Unusual Ways to Use Variables Inside Bash Scripts
You might have used variables in Bash before, but probably not like this.

You probably are aware of variables in Bash shell scripting.
Like other programming and scripting languages, you use variables to store the data and then reference them later in future commands.
name="Linux Handbook"
echo "Hello $name!"
And it will print "Hello Linux Handbook!".
Outside of the example above, there's also a surprisingly large amount of things you can do with variables, such as filling in a default value when the variable isn't set, and assigning multiple values to a single variable.
Let me show you these unusual and advanced usage of variables in bash scripting.
Using arrays
Excluding the above example, the most common type of variable you'll find in Bash is arrays:
name=("Linux Handbook" "It's FOSS")
The above example assigns both Linux Handbook
and It's FOSS
as the values for the name
variable.
How do you go about accessing each value though?
If you run echo $name
, you'll see that it prints the first value, Linux Handbook
. To access the other values, you'll need to use a different syntax for variables.
To begin pulling the other values, let's start with getting familiar with the following:
echo "Hello ${name}!"
This is the basis for what will allow you to obtain all of the variable's values, and is also what's going to allow you to perform the rest of the methods for interacting with variables throughout the rest of this article.
Going back to the aforementioned example, you can use the ${variable[number]}
syntax to pull a certain item from a variable:
echo "Hello ${name[1]}!"
This one will print "Hello It's FOSS!"
If you couldn't already see from the above example, the first item in the array actually starts at 0 instead of 1.
So to pull the Linux Handbook
value, you would pull the item at position 0:
echo "Hello ${name[0]}!"
Likewise, simply omitting the [number]
part simply makes it default to the first item in the array (that being at index 0).
And those make up all the variable types you'll actually find inside of Bash.
Let's now get in to how you can actually work with and modify the output of those variables when we call them.
Setting the value when a variable isn't set
You can make a variable default back to a certain string when a variable isn't set like so:
${variable:-string}
Take this example:
echo "Hello ${name:-nobody}!"
Since the variable name
is not set, it will be defaulted to nobody
and the command above will print "Hello nobody!".
If the variable is set beforehand, its value will be used:
name="Linux Handbook"
echo "Hello ${name:-nobody}!"
The above command prints "Hello Linux Handbook!"
Setting the value when a variable is already set
You can also set a variable to return a certain value when the variable is set. This would cause the variable to print a certain string whenever it is set, and print nothing when it is not.
The syntax for this method is similar to the previous one, but uses the +
sign instead of -
.
${variable:+string}
Take this example where variable name is not set. So it won't print anything for that variable part and the output for the command will be "Hello !"
echo "Hello ${name:+person}!"
Modify the above example and set the variable name
. With this special method, it will not use the already set value and use the one you provided.
name="Linux Handbook"
echo "Hello ${name:+person}!"
The above command will print "Hello person!".
You'll probably find more use cases with the ${variable:-string}
syntax, as some of the benefits of ${variable:+string}
can already be accomplished with other tools like the testing ([]
) operator:
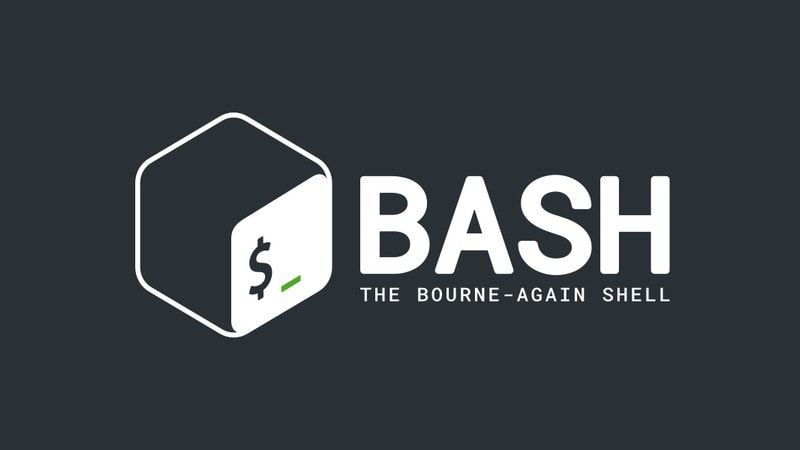
Indirect references to variables
The next example will use the following syntax:
${!variable}
This one is a bit more complex in how it functions, but makes sense after you see how it works:
name="Linux Handbook"
variable="name"
echo "Hello ${!variable}!"
It will print "Hello Linux Handbook".
That !
character before the variable name causes the normal variable name to be substituted, but then uses the name of that string to find the relevant variable.
Likewise:
variable
is substituted for the stringname
, followed by:name
being substituted for its value ofLinux Handbook
.
Finding the length of a variable
Let's use the previous method, but exchange the !
character for #
:
${#variable}
Using this method will print the length of the variable, but will act slightly different depending on if the variable is a string or an array.
Using that syntax on a string will cause the variable to be substituted for the amount of characters in the string.
variable="name"
echo "Length is ${#variable}."
It will prnt 4. But on the otherhand, using it on an array will print the number of items inside the array, i.e. array size, which is 2 here.
variable=("name" "word")
echo "Length is ${#variable}."
Lowercasing and capitalizing strings
Lastly, you can capitalize and lowercase a string using the ^
and ,
operators respectively.
You provide these at the end of the variable name like this:
# Capitalizes
${variable^}
${variable^^}
# Lowercases
${variable,}
${variable,,}
What's the difference between providing one and two of the characters?
Providing just one (i.e. ${variable^}
) will cause only the first letter to be modified, while providing two (${variable^^}
) will cause the entire string to be modified.
The example below prints Name
instead of name
:
variable="name"
echo "${variable^}"
But this one prints NAME
:
variable="name"
echo "${variable^^}"
Similarly, you can print variables in lowercases as well.
The example below will print wIDEname
:
variable="WIDEname"
echo "${variable,}"
And this one will print widename
:
variable="WIDEname"
echo "${variable,,}"
Wrapping up
These are only some of the ways you can use variables inside of Bash. You can find various more ways to interact with variables inside of Bash's documentation on variable expansion.
Got any questions with how the above examples worked, or something just not working right? Feel free to leave any of it in the comment section below.
Sole Linux user with Ubuntu running my desktops and servers. You can check out some stuff I've made on GitHub.