Appending to Arrays in Bash
In this quick Bash tip, you'll learn about appending to an existing array in bash.

Unlike many programming languages, bash doesn't have any pre-built function that lets you append data to arrays in bash.
But this doesn't mean it can't be done.
And in this tutorial, I will show you two ways to append to an array in bash:
- Using the shorthand operator (allows you to append multiple elements)
- By defining the last index
Append to array using += in bash
The shorthand operator (+=
) is the easiest way to append to an array in bash, and it can be used in the following manner:
Array+=("Data to append")
For example, I want to work with an array named arrVar
which contains the following:
arrVar=("Ubuntu" "Debian" "openSUSE" "Fedora" "Void" "Gentoo")
And I want to append one more value Arch
to the existing array, so my script would look like this:
#!/bin/bash
#Main Array to work with
arrVar=("Ubuntu" "Debian" "openSUSE" "Fedora" "Void" "Gentoo")
#Value that needs to be appended
arrVar+=("Arch")
To print the values, I will be using a simple for loop in bash that will print the value of arrVar
one by one in each iteration:
#!/bin/bash
#Main Array to work with
arrVar=("Ubuntu" "Debian" "openSUSE" "Fedora" "Void" "Gentoo")
#Value that needs to be appended
arrVar+=("Arch")
#Printing the values of an array
for value in "${arrVar[@]}"
do
echo $value
done
To run the script, make it executable using the chmod command.
Finally, execute the script:
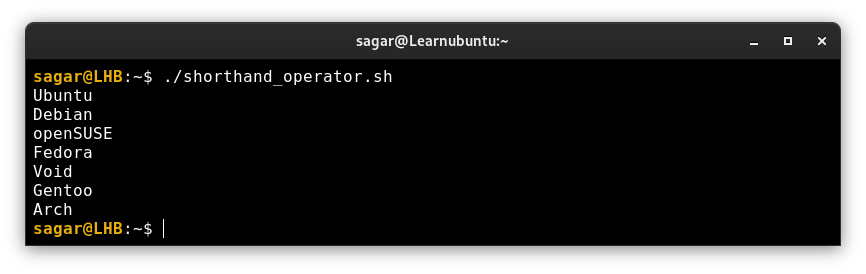
Append to multiple arrays in bash
If you want to append multiple elements, you can add more data as shown:
my_array+=("element1" "element2" "element3")
For example, here, I added 3 more elements (names of distros):
arrVar+=("Pop_OS" "Linux_Mint" "Zorin")
And when executed, it gave me the following results:
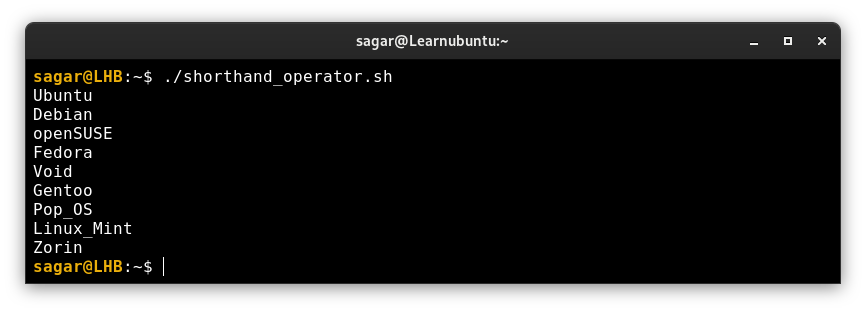
A convenient way to append elements. Isn't it?
Append to array to bash by defining the last index
This is yet another simple way to append to an array in bash, where you will be adding data to the last index.
But how are you supposed to find the last index? I know if you have a small array, it can be done manually, but what about the times when dealing with 100s or 1000s of data entries?
In that case, you can use #
and @
in the following manner:
array_name[${#array_name[@]}]="Data to append"
For example, if I want to add Slackware
to the array named arrVar
, then, my bash argument would look like this:
arrVar[${#arrVar[@]}]="Slackware"
And this is how my script would look like:
#!/bin/bash
#Main Array to work with
arrVar=("Ubuntu" "Debian" "openSUSE" "Fedora" "Void" "Gentoo")
#Value that needs to be appended
arrVar[${#arrVar[@]}]="Slackware"
#Printing the values of an array
for value in "${arrVar[@]}"
do
echo $value
done
Now, if I execute the above script, the Slackware
should be the last entry in the output:
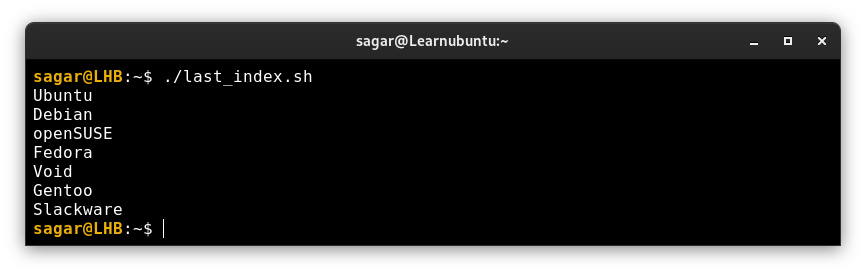
Pretty cool. Isn't it?
Learn bash scripting for free
If you are just getting started with the bash, we have a dedicated series of tutorials on bash that would help you a lot to get the basics right:
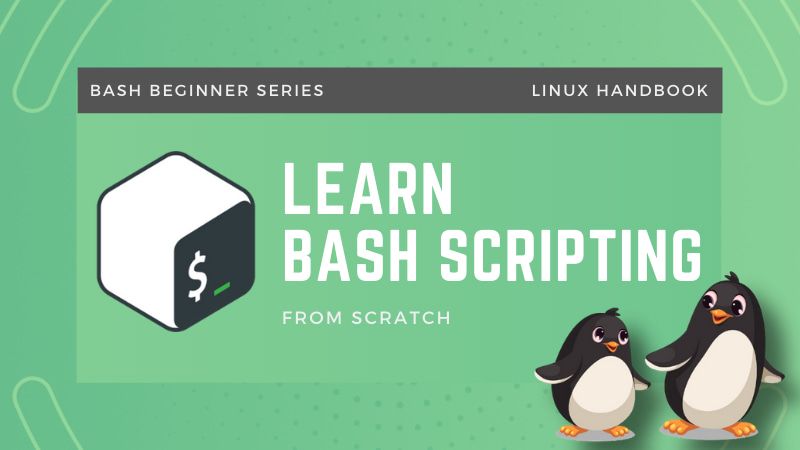
I hope you'd no longer find appending to array in bash a challenging task.
A software engineer who loves to tinker with hardware till it gets crashed. While reviving my crashed system, you can find me reading literature, manga, or watering my plants.