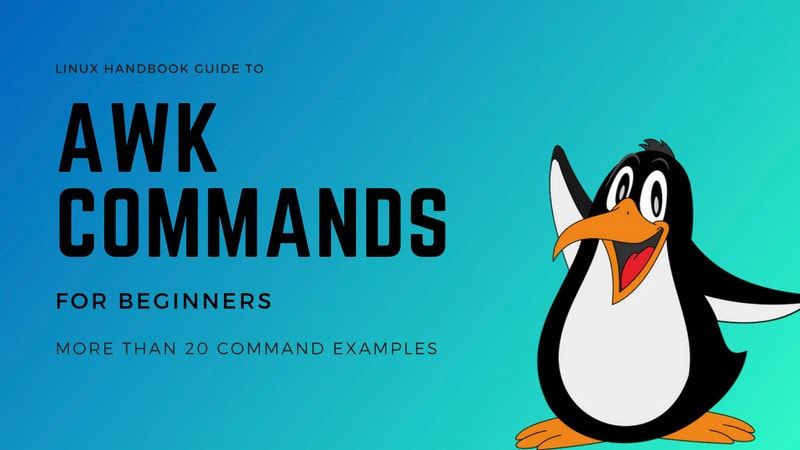
Team LHB indicates the effort of a single or multiple members of the core Linux Handbook team.