5 Examples of Python While Loop
Wondering how to use while loop in Python? These 5 code snippets show how while loop is used in Python scripts.

Even though the for loop achieves the same thing with fewer lines of code, you might want to know how a “while” loop works.
Of course, if you know any other programming languages, it will be very easy to understand the concept of loops in Python.
In this article, I shall highlight a few important examples to help you know what a while loop is and how it works.
Note: I’d also recommend you to check out some of the best free Python resources if you’re just starting out.
While Loop in Python
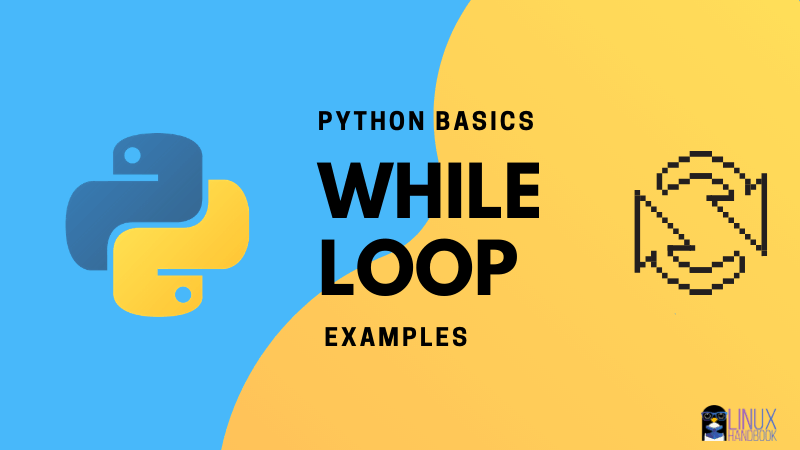
A while loop executes the statement(s) under it till the condition is true. It is worth noting that repeatedly executing the statements is also known as iteration.
Unless the condition is false, it keeps executing the same statement(s). And, when the condition is false – it skips the statements under while loop and executes the next statement(s) in the program.
So, for instance, if you have a condition that is always true – it ends up being an infinite loop. You will have to close the program in order to stop the execution.
Fret not, in this article, I shall include an example for an infinite while loop and some common examples that use if-else or break statement coupled with the while loop.
Python While Loop Examples
Let us take a look at a few examples of while loop in Python so that you can explore its use easily in your program.
1. Printing a range of numbers in Python
number = 0
while number <=5:
print(number)
number +=1
print("Printed a range of numbers")
So, here, you have to adjust/tweak the range using a less-than-equal to operator in the condition and keep incrementing the numbers to display the numbers.
Of course, it takes more lines of code when compared to for loop for the same thing.
2. Using if statement within While loop
number = 0
while number <=5:
print(number)
if number == 2:
print(number)
number +=1
print("Printed!")
Just like you would normally use the if statement, you can utilize it within the while loop as required.
3. Using else with While loop in Python
You can pair another set of statements with the while loop using the else statement if the condition is false. Here’s how it would look like:
number = 0
while number <=5:
print(number)
number +=1
else:
print("Done printing numbers till 5")
4. Using break statement within While loop
When you use a break statement within a while loop – it halts the loop and executes the statement outside the while loop. Here’s an example:
number = 0
while number <=5:
print(number)
if number == 2:
break
number +=1
print("Printed!")
5. Using continue statement within While loop
Whenever you write a continue statement, it directly executes the loop again by ignoring the statements after the continue statement.
number = 0
while number <=5:
number +=1
if number == 2:
continue
print(number)
print("Printed!")
6. Executing an infinite loop
If the condition never ends up to be false, it becomes an infinite loop. Here’s an example:
while 1==1:
print("Looping......")
Wrapping Up
Now that you’ve seen how a while loop works – feel free to explore using it for a variety of programs as required.
If you’re aware of clever trick using the while loop or have trouble using it in your program, let me know in the comments below.
A passionate technophile who also happens to be a Computer Science graduate. He has had bylines at a variety of publications that include Ubergizmo & Tech Cocktail. You will usually see cats danc