Useful Bash Commands You May Not Know About
Here are some lesser known but useful bash commands that will help make the shell scripts you write cleaner and more maintainable.

Bash is a fairly powerful language to program in, and is also quite easy to start off with.
After all, it's almost universally the shell you're going to see when you open up your terminal. That makes it extremely useful to get accustomed to.
There's some powerful commands in Bash that you may not be aware of though, even if you're fairly seasoned with using the language. All of these commands can serve quite useful purposes though, and can make the shell scripts you write cleaner, more maintainable, and just outright more powerful than they could've been before.
Read: Asking input from the User
The read command allows you to take input from a user and store it inside a variable.
#!/usr/bin/env bash
echo "What is your name?"
read name
echo "Your name is ${name}!"
This will wait for input from you, the user, and then sets the value for the name
variable to the string that you enter.
For example, if you specified your name as Linux Handbook
when running the above script, it would output Your name is Linux Handbook!
.
You can also make the above example more compact by specifying the -p
option to the read
command, which will print the string specified after -p
, and then ask the user for input:
#!/usr/bin/env bash
read -p "What is your name? " name
echo "Your name is ${name}!"
You'll also notice that I added a space after What is your name?
. If we didn't add it, it would literally print What is your name?
with no space, which would make it look a bit more weird when the user is entering in their input:
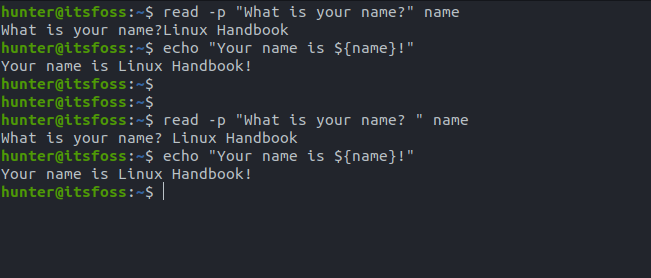
Mapfile: Assigning a variable the values of a file's lines
You can use the mapfile
command to read the content of a file, and then assign that output to a Bash array, with each array item being created whenever a new line is encountered in the file.
For example, let's create a file called file.txt
that contains the following text:
Line 1
Line 2
Line 3
Line 4
Line 5
You could convert this file into a Bash array by running the following, which assigns the file's content to the file_var
variable:
#!/usr/bin/env bash
mapfile file_var < file.txt
for i in "${file_var[@]}"; do
echo "${i}"
done
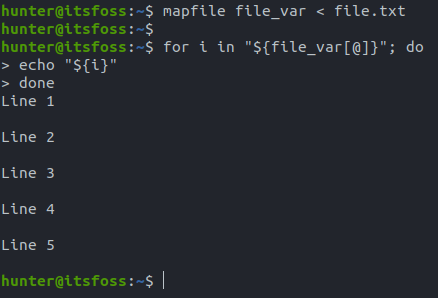
You'll notice that the above produces some weirdly-spaced output as well that doesn't align with what's inside the file, which can be resolved by adding the -t
option to the mapfile
command:
#!/usr/bin/env bash
mapfile -t file_var < file.txt
for i in "${file_var[@]}"; do
echo "${i}"
done
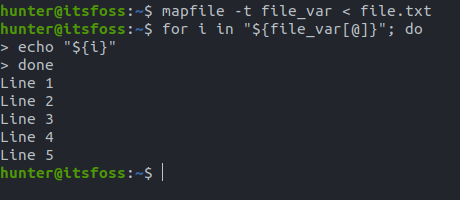
Lastly, you may notice that the following does not work:
#!/usr/bin/env bash
cat file.txt | mapfile -t file_var
This is because the mapfile
command on the right side of the pipe is run in a subshell (or in other words, a new instance of Bash), which is unable to affect your current shell.
Source: Put variables from a file into your shell's environment
Say you have the following imaginary file, config.sh
, with the following content:
username=linuxhandbook
domain=linuxhandbook.com
What if you'd like to take the content of that file and put it into your current shell's environment?
This can be done quite simply by just using the source command:
source config.sh
You can then check to see that the variables were assigned as expected:
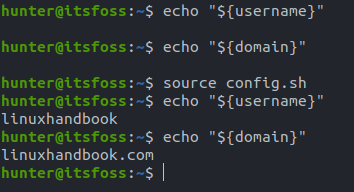
Under the hood, the source
command actually runs the specified file in your current shell's environment, so typing any commands in that file would actually cause them to be executed when running source
.
For example, let's put the following into config.sh
:
name="Linux Handbook"
echo "Hello ${name}!"
echo "Have a good day!"
If we then run soure config.sh
, you'll see that the two echo
commands get run, as well as the name
variable getting assigned:
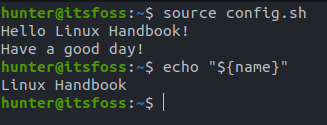
You can also use .
in place of source
, which acts just the same way that source
would.
Wrapping Up
And just like that you're further on your way to doing more powerful and useful tasks, all the while being able to stay inside of Bash.
Are there any other commands that you frequently use in Bash? Feel free to leave any of them in the comments below.
Sole Linux user with Ubuntu running my desktops and servers. You can check out some stuff I've made on GitHub.